Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial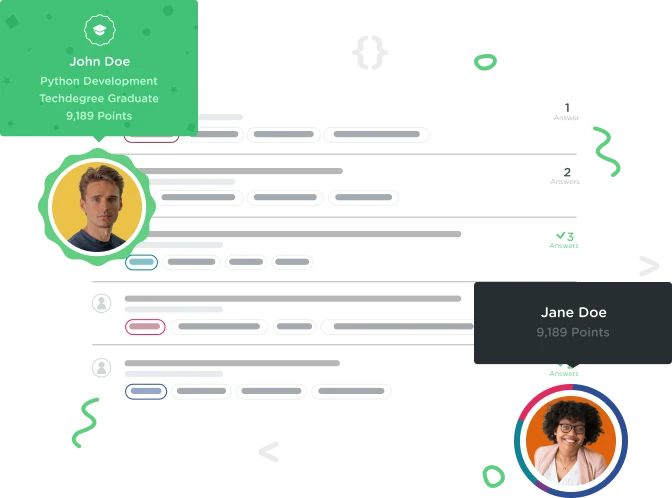

R R
1,570 PointsMy code is working but it's not very random
Would love some feedback on this before I watch the solution video. I'm sure this is wrong-specifically the second one. How do I get more random results? And I'm totally stumped on the the "taking it further" challenge at the end of the video.
Code for first challenge:
var userNum = prompt("Hey you! Type in a number. Any number");
var num = parseInt(userNum);
var result = parseInt(Math.random() * userNum ) + 1;
document.write(result);
Taking it further challenge:
var userNumA = prompt("Hey you! Type in a number. Any number");
var userNumB = prompt("OK, type in a different number.");
//var num = parseInt(userNum);
//var result = parseInt(Math.random() * userNum ) + 1;
//document.write(result);
var valueA = parseInt(Math.random() * userNumA) +1;
var valueB = parseInt(Math.random() * userNumB) +1;
console.log(valueA);
console.log(valueB);
var valueC = (valueB - valueA);
document.write(valueC);
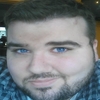
Marcus Parsons
15,719 PointsHey Justin,
The problem with the 2nd part of the code is that it will not always will return a value that's between the two sets of numbers given. For example, let's say that userNumA is 50 and userNumB is 100. valueA would generate a value from 0-50 and valueB would generate a number from 0-100. Looking at the combinations of those numbers (and the maximum output of Math.random() which is 0.9999999...), the range goes from 1-100, not from 50-100, which is what we want. The random number should always fall into the range given by the user's input, which is why we use a variation of the solution I provided in the answer. Does that make sense?
4 Answers
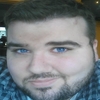
Marcus Parsons
15,719 PointsHey R R,
You can condense that entire 2nd challenge down into just 5 lines.
var userNumA = prompt("Hey you! Type in a number. Any number");
var userNumB = prompt("OK, type in a different number.");
userNumA = parseInt(userNumA);
userNumB = parseInt(userNumB);
document.write(Math.floor(Math.random() * (userNumB - userNumA + 1)) + userNumA);
You can even do some error checking to make sure the user didn't accidentally put in something that wasn't a number and have to keep refreshing the page to rerun the function:
//Initialize the two inputs
var userNumA, userNumB;
//This loop will run until both inputs are numbers only
do {
userNumA = prompt("Hey you! Type in a number. Any number");
userNumB = prompt("OK, type in a different number.");
} while (isNaN(userNumA) || isNaN(userNumB))
userNumA = parseInt(userNumA);
userNumB = parseInt(userNumB);
document.write(Math.floor(Math.random() * (userNumB - userNumA + 1)) + userNumA);

R R
1,570 PointsThank you Justin and Marcus. I didn't think about doing this:
(Math.floor(Math.random()...
Very nice tip. Thank you!
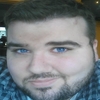
Marcus Parsons
15,719 PointsAnytime, R R! If you have any more questions, we are here to help. Happy coding! :)
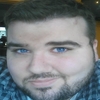
Marcus Parsons
15,719 PointsOh, and check out my comment to Justin for the reasoning behind why your 2nd set of code should use the same math algorithm that my answer uses.

R R
1,570 PointsMarcus, I also didn't think about redefining a variable later in the code. In your sample, userNumA is, at first, whatever the user plugs in. Then you turn userNumA into an integer but keep the same variable name. So now, userNumA is the integer version of the user's input. Am I understanding this correctly? I created a new variable when I used parseInt. So, thats not necessary? is there a best practice? I do like how this makes the code shorter.
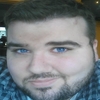
Marcus Parsons
15,719 PointsIt not only makes the code shorter but more importantly it makes it right. I hope you read my comment thoroughly and understand why the code you have doesn't work. It doesn't matter that I said userNumA = parseInt(userNumA);
or if I made it valueA. Either way, you are parsing the input into an integer. You still can't apply the randomness the way you did and have it fall within a range. It can't be done separately and then stitched together because of the way the random() function works. It must be done the way I did in my answer, and again, my comment below Justin's comment explains why that is so.
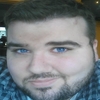
Marcus Parsons
15,719 PointsI should go into more detail about why you have to do it all of that math in one line instead of separating it.
We are looking at that last line that is written to the page via document.write():
Math.floor(Math.random() * (userNumB - userNumA + 1)) + userNumA
That line Is responsible for setting the value that falls within the specified range. Also notice that Math.floor() does not include the last addition of userNumA. Let's look at the maximum and minimum possible output for this line of code. Math.random() can only ever get to 0.999999... because it has a range from [0,1) in interval notation (0 is inclusive, 1 is exclusive). So, if we say we got the maximum random value of 0.999999... and we multiply that by the user's input (let's say B is 100 and A is 50), we get "0.999999.. * (100-50+1)" which equals "0.99999... * (51)" which equals approximately "50.999999..." and then we floor that value we get 50 because all floor does is take away any decimals attached to the integer basically (or rounds down regardless of decimal value). Then, the program would add the 50 gathered from the floor operation and add it to the 50 stored in userNumA. Thus, the maximum possible value is 100.
The minimum possible value for this command would come from Math.random() being 0. So if we say "0 * (100-50+1)" it is 0 because anything times zero is zero, right? So, then we add the 50 stored in userNumA and we get the minimum possible value is 50.
If you apply the same logic of Math.random() to your code, you'll see exactly what I'm talking about when I say the range of your code is [0, Maximum # input] (regardless of input values) whereas that code I posted has a range of [Minimum # input, Maximum # input] (regardless of input values).
If you want me to go into detail on your code, I will do so.

R R
1,570 PointsThank you so much, Marcus. I need to dig into your response for a bit to fully digest it. I'll get this eventually. :-)
Justin Swatloski
5,769 PointsJustin Swatloski
5,769 PointsAs far as I can tell, your first code is plenty random. Here's the results of me running the following code:
I entered 15 for the value, my results: 8, 5, 13, 15, 3, 4, 2, 4, 8, 15.
I didn't evaluate the second one, because I didn't see where you were having an issue. :x