Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial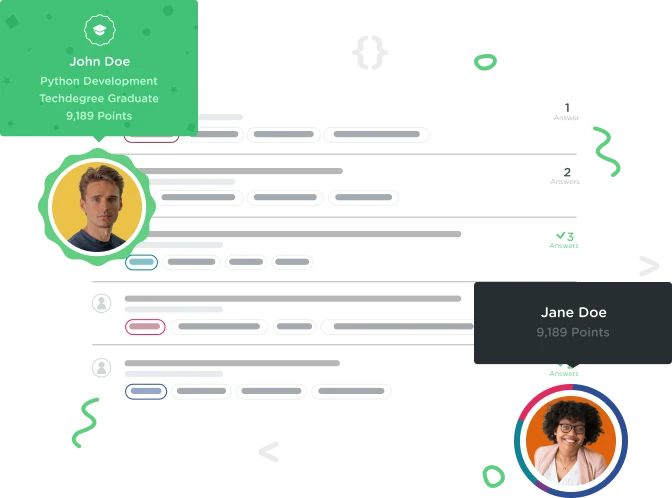

Denis Chernenko
4,077 Pointsmy Class + regular expressions is not working together
I expect to provide class 3 positional arguments, but have an error: TypeError: init() takes 1 positional argument but 2 were given
Please advice what need to be done correctly
import re
string = '''Love, Kenneth: 20
Chalkley, Andrew: 25
McFarland, Dave: 10
Kesten, Joy: 22
Stewart Pinchback, Pinckney Benton: 18'''
players_compiled = re.compile(r"""
^(?P<last_name>[\w ]*)
,\s
(?P<first_name>[\w ]*)
:\s
(?P<score>[\d]*)$
""", re.X|re.M)
class Player:
def __init__(self, **kwargs):
for key, value in kwargs.items():
if key == "first_name":
self.first_name = value
elif key == "last_name":
self.last_name = value
elif key == "score":
self.score = value
something = Player(players_compiled.finditer(string))
print (something)
2 Answers

Greg Kaleka
39,021 PointsHi Denis,
I think you're over-thinking this a bit. You don't need to dynamically set the Player's attributes - you know what they will be. A simple init statement is all you need. It should accept last_name
, first_name
and score
as arguments.
I see what you're trying to do, which is pretty clever, although as your code is written, you're just passing an iterable object to init, which is why you're getting that error. You haven't passed any kwargs. Jump into a python shell to prove this to yourself.
>>> players_compiled.finditer(string)
<callable-iterator object at 0x10e4dd410>
Again, there's no reason not to set the arguments explicitly in your init, but if you did want to just accept **kwargs, you would need a for loop and groupdict()
. If you want a little project, go back to the previous video and see if you can figure out how to create instances of Player rather than printing the dictionary. Hint - you'll need to use ** on the dictionary...
Let me know if this all makes sense!
Cheers
-Greg
class Player:
def __init__(self, last_name, first_name, score):
self.last_name = last_name
self.first_name = first_name
self.score = score

Denis Chernenko
4,077 PointsHi Greg,
Thank you for explanation. Actually i've passed exam but i want to understand how classes + regular expressions can work together with multiple number of arguments like it happens in real life :)
I would really appreciate other code example if it possible to use class to get some data(tuple, dictionary) from imported file.