Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial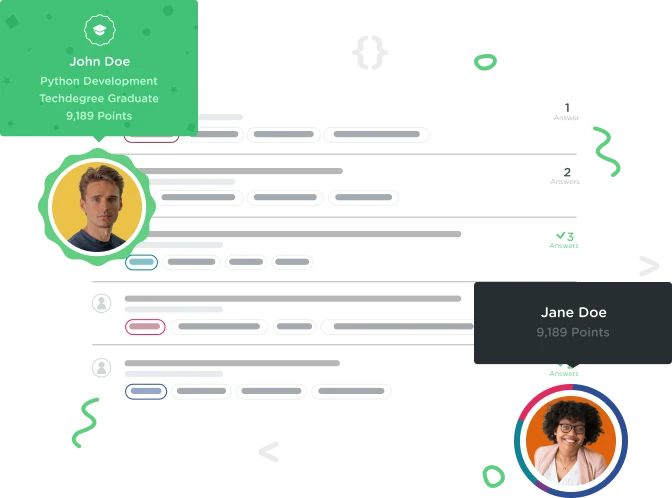

Braeden Long
2,137 PointsMy code executes correctly, but could it be improved? Final challenge for JavaScript Loops, Arrays and Objects
Hello, forums! I hope I put this in the right place. I have just written a block of code that executes the two challenges left at the end of JavaScript Loops, Arrays, and Objects. The challenges were to (1) print out the information for multiple students that have the same name and (2) alert the user when the input provided was not a student's name.
Here is my final product:
var message = '';
var student;
var sameStudents;
var search;
var multipleStudents = [];
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function printStudentReport(student) {
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
return report;
}
while (true) {
search = prompt("Search for a student's records by name [i.e. Dave]. If you wish to view the results, type quit.")
if (search === null || search.toLowerCase() === 'quit') {
break;
}
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if (search.toLowerCase() !== student.name.toLowerCase()) {
alert("The input you have provided did not provide any results. Please try again.")
break;
}
break;
}
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if (search.toLowerCase() === student.name.toLowerCase()) {
multipleStudents.push(student);
}
}
for (var i = 0; i < multipleStudents.length; i += 1) {
sameStudents = multipleStudents[i];
message += printStudentReport(sameStudents);
}
}
print(message);
If you guys see anything that I can improve on please let me know! I'm really proud to have accomplished this challenge.
Thanks, everyone!
1 Answer
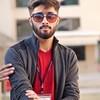
Huzaifa Sajjad Malik
Courses Plus Student 10,823 Pointshere is a better approach
var message = '';
var student;
var search;
var check=false;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function generate(student)
{
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
return report;
}
while(true)
{
search=prompt("Enter Student name or quit to exit");
if(search === null || search.toLowerCase() === "quit")
break;
else
{
for (var i = 0; i < students.length; i += 1)
{
student = students[i];
if(student.name === search)
{
check=true;
message+=generate(student);
}
}
if(check)
{
alert("Name Found! Displaying Your Data");
break;
}
else
{
alert("Name not Found!");
continue;
}
}
}
print(message);
Kingsley Felix
8,591 PointsKingsley Felix
8,591 PointsThanks