Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial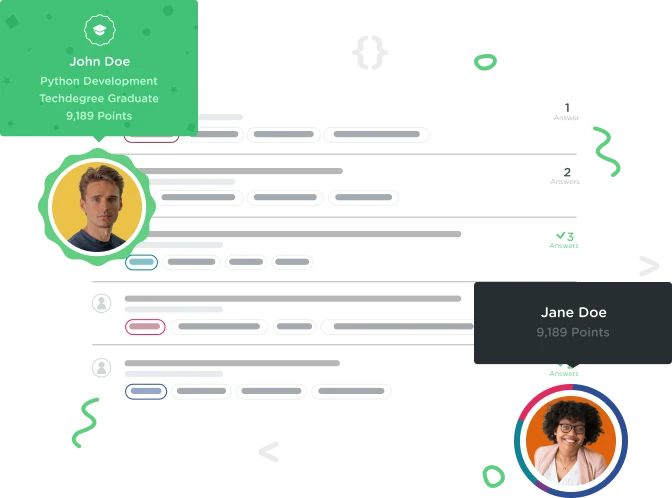

Bryan Quah
6,642 PointsMy code is able to carry out its purpose but does not work when I submit it for the code challenge.
I am able to create instances of the class to carry out the method. However, the code is not accepted as the answer for the challenge. Also, could someone explain how defining current_score works in this context and how it affects the rest of the code. It seems that there is no difference in the outcome when I define current_score in different ways.
class Game:
def __init__(self):
self.current_score = [0, 0]
def score(self,current_score = [0,0]):
playerArg = input("> Choose 1 or 2 ... ")
if playerArg == '1':
self.current_score[0] += 1
elif playerArg == '2':
self.current_score[1] += 1
else:
return self.score(current_score)
return self.current_score
8 Answers
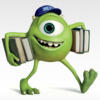
Tobias Edwards
14,458 PointsOkay, Good News! I found the challenge and quickly completed it! Without giving away the answer, I've tried to come up with a solution:
Firstly, self is used inside methods (functions inside classes) and you basically have to put this word self in front of any variable under a class - this shows that the variables are 'linked' to that class. For example, in our case, to show variables are 'linked' to the Game class, we have to put self before it. self doesn't have to be called self, it could be called anything, but its just a common word among programmers to show that variables and methods are 'linked' to their classes. If I have not explained this very well, which is probably the case, I would advise looking self up and doing some research. It took me about 20 mins to finally get the hang of it... lol
Anyway, back to the challenge: It says you need to create a score method (a function inside the Game class) which takes in a player argument. What you have done is not this unfortunately, instead you have taken in current_score and set its value to [0, 0]. Change this argument to a variable that represents a player argument e.g. player_arg.
Ok, to show this variable player_arg is linked to the Game class, you need to put self in front of it. To do this, you need to create a variable called self.player_arg and assign (=) it to player_arg:
self.player_arg = player_arg
Great! Now you have to throw in a couple of if-statements.... The task doesn't say it wants user input so you can take that bit of code out. The rest looks awesome!
The only other thing is the task doesn't want you to return a value, so you can take the two return statements out at the end. REMEMBER: Only do what the task says - cos' the tasks are like strict teachers (very picky). Instead, you can replace the return statement in you else statement with pass to make it syntactically correct.
WOW! And I think we're done!
If you have any other questions, make sure to respond to this post. Good luck and I hope you have a Merry Christmas!
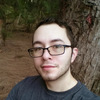
Unsubscribed User
11,389 PointsSome of these answers are too complex for the given situation. All they ask is to raise the score of the player given.
No input checking. No asking the compiler questions. Just an increase.
def score(self, player):
self.current_score[player - 1] += 1
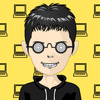
Erion Vlada
13,496 Pointsclass Game:
def __init__(self):
self.current_score = [0, 0]
def score(self, player):
self.player = player
if self.player == 1:
self.current_score[0] += 1
elif self.player == 2:
self.current_score[1] += 1
I got frustrated with this too, after realising how easy it was... Anyway, don’t make any modifications to the __init__
, its not necessary. All you need is an argument, player in my case and within the score method
you set self.player = player
and evaluate the user input (score argument
).
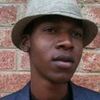
MUZ140734 Tinashe Chibwe
16,960 PointsTry this below. short and precise
class Game:
def init(self): self.current_score = [0, 0]
def score(self, player): self.current_score[1] += 1
return player
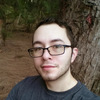
Unsubscribed User
11,389 PointsThis does not change the score for either player, just one as the player variable which is passed in is not referenced by the score function you wrote.
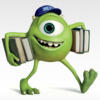
Tobias Edwards
14,458 PointsI don't know what code challenge this is for or what the task is. But I will give some general tips:
Make sure you name your variable/method/class names exactly how they state
Read the task question (what is it asking?)
Try alternative methods
Also, isn't playerArg suppose to be self.playerArg? I'm probably wrong but its just a thought.

Bryan Quah
6,642 PointsThis is the question for the code challenge:
Add a score method to Game that takes a player argument that'll be either 1 or 2. Increase that player's value in self.current_score by 1. You'll need to adjust the index (i.e. player = 1 means self.current_score[0] needs to increase).
I don't completely understand how the self argument works.

Alex Falconer-Athanassakos
2,635 Pointsclass Game:
current_score = [0,0]
def __init__(self, current_score):
self.current_score = score(current_score)
def score(self, current_score):
player_argument = input("Please pick 1 or 2: ")
if player_argument == 1:
current_score[0] += 1
elif player_argument == 2:
current_score[1] += 1
return current_score
To no avail
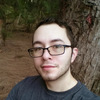
Unsubscribed User
11,389 PointsThe goal is to increase the player's score variable not return it. Because of this there is no reason to pass in current_score when i can be referenced by passing in self and using self.current_score
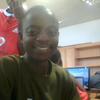
beven nyamande
9,575 Pointswhat about using a tuple,consider the following
player = (1,2)
#do something like player[0]
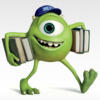
Tobias Edwards
14,458 PointsAlex Falconer-Athanassakos
Firstly, the challenge starts like this:
class Game:
def __init__(self):
self.current_score = [0, 0]
Remember to not change the start-off code unless the challenge explicitly states to do so. You need to build on top of that.
Secondly, read over my previous posts and compare your code with Bryan Quah's. This should help you pass the challenge and find out your mistakes.
Bryan Quah
6,642 PointsBryan Quah
6,642 PointsHey, thanks for the help! I managed to get the code working.
Here is the code I used:
The only question I have left is that - Why do we have to define 'player = 1' when we call the argument in the 'score' method when it is already defined in the 'init' method?
Tobias Edwards
14,458 PointsTobias Edwards
14,458 PointsThe code starts off like this:
And asks you to 'Add a score method to Game'. So there is no need in you code to set self.player to 1. The task wants you to take in an argument called player, so we should not set it to 1.
Similarly, in our score method, you do not need to set the player argument to 1. The whole point of this program is that players 1 or 2 can increment their score; in your program, only player 1 can increment their score because you set player to 1.
Lastly, although the syntax is fine, the task doesn't ask you to return anything, so there is no need for the return statement on the last line.
I hope this clears things up - we do not need to define player = 1 again because we shouldn't have in the first place.