Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial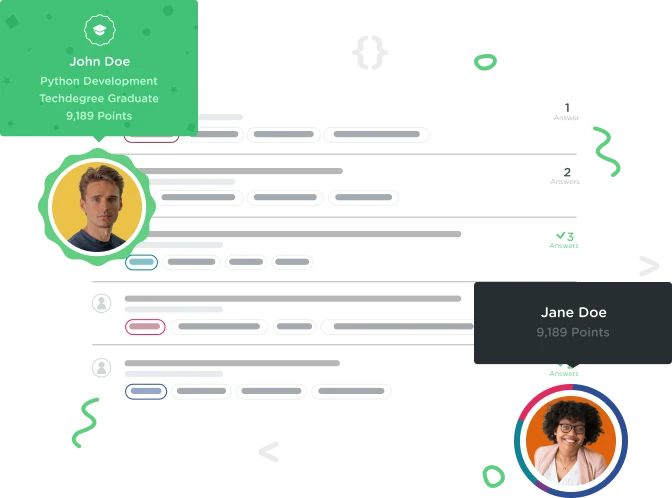
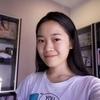
Amber Lim
4,707 PointsMy code does not print out anything after I tried to add the "print out student with same names" feature.
I created an empty array foundStudents
to "push" the students with the same names. Nothing was printed onto the screen. I'd appreciate any pointers.
//establishing variables
var message = '';
var student;
var search;
var foundStudents = [];
//functions
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getStudentReport( student ){
var report = '';
for(var i = 0; i<student.length; i++){
report += '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
}
return report;
}
//looping through students' information
while(true){
search = prompt("Type a student's name to access their records or type 'quit' to quit this search.");
if( search === null || search === 'quit' ){
break;
}
for (var i = 0; i < students.length; i += 1){
student = students[i];
if(search.toLowerCase === student.name.toLowerCase()){
foundStudents.push(student);
}
}
if(foundStudents.length === 0){
alert(student.name + "is not on our records.");
} else {
message = getStudentReport(foundStudents);
print(message);
}
}
2 Answers
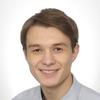
Sergey Podgornyy
20,660 PointsAbsolutely agree with Ashish, but I change your code according to JavaScript best practices:
// Fill test data about students
var students = [
{
name: 'Aaron',
track: 'JavaScript',
points: 500,
achievements: []
}, {
name: 'Tom',
track: 'PHP',
points: 1500,
achievements: []
}, {
name: 'Stewie',
track: 'Ruby',
points: 50,
achievements: []
}
];
var foundStudents = [];
// Functions declaration
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getStudentReport(students) {
return students.reduce(function(sum, current) {
return sum
+ '<h2>Student: ' + current.name + '</h2>'
+ '<p>Track: ' + current.track + '</p>'
+ '<p>Points: ' + current.points + '</p>'
+ '<p>Achievements: ' + current.achievements + '</p>';
}, '');
}
// Searching for students
while (true) {
var search = prompt("Type a student's name to access their records or type 'quit' to quit this search.");
if (search === null || search === 'quit') {
break;
}
students.forEach(function(item, index) {
if (search.toLowerCase() === item.name.toLowerCase()) {
foundStudents.push(item);
}
});
if (foundStudents.length) {
var message = getStudentReport(foundStudents);
print(message);
} else {
alert(search.name + "is not on our records.");
}
}
You could test it online here
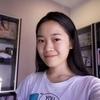
Amber Lim
4,707 PointsThanks for the help, Sergey, and pointing out my errors. Really appreciate it. Your code is a lil' complex for my brain to handle right now. I hope one day I can read your code like I read a book. :')
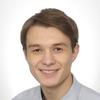
Sergey Podgornyy
20,660 PointsAmber, if you will practice every day, it will be very soon You just need to learn
Callback
topic

paulos kesete
Courses Plus Student 2,623 Pointsvar foundStudents = []; var search;
alert( search + " is not on our records.");
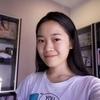
Amber Lim
4,707 PointsAshish, by student[i].property what do you mean? Can you please elaborate? Perhaps leave comments in the actual code where this advice can be applied. Because I don't understand how student[i].property
should be used in the code. Thank you so much!
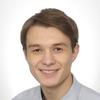
Sergey Podgornyy
20,660 PointsHi Ashish, I'll answer for Ashish. Hope this is Ok?
In your code in function getStudentReport()
inside for
loop you wrote just:
for(var i = 0; i<student.length; i++){
report += '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
}
But in this case student
is an array, and you should specify which element you wanna display, so you need to specify an index:
for(var i = 0; i<student.length; i++){
report += '<h2>Student: ' + student[i].name + '</h2>'
+ '<p>Track: ' + student[i].track + '</p>'
+ '<p>Points: ' + student[i].points + '</p>'
+ '<p>Achievements: ' + student[i].achievements + '</p>';
}
Same as you reassign here:
for (var i = 0; i < students.length; i += 1){
student = students[i]; // <<== HERE
if (search.toLowerCase() === student.name.toLowerCase()) {
foundStudents.push(student);
}
}
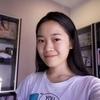
Amber Lim
4,707 PointsSergey! Thank you so freaking much for the encouragement and solution. It helped. Really appreciate it!
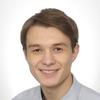
Sergey Podgornyy
20,660 PointsYou are welcome
Ashish Singh
452 PointsAshish Singh
452 PointsHey Amber Lim , Ok I caught some errors in your code