Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial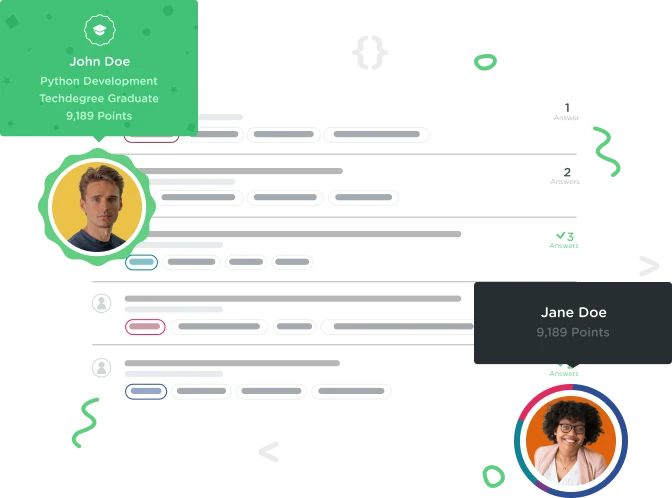
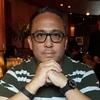
hapaman
Full Stack JavaScript Techdegree Student 3,026 PointsMy code for this challenge - Is this overengineered?
Any feedback is highly appreciated. I added code with my comments and commented out console.logs to explain and also show how my mind was working while trying to figure this out:
//Code with comments and console.logs
//user's answer
var ans;
//the correct answer
var correctAns;
//correct answer count
var correctAnsCnt = 0;
//incorrect answer count
var incorrectAnsCnt = 0;
//Final message showing how many answers were correct
var correctAnsMsgBeg = '<h1>You answered ';
var correctAnsMsgEnd = ' out of 3 questions correctly!</h1>';
//Questions and answers arrays
var qAndA = [
['What number comes after 1?',2],
['How many letters are in the alphabet?', 26],
['What year is it?',2019]
];
//Print message function
function print(message) {
document.write(message);
}
//Looping through questions and answers
for(var i = 0; i < qAndA.length; i++) {
//assign correct answer to var correctAns
correctAns = qAndA[i].pop();
//console.log("The correct answer is (correctAns): " + correctAns);
//answer given by user assigned to var ans
ans = parseInt(prompt(qAndA[i]));
//console.log("Submitted answer (ans): " + ans);
//comparing user answer versus correct answer
if(ans === correctAns) {
//if answer is correct, add to correct answer count
correctAnsCnt++;
//console.log("Correct Answer Count: " + correctAnsCnt);
//print correct message statement
print("<p>Answered <strong>Correctly:</strong> " + qAndA[i] + "</p>");
} //if answer is incorrect...
else {
//add to incorrect answer count
incorrectAnsCnt++;
//console.log("Incorrect Answer Count: " + incorrectAnsCnt);
//print incorrect message statement
print("<p>Answered <strong>Incorrectly:</strong> " + qAndA[i] + "</p>");
}
} //when loop condition fails, print final message below
print(correctAnsMsgBeg + correctAnsCnt + correctAnsMsgEnd);
//Code without comments and console.logs
var ans;
var correctAns;
var correctAnsCnt = 0;
var incorrectAnsCnt = 0;
var correctAnsMsgBeg = '<h1>You answered ';
var correctAnsMsgEnd = ' out of 3 questions correctly!</h1>';
var qAndA = [
['What number comes after 1?',2],
['How many letters are in the alphabet?', 26],
['What year is it?',2019]
];
function print(message) {
document.write(message);
}
for(var i = 0; i < qAndA.length; i++) {
correctAns = qAndA[i].pop();
ans = parseInt(prompt(qAndA[i]));
if(ans === correctAns) {
correctAnsCnt++;
print("<p>Answered <strong>Correctly:</strong> " + qAndA[i] + "</p>");
} else {
incorrectAnsCnt++;
print("<p>Answered <strong>Incorrectly:</strong> " + qAndA[i] + "</p>");
}
}
print(correctAnsMsgBeg + correctAnsCnt + correctAnsMsgEnd);
3 Answers
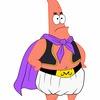
<noob />
17,062 PointsIn order to help u need to correctly format ur code.
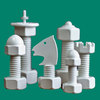
Steven Parker
230,274 PointsFor future expandability, you might not want to alter qAndA, so you could access the inner elements with a second index instead of using "pop". My other suggestion is to use consistent amounts of indenting for each level.
Otherwise, good job!
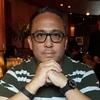
hapaman
Full Stack JavaScript Techdegree Student 3,026 PointsMuch appreciated. Thanks for taking a look.
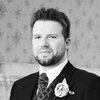
Joel Bardsley
31,248 PointsOne minor change you could make is replacing the hard-coded "3" in the correctAnsMsgEnd string with the qAndA.length value.
Once you reach the point where you've introduced ES2015 in your learning, you'll immediately see how you can make further improvements, such as using Template Strings for your correct answer message. Keep going at your own pace though and keep up the good work.
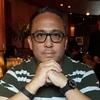
hapaman
Full Stack JavaScript Techdegree Student 3,026 PointsI actually had that in there for awhile, but was encountering some issues overall, and it got removed during my “fix”. I’ll put that back in there.
Much appreciate the feedback!