Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial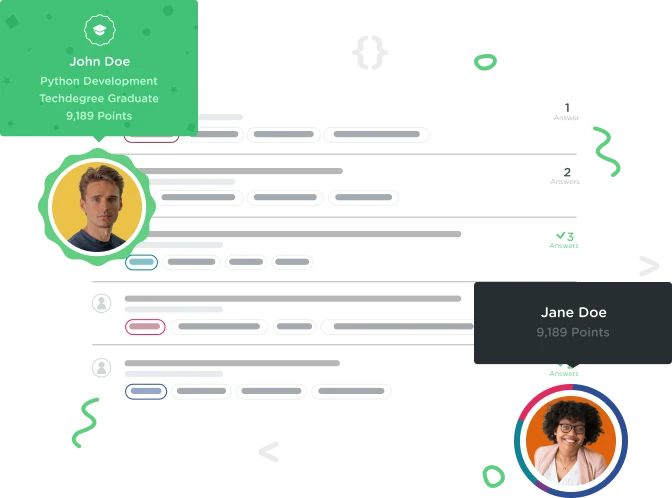
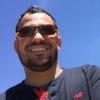
Wameedh Mohammed Ali
16,572 PointsMy Code for the Challenge!
var html = '';
var red;
var green;
var blue;
var rgbColor;
for (var i = 0; i < 10; i += 1) {
red = Math.floor(Math.random() * 256 );
green = Math.floor(Math.random() * 256 );
blue = Math.floor(Math.random() * 256 );
rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')';
html += '<div style="background-color:' + rgbColor + '"></div>';
}
document.write(html);
1 Answer

Gunhoo Yoon
5,027 PointsMine
//Generated html string.
var html = '';
//Returns random number between 0 to max
function randomNumber(max) {
return Math.floor(Math.random() * max + 1);
}
//Returns CSS rgb() function as string e.g)'rgb(r, g, b)'
function randomRGB() {
return 'rgb(' + randomNumber(255) + ',' +
randomNumber(255) + ',' +
randomNumber(255) + ')';
}
//Returns pre-generated div with background style.
//Input: CSS color value as string. e.g)'rgb(r, g, b)', '#000000', 'rgba(r, g, b, o)', 'red'
//(-corrected by Iain Simmons)
//Output: '<div style="background-color: rgb(75, 84, 150)></div>'
function getColorBoxHTML(rgb) {
return '<div style="background-color: ' + rgb + '"></div>';
}
//Append colorBox n times.
for (var i = 0; i < 10; i += 1) {
html += getColorBoxHTML(randomRGB());
}
//Apply to document.
document.write(html);
Don't forget to properly indent code after for loop.
Iain Simmons
Treehouse Moderator 32,305 PointsIain Simmons
Treehouse Moderator 32,305 PointsGood clean code, well commented.
One thing to point out though, due to the modular/functional nature of your code, the
getColorBoxHTML
function can accept any input string of CSS that is a valid value of thebackground-color
property, including color keywords, hex values,rgb()
andrgba()
. So your input/output comments don't reflect all the things it can do! :)Gunhoo Yoon
5,027 PointsGunhoo Yoon
5,027 PointsOh yeah thanks for commenting that out I originally called randomRGB() insdie getColorBoxHTML() then I decided to be open and forgot to change the comment after tweaking it, stupid me.