Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial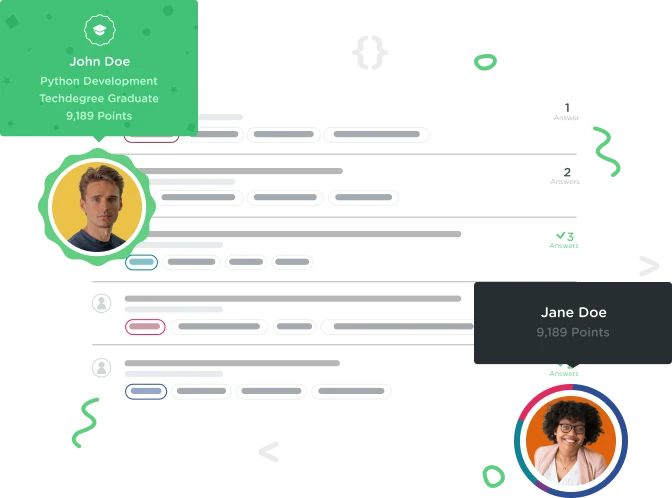
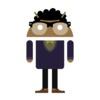
Tim McKyer
10,989 PointsMy code ...
A little help here lol
public class Example {
public static void main(String[] args) {
ShoppingCart cart = new ShoppingCart();
Product pez = new Product("Cherry PEZ refill (12 pieces)");
cart.addItem(pez, 5);
/* Since a quantity of 1 is such a common argument when adding a product to the cart,
* your fellow developers have asked you to make the following code work, as well as keeping
* the ability to add a product and a quantity.
*/
Product dispenser = new Product("Yoda PEZ dispenser");
/* Uncomment the line following this comment,
after adding a new method using method signatures,
to solve their request in ShoppingCart.java
*/
public void addItem(Product item, int quantity) {
System.out.printf("Adding %d of %s to the cart.%n", quantity, item.getName());
cart.addItem(dispenser);
}
public void addItem(Product item)
{
addItem(item,1);
}
}
public class ShoppingCart {
public void addItem(Product item, int quantity) {
System.out.printf("Adding %d of %s to the cart.%n", quantity, item.getName());
/* Other code omitted for clarity. Please imagine
lots and lots of code here. Don't repeat it.
*/
}
}
public class Product {
/* Other code omitted for clarity, but you could imagine
it would store price, options like size and color
*/
private String mName;
public Product(String name) {
mName = name;
}
public String getName() {
return mName;
}
}
3 Answers

Aaron Arkie
5,345 PointsHello Tim! You're in the right mindset! First lets rearrange the code on how it is presented in the original question. Your method addItem in the example class seem to be in the incorrect class. Since we know a shopping cart's sole function is to add and subtract items from the cart then it should contain a method to complete this process hence having the addItem method in the class Shopping cart.
public class Example {
public static void main(String[] args) {
ShoppingCart cart = new ShoppingCart();
Product pez = new Product("Cherry PEZ refill (12 pieces)");
cart.addItem(pez, 5);
/* Since a quantity of 1 is such a common argument when adding a product to the cart,
* your fellow developers have asked you to make the following code work, as well as keeping
* the ability to add a product and a quantity.
*/
Product dispenser = new Product("Yoda PEZ dispenser");
/* Uncomment the line following this comment,
after adding a new method using method signatures,
to solve their request in ShoppingCart.java
*/
cart.addItem(dispenser);
}
}
public class ShoppingCart {
public void addItem(Product item, int quantity) {
System.out.printf("Adding %d of %s to the cart.%n", quantity, item.getName());
/* Other code omitted for clarity. Please imagine
lots and lots of code here. Don't repeat it.
*/
}
public void addItem(Product item){
}
}
public class Product {
/* Other code omitted for clarity, but you could imagine
it would store price, options like size and color
*/
private String mName;
public Product(String name) {
mName = name;
}
public String getName() {
return mName;
}
}

Aaron Arkie
5,345 PointsOkay now that we have our addItem method in the correct class let's take a look at what we need. First off you were correct about needing to create another addItem method in the shopping cart class. The first addItem method takes two parameters, one that accepts a class which holds a String. This can be seen in the Product class that has a constructor that "demands" a data type of a String. The second parameter asks for an int which the user will set manually when using the method.
Now to the problem at hand. We know from the Example class we are supposed to uncomment the line cart.addItem(dispenser) once we have created a new method that ONLY accepts a variable of whatever dispenser is. What is dispenser? Dispenser is an object of the Product class. We know from the previous method that takes in two parameters allows for a variable with a type of class to be accepted. So this is why you created a method that only takes one parameter of what the item is. Furthermore, we also know that we need a method that only requires one item to be added to the cart.
public void addItem(Product item)
{
// addItem(item,1); error is here
}

Aaron Arkie
5,345 PointsNow that we have an addItem method that only asks for a product object we can focus on the task at hand. We need to find a way to store only one item in the cart. We know that before in the first method which accepts two parameters one of them asked for a quantity. So we initially had to set a value that was an int into the method. The number we chose was 5. However, since we only need one item it is no longer necessary to have a second parameter that accepts an int since it will always be just one.
Now lets ask ourselves, how can we store a value locally in a method that is not required to be set by the user each time but will always contain a specific value.
public void addItem(Product item){
//I need an int but i need something to hold it in with a value of 1
//then i need a display message that outputs the information i put in (see first addItem method)
}
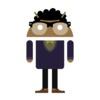
Tim McKyer
10,989 PointsHey man, it's still not working and I'm still not quite understanding where you are coming from lol xD