Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial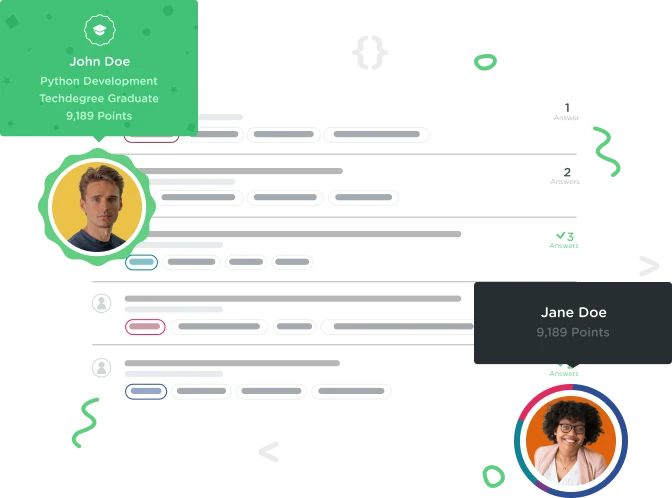

Robert Young
11,579 PointsMy code is very verbose but it works - could not remember EVERYTHING - any tips?
I tried to do this all off the top of my head - the function just would not work and as a result it became overly verbose - I feel I am improving but this is all I could do for this - anyone got any tips?
var html;
var stud1 = [];
var stud2 = [];
var stud3 = [];
var stud4 = [];
var stud5 = [];
var name;
var track;
var achievements;
var points;
function print(message) {
var div = document.getElementById('output');
div.innerHTML = message;
}
var students = [
{ name: 'Dave', track: 'Front End Development', achievements: 158, points: 14730 },
{ name: 'Jody', track: 'iOS Development with Swift', achievements: '175', points: '16375' },
{ name: 'Jordan', track: 'PHP Development', achievements: '55', points: '2025' },
{ name: 'John', track: 'Learn WordPress', achievements: '40', points: '1950' },
{ name: 'Trish', track: 'Rails Development', achievements: '5', points: '350' }
];
for (var i = 0; i < students.length; i += 1) {
name = students[i].name;
if (name === 'Dave'){
stud1.push(students[0].name, students[0].track, students[0].achievements, students[0].points);
console.log(stud1);
} else if (name === 'Jody'){
stud2.push(students[1].name, students[1].track, students[1].achievements, students[1].points);
console.log(stud2);
} else if (name === 'Jody'){
stud3.push(students[2].name, students[2].track, students[2].achievements, students[2].points);
console.log(stud3);
} else if (name === 'John'){
stud4.push(students[3].name, students[3].track, students[3].achievements, students[3].points);
console.log(stud4);
} else if (name === 'Trish'){
stud5.push(students[4].name, students[4].track, students[4].achievements, students[4].points);
console.log(stud5);
}
}
html = '<h2>Student: ' + stud1[0] + '</h2>'
html += '<p>Track: ' + stud1[1] + '</p><p>Points: ' + stud1[2] + '</p><p>Achievements: ' + stud1[3] + '</p>';
html += '<h2>Student: ' + stud2[0] + '</h2>'
html += '<p>Track: ' + stud2[1] + '</p><p>Points: ' + stud2[2] + '</p><p>Achievements: ' + stud2[3] + '</p>';
html += '<h2>Student: ' + stud3[0] + '</h2>'
html += '<p>Track: ' + stud3[1] + '</p><p>Points: ' + stud3[2] + '</p><p>Achievements: ' + stud3[3] + '</p>';
html += '<h2>Student: ' + stud4[0] + '</h2>'
html += '<p>Track: ' + stud4[1] + '</p><p>Points: ' + stud4[2] + '</p><p>Achievements: ' + stud4[3] + '</p>';
html += '<h2>Student: ' + stud5[0] + '</h2>'
html += '<p>Track: ' + stud5[1] + '</p><p>Points: ' + stud5[2] + '</p><p>Achievements: ' + stud5[3] + '</p>';
print(html);
3 Answers
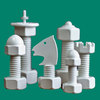
Steven Parker
231,268 PointsYou can make your program more "DRY" (concise) by using the loop to perform the repetitive tasks instead of creating separate arrays and then coding them individually for each student.
For example, except for the "print" function and the "students" array, this code can be condensed like this:
var html = "";
for (var i = 0; i < students.length; i += 1) {
var stud = students[i];
html += "<h2>Student: " + stud.name + "</h2>";
html += "<p>Track: " + stud.track + "</p><p>Points: " + stud.points;
html += "</p><p>Achievements: " + stud.achievements + "</p>";
}
print(html);
This also eliminates the "studN" arrays. The variables with the same names as the properties were already unused.

Robert Young
11,579 Points@stevenparker hey - thank you so much - I will look at this soon I promise - I am currently purging my workspace and making everything in to a repo so it's easier for me to manage and search my own code :)
Really appreciate your help :)

Robert Young
11,579 PointsSteven Parker Noted and done - thank you for pointing that out also :)
Robert Young
11,579 PointsRobert Young
11,579 PointsHey @stevenparker - thank you - that is what I was trying but it was getting the data from the arrays that stumped me - I think I need a break and will look again later this week once I've learned a bit more (hopefully)