Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial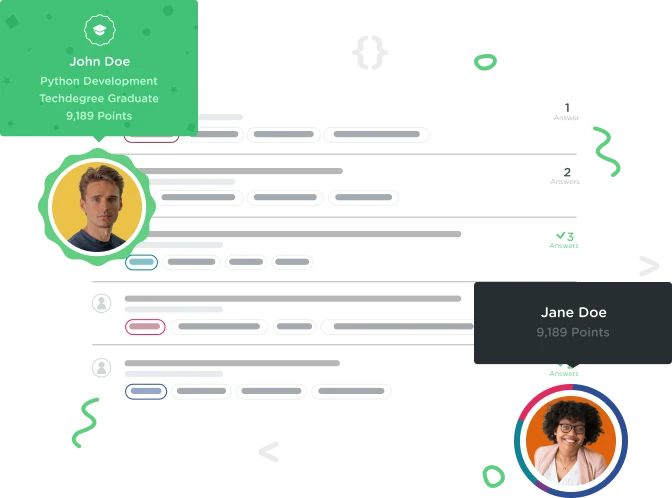
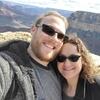
James Crosslin
Full Stack JavaScript Techdegree Graduate 16,882 PointsMy chargeFines solution took a more long term view of fines. I used a setInterval to automatically charge Patrons
This took quite a lot of independent searching around to learn .bind(), and I'm pretty proud of it. I managed to contain this auto-running daily charge within the object, so as soon as it is created, and without the need of any outside chargeFines call, the countdown starts. This solves the problem of having to match back dates and then charge based on a comparison, as it checks daily on its own and automatically fines patrons.
Let me know what you think and what you would've done differently!
class Library {
constructor(name) {
this.name = name
this.books = []
this.patrons = []
// binding 'this' to my 'this.chargeFines' was very important, because the asynchronous
// 'setInterval' method would cause 'this' to reference 'window' when the scope changes
this._autoCharge = setInterval(this.chargeFines.bind(this), 8.64e7)
this._dailyFine = 0.1
}
addBook(Book) {
this.books.push(Book)
}
addPatron(Patron) {
this.patrons.push(Patron)
}
chargeFines() {
const today = new Date()
for (let i = 0; i < this.books.length; i++) {
if (this.books[i].patron && this.books[i].dueDate < today) {
this.books[i].patron.balance += this._dailyFine
}
}
return "Successful auto-charge cycle complete."
}
}