Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial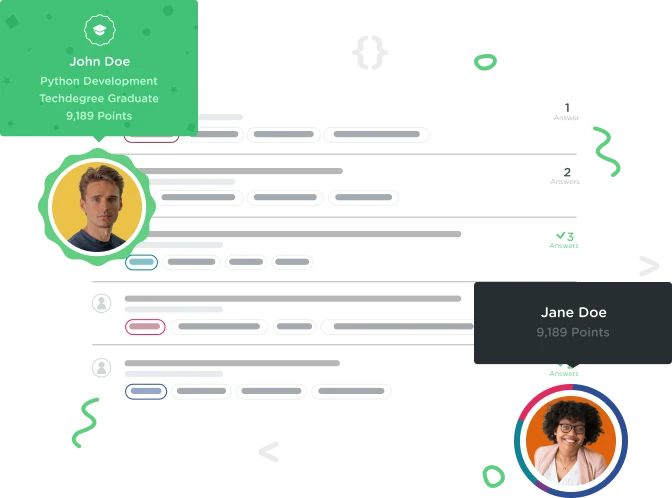

Iaroslav Moskviak
4,191 PointsMy code is not working even though it's the same as provided in the video
import os
shopping_list = []
def clear_screen():
os.system("cls" if os.name == "nt" else "clear")
def show_help():
clear_screen()
print("What should we pick up at the store?")
print("""
Enter 'DONE' to stop adding items.
Enter 'HELP' for this help.
Enter 'SHOW' to see your current list.
""")
def add_to_list(item):
show_list()
if len(shopping_list):
position = input("Where should I add {}?\n"
"Press ENTER to add to the end of the list\n"
"> ".format(item))
else:
position = 0
try:
position = abs(int(position))
except ValueError:
position = None
if position is not None:
shopping_list.insert(len(position-1, item))
else:
shopping_list.append(new_item)
show_list()
def show_list():
clear_screen()
print("Here's your list:")
index = 1
for item in shopping_list:
print("{}. {}".format(index, item)
index += 1
print("-"*10)
show_help()
while True:
new_item = input("> ")
if new_item.upper() == 'DONE' or new_item.upper() == "QUIT":
break
elif new_item.upper() == 'HELP':
show_help()
continue
elif new_item.upper() == 'SHOW':
show_list()
continue
else:
add_to_list(new_item)
show_list()
MOD: I formatted your code to make it easier to read. For how to do this, see rydavim's comment below on how to do this, or see the Markdown Cheatsheet below the textbox when you're typing the question. Thanks!
1 Answer
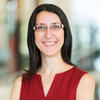
Louise St. Germain
19,424 PointsHello Iaroslav,
I can see three problems (there might be more):
-
You are missing a closing parenthesis on this line inside of show_list():
# You have this: (notice there are two opening parentheses and only one closing parenthesis) print("{}. {}".format(index, item) # Change it to: print("{}. {}".format(index, item))
Your whole file is indented with a mix of tabs and spaces. The code won't work properly until you make them either all tabs or all spaces (I recommend all spaces), so that's one thing you'll need to go through each line and make sure everything is done the same way. Right now the while loop is primarily spaces, and the rest of it is primarily tabs.
This line is causing some problems with Python (inside the add_to_list() function):
shopping_list.insert(len(position-1, item))
There are two issues with that len() function: one is that len() only takes one argument, and you gave it two things: position-1, and item. Second, even if you move one of the closing parenthesis over to the left, so that len only has one argument, like this:
shopping_list.insert(len(position-1), item)
there is still a problem because len() needs an iterable (string, list, etc), and an integer is not an iterable.
The code should work if you take out len() completely and just use the position instead:
shopping_list.insert(position-1, item)
After that, the only other thing that could be worth changing is to move the show_help() to just inside the while loop instead of being before it - that way people are reminded of their options every time they are prompted to enter something.
I hope this helps!
rydavim
18,814 Pointsrydavim
18,814 PointsThis will be difficult to troubleshoot without formatted code for folks to look at. Try taking a snapshot of your workspace, or using markdown to format the code in your post.
To share your workspace, first take a snapshot by clicking the camera icon in the top right of the workspace. This allows you to share your workspace so that others can open a copy of it. Don't worry, they won't be able to change the code on your end.
Alternatively, you can use markdown to format your code in a post such that it's easier to read and copy-paste accurately. To format a code block, simply wrap it in three back-ticks (```). You can optionally add a language for fancy syntax highlighting - make sure you don't have a space after the back-ticks (```python).
Example:
shopping_list = []