Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial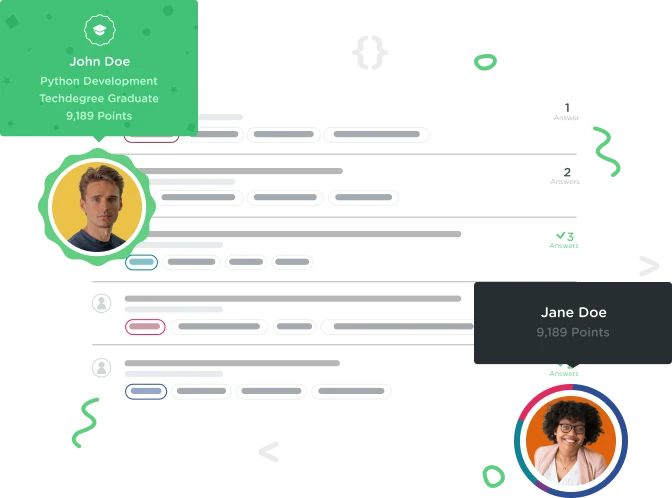

Susan Rusie
10,671 PointsMy code looks like it is right and all my dependencies are installed, but why am I getting this error?
I got Mongo running and started to run "node app.js" to Test the Routes and it isn't working because I am getting this error. What is causing this error? I have installed all the dependencies as instructed. Why is that? Here is my code:
Rusie@DESKTOP-05UMA67 MINGW64 ~/Documents/susan_treehouse/Building_a_Rest_API/Test_Routes_With_Postman (master)
$ node app.js
module.js:471
throw err;
^
Error: Cannot find module 'mongooose'
at Function.Module._resolveFilename (module.js:469:15)
at Function.Module._load (module.js:417:25)
at Module.require (module.js:497:17)
at require (internal/module.js:20:19)
at Object.<anonymous> (C:\Users\Rusie\Documents\susan_treehouse\Building_a_R
est_API\Test_Routes_With_Postman\app.js:13:16)
at Module._compile (module.js:570:32)
at Object.Module._extensions..js (module.js:579:10)
at Module.load (module.js:487:32)
at tryModuleLoad (module.js:446:12)
at Function.Module._load (module.js:438:3)
at Module.runMain (module.js:604:10)
at run (bootstrap_node.js:394:7)
at startup (bootstrap_node.js:149:9)
at bootstrap_node.js:509:3
app.js
'use strict';
var express = require("express");
var app = express();
var routes = require("./routes");
var jsonParser = require("body-parser").json;
var logger = require("morgan");
app.use(logger("dev"));
app.use(jsonParser());
var mongoose = require("mongooose");
mongoose.connect("mongodb://localhost:27017/qa");
var db = mongoose.connection;
db.on("error", function(err){
console.error("connection error:", err);
});
db.once("open", function(){
console.log("db connection successful");
});
app.use("/questions", routes);
// catch 404 and forward to error handleer
app.use(function(req, res, next){
var err = new Error("Not Found");
err.status = 404;
next(err);
});
// Error Handler
app.use(function(err, req, res, next){
res.status(err.status || 500);
res.json({
error: {
message: err.message
}
});
});
var port = process.env.PORT || 3000;
app.listen(port, function(){
console.log("Express server is listening on port", port);
});
models.js
'use strict';
var mongoose = require("mongoose");
var Schema = mongoose.Schema;
var sortAnswers = function(a, b) {
//- negative a before b
//0 no change
//+ positive a after back
if(a.votes === b.votes){
return b.updatedAt - a.updatedAt;
}
return b.votes - a.votes;
}
var AnswerSchema = new Schema({
text: String,
createdAt: {type: Date, default: Date.now},
updatedAt: {type: Date, default: Date.now},
votes: {type: Number, default:0}
});
AnswerSchema.method("update", function(updates, callback) {
Object.assign(this, updates, {updatedAt: new Date()});
this.parent().save(callback);
});
AnswerSchema.method("vote", function(vote, callback) {
if(vote === "up") {
this.votes += 1;
} else {
this.votes -= 1;
}
this.parent().save(callback);
});
var QuestionSchema = new Schema({
text: String,
createdAt: {type: Date, default: Date.now},
answers: [AnswerSchema]
});
QuestionSchema.pre("save", function(next){
this.answers.sort(sortAnswers);
next();
});
var Question = mongoose.model("Question", QuestionSchema);
module.exports.Question = Question;
mongoose_sandbox.js
'use strict';
var mongoose = require("mongoose");
mongoose.connect("mongodb://localhost:27017/sandbox");
var db = mongoose.connection;
db.on("error", function(err){
console.log("connection error:", err);
});
db.once("open", function(){
console.log("db connection successful");
// All database communication goes here
var Schema = mongoose.Schema;
var AnimalSchema = new Schema({
type: {type: String, default: "goldfish"},
size: String,
color: {type: String, default: "golden"},
mass: {type: Number, default: 0.007},
name: {type: String, default: "Angela"}
});
AnimalSchema.pre("save", function(next){
if(this.mass >= 100) {
this.size = "big";
} else if (this.mass >= 5 && this.mass < 100) {
this.size = "medium";
} else {
this.size = "small";
}
next();
});
AnimalSchema.statics.findSize = function(size, callback){
//this == Animal
return this.find({size: size}, callback);
}
AnimalSchema.methods.findSameColor = function(callback) {
//this == document
return this.model("Animal").find({color: this.color}, callback);
}
var Animal = mongoose.model("Animal", AnimalSchema);
var elephant = new Animal({
type: "elephant",
color: "gray",
mass: 6000,
name: "Lawrence"
});
var animal = new Animal({}); //Goldfish
var whale = new Animal({
type: "whale",
mass: 190500,
name: "Fig"
});
var animalData = [
{
type: "mouse",
color: "gray",
mass: 0.035,
name: "Marvin"
},
{
type: "nutria",
color: "brown",
mass: 6.35,
name: "Gretchen"
},
{
type: "wolf",
color: "gray",
mass: 45,
name: "Iris"
},
elephant,
animal,
whale
];
Animal.remove({}, function(err) {
if (err) console.error(err);
Animal.create(animalData, function(err, animals){
if (err) console.error(err);
Animal.findOne({type:"elephant"}, function(err, elephant) {
elephant.findSameColor(function(err, animals){
if (err) console.error(err);
animals.forEach(function(animal){
console.log(animal.name + " the " + animal.color + " " + animal.type + " is a " + animal.size + "-sized animal.");
});
db.close(function(){
console.log("db connection closed");
});
});
});
});
});
});
routes.js
'use strict';
var express = require("express");
var router = express.Router();
var Question = require("./models").Question;
router.param("qID", function(req,res,next,id){
Question.findById(id, function(err, doc){
if(err) return next(err);
if(!doc) {
err = new Error("Not Found");
err.status = 404;
return next(err);
}
req.question = doc;
return next();
});
});
router.param("aID", function(req,res,next,id){
req.answer = req.question.answers.id(id);
if(!req.answer) {
err = new Error("Not Found");
err.status = 404;
return next(err);
}
next();
});
// GET /questions
// Route for questions collection
router.get("/", function(req, res, next){
Question.find({})
.sort({createdAt: -1})
.exec(function(err, questions){
if(err) return next(err);
res.json(questions);
});
});
// POST /questions
// Route for creating questions
router.post("/", function(req, res, next){
var question = new Question(req.body);
question.save(function(err, question){
if(err) return next(err);
res.status(201);
res.json(questions);
});
});
// GET /questions/:id
// Route for specific questions
router.get("/:qID", function(req, res, next){
res.json(req.question);
});
// POST /questions
// Route for creating an answer
router.post("/:qID/answers", function(req, res, next){
req.question.answers.push(req.body);
req.question.save(function(err, question){
if(err) return next(err);
res.status(201);
res.json(question);
});
});
// PUT /questions/:qID/answers/:aID
// Edit a specific answer
router.put("/:qID/answers/:aID", function(req, res){
req.answer.update(req.body, function(err, result){
if(err) return next(err);
res.json(result);
});
});
// DELETE ?questions/:qID/answers/:aID
// Delete a specific answer
router.delete("/:qID/answers/:aID", function(req, res){
req.answer.remove(function(err, question){
req.question.save(function(err, question){
if(err) return next(err);
res.json(question);
});
});
});
// POST/questions/:qID/answers/:aID/vote-up
// POST/questions/:qID/answers/:aID/vodte-down
// Vote on a specific answer
router.post("/:qID/answers/:aID/vote-:dir",
function(req, res, next){
if(req.params.dir.search(/^(up|down)$/) === -1) {
var err = new Error("Not Found");
err.status = 404;
next(err);
} else {
req.vote = req.params.dir;
next();
}
},
function(req, res, next){
req.answer.vote(req.vote, function(err, question){
if(err) return next(err);
res.json(question);
});
});
module.exports = router;
package.json
{
"name": "Create_An_Express_App",
"version": "1.0.0",
"main": "app.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"keywords": [],
"author": "",
"license": "ISC",
"dependencies": {
"body-parser": "^1.15.2",
"express": "^4.13.4",
"mongoose": "^4.4.20",
"morgan": "^1.7.0"
},
"description": ""
}
2 Answers

Stephan Olsen
6,650 PointsIn your app.js you're requiring mongoose with an 'o' too much.
var mongoose = require("mongooose");
// Should be
var mongoose = require("mongoose");

Ivan Kuznetsov
Courses Plus Student 733 PointsJESUS CHRIST!!!
Susan Rusie
10,671 PointsSusan Rusie
10,671 PointsThank you. That fixed the problem.