Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial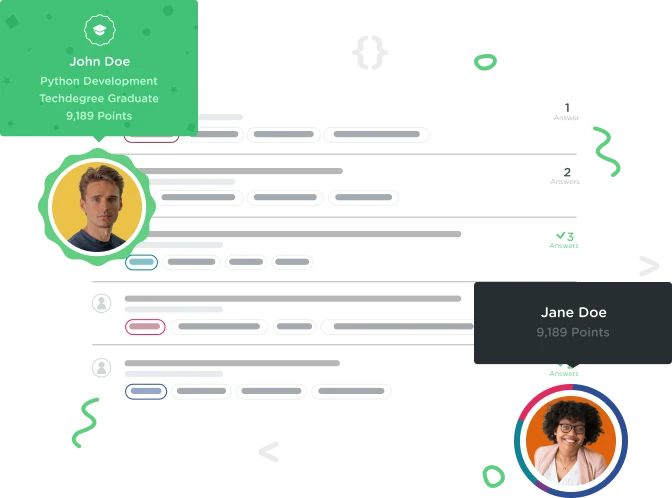

Adam Laszlo Vincze
4,083 PointsMy build an object challenge
Hello,
Here is my code. Do you think it works?
/* Part one */
var student;
var response = '';
function print(message) {
var out = document.getElementById('output');
out.innerHTML = message;
}
var students = [
{
name: 'Steve Butabi',
track: 'Web',
achievements: '668',
points: '1556'
},
{
name: 'Forrest Runner',
track: 'IOS',
achievements: '1125',
points: '2836'
},
{
name: 'Laslie Thomson',
track: 'Graphic Design',
achievements: '315',
points: '553'
},
{
name: 'Tom Kukac',
track: 'Android',
achievements: '995',
points: '2365'
},
{
name: 'Robert Nash',
track: 'C#',
achievements: '550',
points: '1316'
},
];
/* Part two */
for (var i = 0; i < students.length; i++) {
var query = students[i];
response += '<h2>Student:'+ query.name +'</h2>';
response += '<h2>Skills:'+ query.track +'</h2>';
response += '<h2>Achievements:'+ query.achievements +'</h2>';
response += '<h2>Points:'+ query.points +'</h2>';
}
print(response);
1 Answer

Iain Simmons
Treehouse Moderator 32,305 PointsYeah it works! Instead of declaring a new variable query
in the loop, you might as well use student
, since you declare that at the top but don't use it.
And you could have used a for..in
loop to access all the properties in each object instead of hard coding the keys, but that's fine.
Here's how I did it:
var html = '';
var students = [
{
name: 'Iain Simmons',
track: 'Full Stack JavaScript',
achievements: 219,
points: 18767
},
{
name: 'Dave McFarland',
track: 'Full Stack JavaScript',
achievements: 197,
points: 18375
},
{
name: 'Kenneth Love',
track: 'Learn Python',
achievements: 38,
points: 8504
},
{
name: 'Andrew Chalkley',
track: 'Full Stack JavaScript',
achievements: 175,
points: 14544
},
{
name: 'Nick Pettit',
track: 'Front End Web Development',
achievements: 79,
points: 6470
}
];
function print(nodeID, message) {
document.getElementById(nodeID).innerHTML = message;
}
function buildDefinitionList(obj) {
var prop;
var dListHTML = '<dl>';
for (prop in obj) {
dListHTML += '<dt>' + prop + '</dt>';
dListHTML += '<dd>' + obj[prop] + '</dd>';
}
dListHTML += '</dl>';
return dListHTML;
}
function buildStudentList(list) {
var i;
var uListHTML = '<ul>';
for (i = 0; i < list.length; i++) {
uListHTML += '<li>' + buildDefinitionList(list[i]) + '</li>';
}
uListHTML += '</ul>';
return uListHTML;
}
html = buildStudentList(students);
print('output', html);
Guillaume Proulx
Courses Plus Student 11,949 PointsGuillaume Proulx
Courses Plus Student 11,949 PointsThis code works for me :
I got this output in the dive with the id #output
Student:Steve Butabi Skills:Web Achievements:668 Points:1556 Student:Forrest Runner Skills:IOS Achievements:1125 Points:2836 Student:Laslie Thomson Skills:Graphic Design Achievements:315 Points:553 Student:Tom Kukac Skills:Android Achievements:995 Points:2365 Student:Robert Nash Skills:C# Achievements:550 Points:1316
If that's what you wanted, then you're good to go my friend ! You can get rid of the 'var student;' though