Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial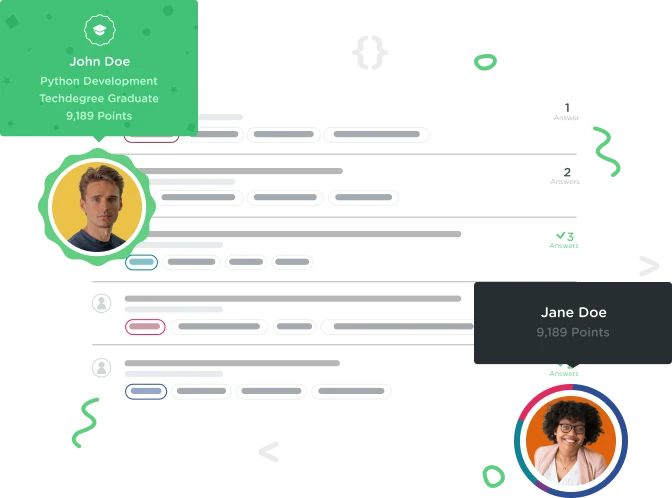
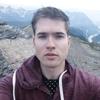
Gian Lazzarini
3,263 PointsMy C# Basics Final Quiz 1-3 code works in visual studio but won't pass the quiz. What's wrong?
I don't understand, I have all of the input validation, error handling working but it still won't pass the quiz. Is there something I've missed, gotten wrong or messed up? Or is this possibly just how the quiz is interpreting my code that won't allow it to be accepted?
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
while (true)
{
Console.Write("Enter the number of times to print \"Yay!\": ");
string userEntry = Console.ReadLine();
int x = 0;
if(userEntry.ToLower() == "quit") {
break;
}
try
{
if (int.Parse(userEntry) < 0)
{
Console.WriteLine("You must enter a positive number.");
}
while (x < int.Parse(userEntry))
{
Console.Write("Yay!");
x++;
}
}
catch (FormatException)
{
Console.WriteLine("You must enter a whole number.");
continue;
}
}
Console.WriteLine("Code Execution Completed");
}
}
}
2 Answers
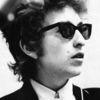
michaelcodes
5,604 PointsHello there is nothing wrong with your code, I had the same issue! For this specific challenge they actually do NOT want you to continue looping to ask for correct input. They only want you to catch the problems. Just get rid of the while loop and you should be all set.
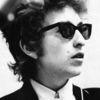
michaelcodes
5,604 PointsAwesome! I'm glad I was able to help. In practice continually prompting for the input seems like the logical thing to do, I guess we both just got a little ahead of ourselves adding in the while loop :)
Gian Lazzarini
3,263 PointsGian Lazzarini
3,263 PointsAwesome, thank you so much for the feedback! That makes sense.
Gian Lazzarini
3,263 PointsGian Lazzarini
3,263 PointsSeriously you are a lifesaver, I just went through the quiz and rewrote all of my answers more simply without the outer loop like you mentioned and was able to get through all of them without any trouble!
Here's what I came up with by the 3rd answer. It's pretty much the same but without nesting all of the code in a while loop like I have in the code above. Also I went ahead and used a for loop to refactor the "Yay!" iteration into fewer lines of code.