Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial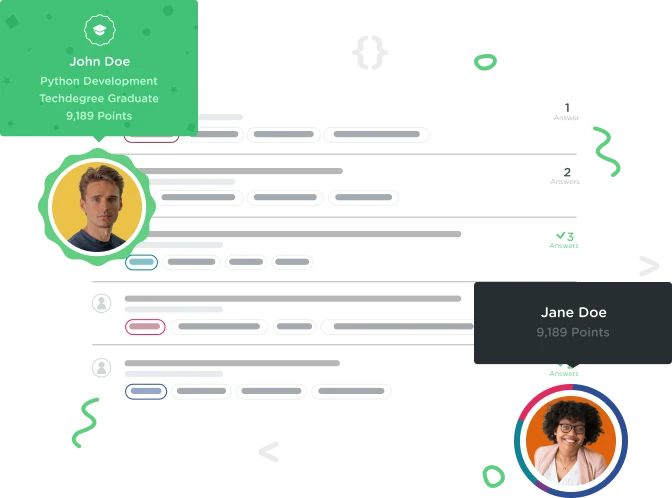
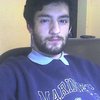
Dario Bahena
10,697 PointsMy code keeps varying between answers.
# The dictionary will be something like:
# {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Often, it's a good idea to hold onto a max_count variable.
# Update it when you find a teacher with more classes than
# the current count. Better hold onto the teacher name somewhere
# too!
#
# Your code goes below here.
teachers = {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
'Kenneth Love': ['Python Basics', 'Python Collections']}
teacher_list = [] #creats a list of teacher names
teacher_value_list = [] #vlues of the teacher names
teacher_dict = {} #contains values with amount per key
final_dict = {}
count = 0
for i in teachers:
teacher_list.append(i) #spits teacher names to teach_list
teacher_value_list.append(i)
for i in teachers:
#teacher_dict.update({i:len(i)})
teacher_dict.update({i:len(teachers[i])})
print(teacher_dict)
#print(teacher_list)
#print(count)
for i in teacher_list:
if len(teachers[i]) > count:
count = count + len(teachers[i])
final_dict.update({i:teachers[i]})
if len(teachers[i]) < count:
count = count - len(teachers[i])
try:
del final_dict[i]
except:
pass
print(count)
print(final_dict)
#I know that it is not in a function. I wrote this just to test
#out the main routine. I don't understand why it is giving #me different results each time.
[edit formatting --cf]
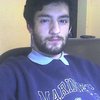
Dario Bahena
10,697 PointsIn the first if statement, I test the value of the teacher i in the dictionary to the count variable. since I have the count start at 0 the count will go up by the length of the values in i teacher. After that, the final_dict dictionary will update to the teacher and length of the value that the initial loop performed on. the second if tests if the value is less then count. since count went up already it should return as false and loop again if it $sics', 'Python Collections']} prints.
2 Answers
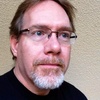
Chris Freeman
Treehouse Moderator 68,423 PointsThe main issue is how your tracking the count
. The key is to define precisely what count
represents. If it is to be the "current maximum value found so far", then the assignment to count is in error:
# instead of
count = count + len(teachers[i])
# use
count = len(teachers[i])
And drop the "count = count - len(teachers[i])" from the second if statement.
The next issue is how you're tracking the teacher with the maximum count. Instead of trying to use a dictionary update and delete to alter the "finial" teacher, you can simple overwrite final_dict
when needed:
# change first if to use:
final_dict = {i: teachers[i])
# remove try/except from second if statement
After both of these changes, you'll notice the second if statement is no longer needed.
Finally, you don't need to reconstruct the dictionaries throughout your code.
# the for loop:
for i in teachers:
print(i)
# and the teacher_list you've created:
for i in teacher_list:
print(i)
# do the SAME thing.
teacher_list
is not needed, use the loop directly on teachers
which is actually the loop over the keys of the teachers
dictionary.
Also, you don't have to track the "final" teacher using a dictionary. You could simply track the teacher name then recall that teachers data when needed:
for i in teachers:
if len(teachers[i]) > count:
count = len(teachers[i])
max_teacher = i
print(count)
print({max_teacher: teachers[max_teacher]})
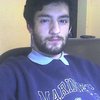
Dario Bahena
10,697 PointsThanks a bunch! This had me stuck for a while.
Chris Freeman
Treehouse Moderator 68,423 PointsChris Freeman
Treehouse Moderator 68,423 PointsCan you explain more about what values are varying and what are the values?