Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial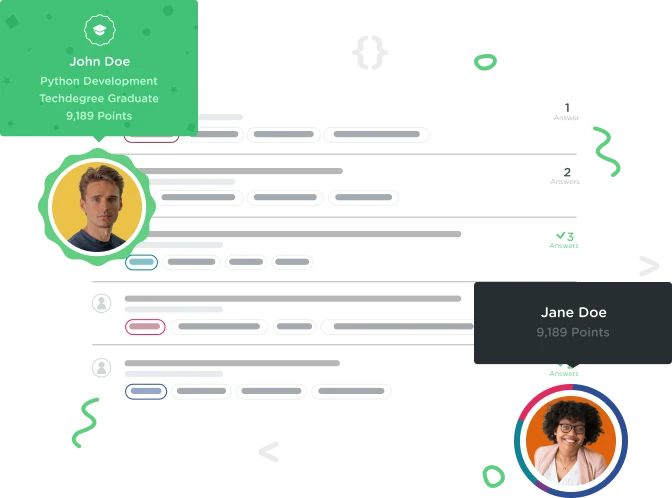
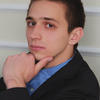
Iskander Ismagilov
13,298 PointsMy code for number_game is far cry from Kenneth's. Appreciate for any feedback. And one question if condition.
My:
chance_list = []
import random
random.randint(1, 10)
num = random.randint(1, 10)
def show_help():
print("Guess a number between 1 to 10. You have 5 chances.")
def output_num(num):
print(num)
def add_to_list(new_num):
chance_list.append(new_num)
print("You used {} chances.".format(len(chance_list)))
show_help()
while True:
new_num = input(">")
if len(chance_list) == 5:
break
elif int(new_num) == num:
print("Congratulations!")
break
elif int(new_num) > num:
print("Your number is higher than should be.")
add_to_list(new_num)
continue
elif int(new_num) < num:
print("Your number is lower than should be.")
add_to_list(new_num)
continue
Kenneth's:
import random
rand_num = random.randint(1, 10)
guessed_nums = []
allowed_guesses = 5
while len(guessed_nums) < allowed_guesses:
guess = input("Guess a number between 1 and 10: ")
try:
player_num = int(guess)
except:
print("That's not a whole number!")
break
if not player_num > 0 or not player_num < 11:
print("That number isn't between 1 and 10!")
break
guessed_nums.append(player_num)
if player_num == rand_num:
print("You win! My number was {}.".format(rand_num))
print("It took you {} tries.".format(len(guessed_nums)))
break
else:
if rand_num > player_num:
print("Nope! My number is higher than {}. Guess #{}".format(
player_num, len(guessed_nums)))
else:
print("Nope! My number is lower than {}. Guess #{}".format(
player_num, len(guessed_nums)))
continue
if not rand_num in guessed_nums:
print("Sorry! My number was {}.".format(rand_num))
Why doesn't last condition 'if' print out "Sorry! My number was {}.", if a player makes a mistake first time he put in a number? It prints it out after 5 tries done. But his number is already in guessed num list and it's not a rand_num.
if not rand_num in guessed_nums:
print("Sorry! My number was {}.".format(rand_num))
2 Answers
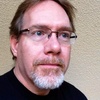
Chris Freeman
Treehouse Moderator 68,441 PointsWith 2-space indentation instead of 4-space, it's not as obvious how the code aligns. The final if
statement has the same indentation as the while
statement. This means the if
will not execute until the while
has run its course and ended with too many guess or one of the break
statements.
Then the if
will do a final check to see if the number was guessed (the rand_num
is found in the guessed_nums
list)
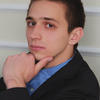
Iskander Ismagilov
13,298 PointsThank you, Chris.