Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial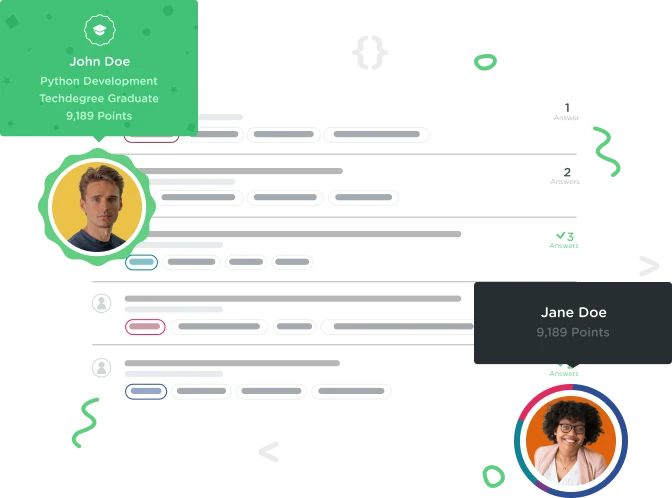
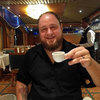
Josh Prakke
4,412 PointsMy code for the Conditional Challege
Here's my take on the challenge. What do you guys think?
/*
JavaScript: Five Question Quiz
Multiple question quiz with
various JavaScript Questions
By: Josh Prakke
Date: 04/07/2017
---- Cheat Sheet ----
1. boolean
2. dry programing
3. strict equality operator
4. modulus
5. concatenation
*/
console.log("Program Begins");
//Prompts user with introduction to quiz and first question
var testConfirm = prompt("Would you like to take a quick quiz on some JavaScript basics?");
var correctAnswers= 0;
console.log(correctAnswers + " correct answers total.");
if (testConfirm.toUpperCase() === "YES" || testConfirm.toUpperCase() === "Y") {
var firstAnswer = prompt("What JavaScript condition can only be \"True\" or \"False\"?");
} else if (testConfirm.toUpperCase() === "NO" || testConfirm.toUpperCase() === "N"){
alert("Okay thanks anyway. Have a nice day!");
} else {
alert("Please enter a valid statement either \"Yes\" or \"No\".");
location.reload();
console.log("Program Ends");
}
/*
Produces questions and records
if correct via correctAnswers variable
*/
if (firstAnswer.toUpperCase() === "BOOLEAN" || firstAnswer.toUpperCase() === "BOOLEANS"){
correctAnswers += 1;
}
console.log(correctAnswers + " correct answers total.");
var secondAnswer = prompt("What is it called when you repeat the same line of code twice?");
if (secondAnswer.toUpperCase() === "DRY PROGRAMMING") {
correctAnswers += 1;
}
console.log(correctAnswers + " correct answers total.");
var thirdAnswer = prompt("What is this operator called \" === \"?");
if (thirdAnswer.toUpperCase() === "STRICT EQUALITY OPERATOR"){
correctAnswers += 1;
}
console.log(correctAnswers + " correct answers total.");
var fourthAnswer= prompt("What is this operator called \" % \"?");
if (fourthAnswer.toUpperCase() === "MODULUS") {
correctAnswers += 1;
}
console.log(correctAnswers + " correct answers total.");
var fifthAnswer= prompt("What is it called when you add multiple variables or strings together?");
if (fifthAnswer.toUpperCase() === "CONCATENATION"){
correctAnswers += 1;
}
console.log(correctAnswers + " correct answers total.");
/*
determines ammount of
correct answers and
rewards user accordingly
*/
if (correctAnswers === 5){
document.write("<p>Congratulations! You got " + correctAnswers + " out of 5 questions right! You have earned the Gold Medal!</p>");
} else if (correctAnswers >= 3){
document.write("<p> Good job! You got " + correctAnswers + " out of 5 questions right! You have earned the Silver Meadl!</p>");
} else if (correctAnswers >= 1){
document.write("<p> You got " + correctAnswers + " out of 5 questions right. You have earned the Bronze Meadal.</p>");
} else{
document.write("<p>You got " + correctAnswers + " out of 5 questions right. Maybe you should study up then come try again!</p>");
}
console.log("Program Ends");
1 Answer
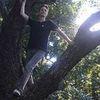
Gyorgy Andorka
13,811 PointsGood job! Maybe that's just me, but if not necessary, instead of .toUpperCase()
I'd rather use .toLowerCase()
on the answers, since uppercase is a convention for naming constants, and full uppercase string literals can mislead the eye of a programmer (after a quick glance, I instantly looked for the definition of the MODULUS and BOOLEAN constants, and was confused for a moment, then I realized I was mistaken :) )
However, there is one part which is problematic and definitely needs to be improved:
if (testConfirm.toUpperCase() === "YES" || testConfirm.toUpperCase() === "Y") {
var firstAnswer = prompt("What JavaScript condition can only be \"True\" or \"False\"?");
// okay... and then?
} else if (testConfirm.toUpperCase() === "NO" || testConfirm.toUpperCase() === "N"){
alert("Okay thanks anyway. Have a nice day!");
} else {
alert("Please enter a valid statement either \"Yes\" or \"No\".");
location.reload();
console.log("Program Ends");
}
This is ugly and very confusing code. After the if
statement you should either play the whole quiz, then continue with the other branches, or leave it as the last option (else
), and ask all the questions there in one place. (In the latter case, you would check in the else if
branch if the answer for the "woud you like to play" question was not a valid statement [i.e. "Y", "YES", "N", or "NO"]; for this, I would use membership check in an array - see below.)
I'm not sure if you've learned about functions yet, but having said the above, the most obvious refactoring would be to pack the whole question-answer section into a function, and just call it after the if
statement:
...
if (testConfirm.toUpperCase() === "YES" || testConfirm.toUpperCase() === "Y") {
playQuiz();
} else if ...
...
function playQuiz() {
var firstAnswer = prompt("What JavaScript condition can only be \"True\" or \"False\"?");
if ((firstAnswer.toUpperCase() === "BOOLEAN" || firstAnswer.toUpperCase() === "BOOLEANS") {
correctAnswers += 1;
}
console.log(correctAnswers + " correct answers total.");
var secondAnswer = prompt("What is it called when you repeat the same line of code twice?");
if (secondAnswer.toUpperCase() === "DRY PROGRAMMING") {
...
}
...
Arrays and data structures will also come later, but note that in the case of the yes/no prompts and the first question, you could first declare the array of possible options, then simply check if your answer is among them - this is especially useful if you have a lot of correct options, and you don't want to check all of them independently (Array.indexOf(<item>)
returns -1 if the item is not found in the array):
if (["boolean", "booleans"].indexOf(firstAnswer.toLowerCase()) !== -1) {
correctAnswers += 1;
}
// For this, you could also use `Array.includes()`, although it's not supported by all browsers yet:
if (["boolean", "booleans"].includes(firstAnswer.toLowerCase()) {
correctAnswers += 1;
}
Also note: DRY programming means you try not to repeat the same line of code twice, it is actually a good practice ("Don't Repeat Yourself") :)
Keep up the good work!
Josh Prakke
4,412 PointsJosh Prakke
4,412 PointsGyorgy Andorka , thanks for the reply! May i say to start the amount of constructive criticism you had was astounding and so relieving. I did not expect that at all and I really appreciate it! I had only used the .toUpperCase() because that's what has been taught i didn't realize it could conflict visually with naming constants. To me the .toUpperCase() string literals looked ugly to begin with it I'm glad I can freely go to .toLowerCase().
As for the bit of code below:
I can definitely say I agree with you and it was not my proudest moment as of yet. I wasn't sure how else to do it until you mentioned putting it at the end with (else) then it clicked and it will be fixed as soon as I'm done typing this response!
As of right now the course just touched on if, else if, else statements and the &&, || operators. We have not learned about arrays or functions yet but from the bit I've read from your comment I'm looking forward to learning about them!
Thank you again for all your help I really appreciate it!
Josh
Josh Prakke
4,412 PointsJosh Prakke
4,412 PointsI updated it with your recommendations.
Gyorgy Andorka
13,811 PointsGyorgy Andorka
13,811 PointsNice! Be aware that even this version would (and should) be further improved in "real life". Note that inside the quiz function there is still a lot of repetition (which again, conflicts with the DRY principle) - we basically do the same thing X times (only the question and the answer differs). This is the kind of situation where you would use some kind of a "loop", but in order to do that, you need to store your question-answer pairs in some kind of data structure first, which you'll give to your function as "argument". Don't worry, in one or two months, these will be as natural as breathing, I just wanted to mention them here.
DRY is not simply for the sake of brevity. Following this principle is a must, because it reduces the number of possible errors in the code. At the moment, if you'd like to change anything in the process of the quiz function, then you have to do it in five different places - e.g. you had to switch
.toUpperCase()
to.toLowerCase()
five times in the second version (and imagine if you have a hundred or more questions...).Also, great to see the informative comments in your code, this is an important practice. Even if the general paradigm today is to use descriptive, longer variable names as much as possible, and only use comments if something is not obvious from the code itself, comments in a healthy amount are always needed.