Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial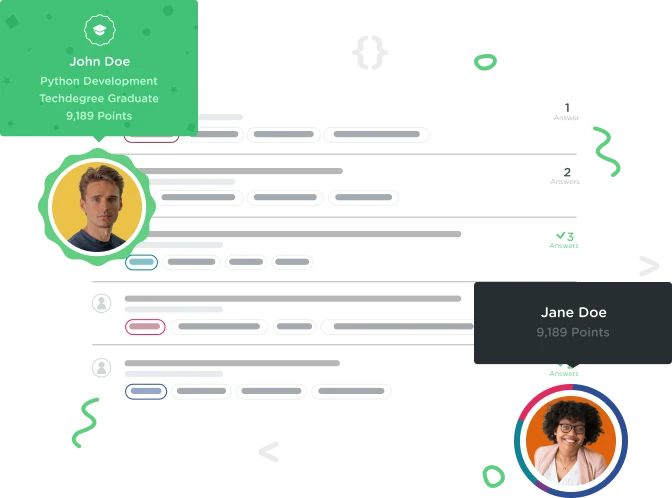

Enemuo Felix
1,895 PointsMy code doesn't print alright
The code runs but the correctAnswer and wrongAnswer array doesnt print. What could be the issue?
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML += message;
}
function buildList(array) {
var htmlList = '<ol>';
for(var q = 0; q < array.length; q += 1) {
htmlList += '<li>' + array[q] + '</li>';
}
htmlList += '</ol>';
return htmlList;
}
var html;
var count = 0;
var questions = [
['What letter is the first alphabet?', ' a'],
['Who won the last world cup?', 'germany'],
['What is the capital of germany?', 'berlin']
];
var correctAnswer = [];
var wrongAnswer = [];
for (var i = 0; i < questions.length; i +=1) {
var userInput = prompt(questions[i][0]);
if(questions[i][1].indexOf(userInput.toLowerCase() ) > -1) {
correctAnswer.push(questions[i][0]);// trying to push the correct input into the correctAnswer array//
count+=1;
print
} else {
wrongAnswer.push(questions[i][0]);// trying to apply the same with line 26 but this time in the wrongAnswer array//
}
html = 'You got ' + count + ' question(s) correctly out of 3';
}
print(html);
print('<h2>You got these question(s) correct ;</h2>');
html+= buildList(correctAnswer);// to print out the correctAnswer to the page with each of them numbered//
print('<h2>You got these question(s) wrong ;</h2>');
html+= buildList(wrongAnswer);//same with line 36//
3 Answers
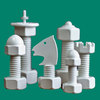
Steven Parker
231,846 PointsYou copy the lists into a string variable instead of printing them to the page. Try this instead:
print('<h2>You got these question(s) correct ;</h2>');
print(buildList(correctAnswer)); // to print out the correctAnswer to the page with each of them numbered//
print('<h2>You got these question(s) wrong ;</h2>');
print(buildList(wrongAnswer)); //same with line 36//
Or, just save the print call until the string has been completely assembled:
print('<h2>You got these question(s) correct ;</h2>');
html += buildList(correctAnswer); // to print out the correctAnswer to the page with each of them numbered//
print('<h2>You got these question(s) wrong ;</h2>');
html += buildList(wrongAnswer); //same with line 36//
print(html); // <-- moved down 4 lines
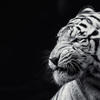
Nick Gentile
4,237 PointsAlso, do what Stephen said. You are running print(html) before you add the answers to your html when you do this:
html+= buildList(correctAnswer);
This matters big time in javascript. What comes first plays a huge role (this is a small example of a RACE CONDITION) -- when something you need to happen before you can do something else isn't happening before you do that 'something else'. Here you're printing your HTML, and then setting your HTML, so you're basically printing unset HTML (nothing)
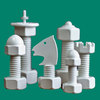
Steven Parker
231,846 PointsThe term "race condition" only applies to situations that occur in concurrent (threaded) code. What's happening here is completely sequential, and simply a matter of "order of execution".
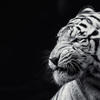
Nick Gentile
4,237 PointsWell the first thing I noticed right away was this line:
if(questions[i][1].indexOf(userInput.toLowerCase() ) > -1) {
Which is a bit of a mess. What I think you are trying to do, is say, if the user's input is equal to the answer for the particular array item you are iterating then do something.
Despite what people may say I'm going to show you how I would write this and then break it down, as it looks like you're trying to do the same thing multiple times in your IF statement, which is breaking your code ( if you run it in a env you get the following error ) : VM893:24 Uncaught TypeError: Cannot read property 'toLowerCase' of null at <anonymous>:24:40
if(questions[i][1] === userInput.toLowerCase()) {
First off, as you can see, you don't need to use indexOf.
You use indexOf to get the index of something within an array, but you're already doing that by using [i][0].
What you're essentially saying is when you call QUESTIONS[i], is the equivalent to questions.indexOf(0) (when i is 0, that is), which returns the following:
['What letter is the first alphabet?', ' a']
When you add the second [1] on you are then iterating through that first question (above).
So when you say 'questions[i][1]', you're you have grabbed the answer for that question, 'a'. There's no need to do any further indexing at this point, you just need to find out if 'a' is equal to what the user input.
What the user input has already been handled by you when you made your VAR USERINPUT. So all you have to do now is ask, is the answer ( questions[i][1]), equal to what the user input?
That's what the code I showed is doing. Read through this and respond if you have questions. Once you understand what my code means plug it in and try. It may not solve your task right away but it will clear away the biggest hiccup I see. You are very close.

Enemuo Felix
1,895 PointsThank you very much Nick for taking the time to help out. Although, I got the idea of what you're trying to say but i don't think i have had any issue with the code or that part of the code until i decided to add the buildList function()
to the code. If i get you correctly , do you mean to say that the code will only run on some selected IDE? I'm sorry I'm only a noob, so I haven't heard of 'env'
Enemuo Felix
1,895 PointsEnemuo Felix
1,895 PointsThank you so much Steven Parker and Nick Gentile for you awesome answers. I tried the second way as suggested by nick but the whole thing was messed up, because the output was not what i wanted. I copied and pasted the result below;
I guess it has something to do with my arrangement of calling the print function towards the end of my code. But everything worked perfectly after i used Steven's first suggestion. Just like this