Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial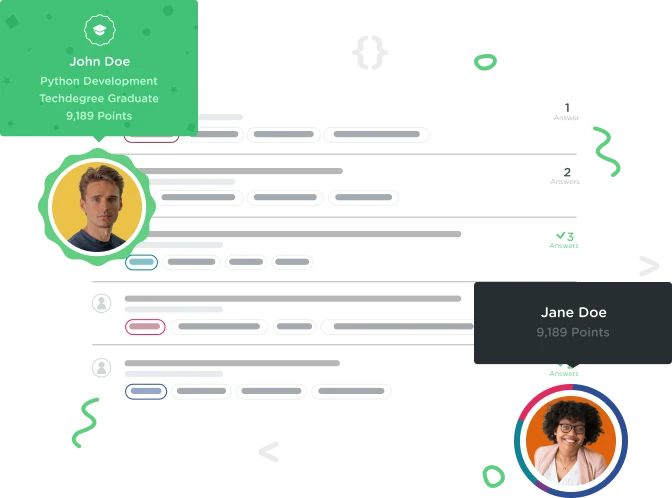
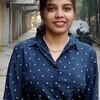
Yogita Verma
5,549 PointsMy code is not working I can't figure out the problem
index.html
<!DOCTYPE html>
<html>
<head>
<title>JavaScript and the DOM</title>
<link rel="stylesheet" href="css/style.css">
</head>
<body>
<h1 id="myHeading">JavaScript and the DOM</h1>
<p>Making a web page interactive</p>
<p class="li-para">Things that are purple:</p>
<input type="text" id="myText">
<ul>
<li>grapes</li>
<li>amethyst</li>
<li>lavender</li>
<li>plums</li>
</ul>
<button type="button" id="bu">Click Me To change para!</button>
<script src="app.js"></script>
</body>
</html>
app.js
const head=document.querySelectorAll('h1');
const bu=document.querySelectorAll('#bu');
const inp=document.querySelectorAll('#myText');
const p=document.querySelectorAll('.li-para');
const t=()=>{
p.innerHTML=inp.value;
}
bu.addEventListener('click',t);
2 Answers
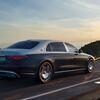
Balazs Peak
46,160 PointsFirst: you can not use "querySelectorAll()" for ids since the id indicates that there is only one of that element. You can use "querySelectorAll()" for classes and tags, and if there is only one of them, you will have a list of one element. But you can not do that with ids, you have to use the "querySelector()" for ids. (The not plural version.) Examples with both the plural version and the singular version:
const head=document.querySelectorAll('h1');
const bu=document.querySelector('#bu');
Second: you can not access the innerHTML property of a list. Only the elements of the list has innerHTML property, not the list itself. You have to iterate through the list to access it's elements and innerHTML properties thereof. Example:
const t=()=>{
for (let i = 0; i<p.length; i++)
{
p[i].innerHTML = inp.value;
}
}
bu.addEventListener('click',t);
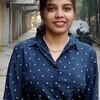
Yogita Verma
5,549 PointsThis does not seem to work .I only want the para with class li-para to change when clicking the button accoring to the input value
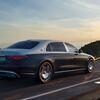
Balazs Peak
46,160 PointsI don't know how you expect this program to work. You'll have to figure out which elements do you want to change and access the corresponding css selectors. But in general, what I've commented about the logic of the DOM traversing is correct.
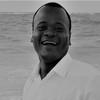
Jimmy Dorvilus
Full Stack JavaScript Techdegree Graduate 27,972 PointsI think you should keep it simple. The following JS code works fine for me.
const p=document.querySelector('.li-para');
const inp= document.querySelector('#mytext');
const bu= document.querySelector('#bu');
bu.addEventListener('click', ()=>{
p.textContent=inp.value;
});
Yogita Verma
5,549 PointsYogita Verma
5,549 PointsIts giving me this error on console '' bu.addEventListener is not a function"