Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial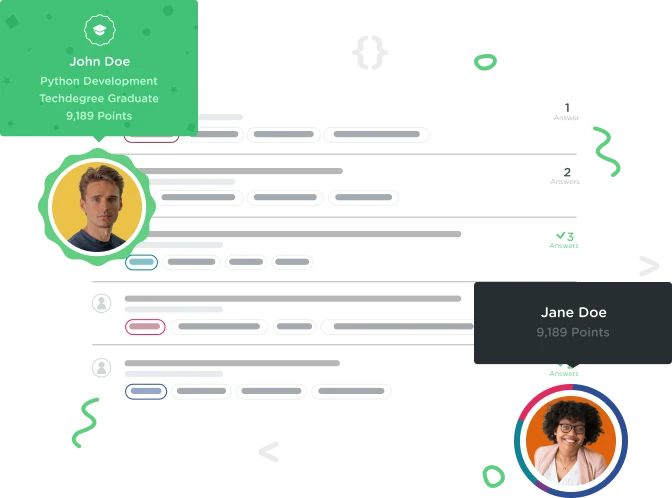

jbiscornet
22,547 PointsMy /cards page is not working. All other pages working properly.
I am working on express basics, I don't know why my cards page is not working. Everything else is working as it should but I can't find why I would be getting the error on that page.
const express = require('express');
const bodyParser = require('body-parser');
const cookieParser = require('cookie-parser');
const app = express();
app.use(bodyParser.urlencoded({ extended: false}));
app.use(cookieParser());
app.set('view engine', 'pug');
const mainRoutes = require('./routes');
const cardRoutes = require('./routes/cards');
app.use(mainRoutes);
app.use('/cards', cardRoutes);
app.use((req, res, next) => {
const err = new Error('Not Found');
err.status = 404;
next(err);
});
app.use((err, req, res, next) => {
res.locals.error = err;
res.status(err.status);
res.render('error', err);
});
app.listen(3000, () => {
console.log('The application is running on localhost:3000!')
});
const express = require('express');
const router = express.Router();
router.get('/', (req, res) => {
res.render('card', {prompt: "Who is buried in grant's tomb?", colors });
});
module.exports = router;
const express = require('express');
const router = express.Router();
router.get('/', (req, res) => {
const name = req.cookies.username
if (name) {
res.render('index', {name: name});
} else {
res.redirect('/hello');
}
});
router.get('/hello', (req, res) => {
const name = req.cookies.username
if (name) {
res.redirect('/');
} else
res.render('hello');
});
router.post('/hello', (req, res) => {
res.cookie('username', req.body.username);
res.redirect('/');
});
router.post('/goodbye', (req, res) => {
res.clearCookie('username');
res.redirect('/hello');
});
module.exports = router;
Thanks in advance for any help, J!
10 Answers

Chris Underwood
Full Stack JavaScript Techdegree Student 19,916 PointsI found an error in my card.pug file. So check there.
Specifically, I had the each loop for colors still in there. It could not find the object colors as it was still in the app.js file. I just removed the loop as we no longer needed it and the project came right up.

Neil McPartlin
14,662 PointsI replaced my card.js file with yours and it failed with 'Invalid status code: 0', so not quite the same as your error. But I note that in line 5, you are still referencing 'colors' even though that array is no longer present in app.js, so start by removing this. I did and your file now works with my other ones.
If you still have an error, take Chris Underwood 's advice and review your card.pug file.

jbiscornet
22,547 PointsThe problem ended up being in what you said Chris Underwood, it was the colors reference that was screwing up the whole thing. I took those two lines out and all the pages work as intended now. Thanks for the suggestions to everyone that tried on this. I was stuck on this problem for a long while, it's nice to be able to solve this one and move on.

Chris Underwood
Full Stack JavaScript Techdegree Student 19,916 PointsGlad my answer helped you. Beauty of Treehouse is that we are not alone in our coding journey.

Nick Edwards
18,388 PointsHey J Biz,
So in your cards.js file if you change the line router.get('/cards', (req, res) to instead say router.get('/', (req, res) it still doesn't work for you?
Because if I have '/cards' it gives me a 404 error, but if I only use '/' it works

jbiscornet
22,547 PointsI only put router.get('/cards', (req, res) for reference because I thought it didn't make a difference, good to know it does, thanks for that Nick. So if you leave it at router.get('/', (req, res)... when you say it works for you, it works with the address (http://localhost:3000/cards), and all the other (cards/1)... flashcard pages?
I get the same error either way, it says "This page isn't working the local host didn't send any data." I've checked over the code so many times and I haven't found any error compared to the way Andrew shows it in the video. It really puzzles me why I am not able to get these pages, especially when all others are working as intended with the same formatting. The only thing I am wondering is when I was trying to get a program installed that wouldn't install, after some research I ended up doing a forced clear cache of the terminal, which I don't think I should have done (live and learn), it didn't help install the program and I later found a different solution. I am wondering if it cleared something out I needed? But at the same time it works for me for all the other pages just fine, so I am lost as to why it is telling me there is no data for any of the cards route.

ac casc
Full Stack JavaScript Techdegree Student 3,778 PointsI will never learn any thing from this Chuckley guy again! Very disorganised.
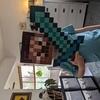
Gabbie Metheny
33,778 PointsAt least attempt to spell someone's name correctly before you insult them, ac casc.
I've been critical of some of Andrew's teaching, but I've found this course to be really excellent, possibly because Joel Kraft worked on it with him. Joel is probably my favorite Treehouse JavaScript teacher, and I think he complements Andrew well.
Out of curiosity, what about Andrew Chalkley's teaching have you found to be disorganized in this course? Personally, I think his explanations have been thorough, and his pace quite reasonable. It's also been great to build something from the ground up, in our own text editors, rather than jumping into preformed logic in Workspaces.

nguoisechia binnary
Full Stack JavaScript Techdegree Student 10,332 PointsYou probably haven't indent correctly of every line in the PUG file.

Recep Onalan
15,541 PointsAt start I also could not get the page /cards. Then in file app.js I imported routes like that:
//Import routes
const mainRoutes = require('./routes');
const cardRoutes = require('./routes/cards');
app.use(mainRoutes);
app.use(cardRoutes);
and in cards.js write my route like that:
router.get('/cards', (req, res) => {
res.render('card', { prompt: "Who is buried in Grant's tomb?" });
});
and it works

Amber Diehl
15,402 PointsI had this exact same problem, too--I was getting a 404 error. I tried what Recep did and it worked. On a whim, I decided to change it back, ensured I saved all changes at the same time, and it worked again the 'right' way. I am thinking that the nodemon might have restarted with the file changes out of sync or something and thus the failure.
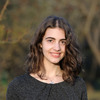
Larisa Popescu
20,242 PointsI have the same problem. I wrote the same code as shown in the video, and this is what I get
RangeError: Invalid status code: 0

Amanda Richardson
Web Development Techdegree Student 13,776 PointsI know this is late but just in case anyone else is having this problem - I struggled for a while to figure out why my /cards page wasn't displaying. It turned out I had accidentally added a random backslash in my card.pug file that was screwing everything up so definitely check that file and make sure there isn't an error somewhere in there.

glasscheck
7,302 PointsIt looks like you havn't made a route for cards in the cards file
router.get('/'
Steven Parker
241,809 PointsSteven Parker
241,809 PointsWhen posting code, always use the instructions for code formatting in the Markdown Cheatsheet pop-up below the "Add an Answer" area.
Or watch this video on code formatting.