Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial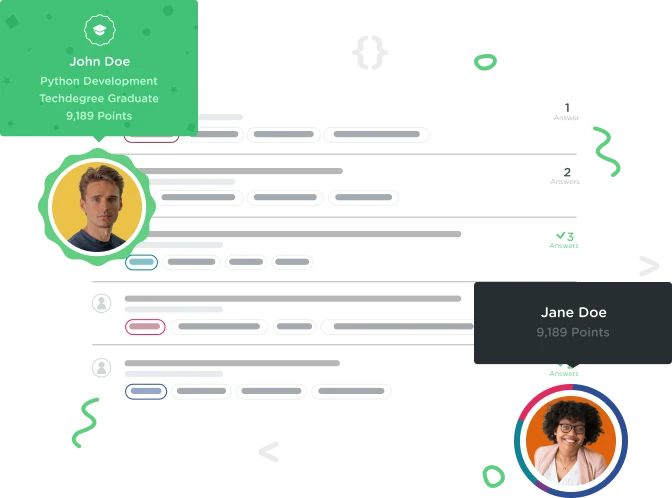

Varun Biswas
Courses Plus Student 516 PointsMy code returns 2 Chomps after the 12 Chomps and before the try-catch block, however we both fed 6. Can't find the bug.
PezDispenser.java
public class PezDispenser {
public static final int MAX_PEZ = 12;
private String mCharacterName;
private int mPezCount;
public PezDispenser(String characterName) {
mCharacterName = characterName;
mPezCount = 0;
}
public boolean dispense() {
boolean wasDispensed = false;
if(!isEmpty()) {
mPezCount--;
wasDispensed = true;
}
return wasDispensed;
}
public boolean isEmpty() {
return mPezCount == 0;
}
public void pezLoad() {
pezLoad(MAX_PEZ);
}
public void pezLoad(int pezAmount) {
mPezCount += pezAmount;
if (mPezCount > MAX_PEZ) {
throw new IllegalArgumentException("Too many PEZ!!!");
}
mPezCount = pezAmount;
}
public String getCharacterName() {
return mCharacterName;
}
}
Example.java
public class Example {
public static void main(String[] args) {
// Your amazing code goes here...
System.out.println("We're making a new Pez Dispenser.");
PezDispenser dispenser = new PezDispenser("Yoda");
System.out.printf("The Dispenser character is %s.\n", dispenser.getCharacterName());
if (dispenser.isEmpty()) {
System.out.println("The Dispenser is Currently Empty.");
}
System.out.println("The Dispenser is now being loaded.\nLoading . . . ");
dispenser.pezLoad();
if (!dispenser.isEmpty()) {
System.out.println("The Dispenser is no longer empty!");
}
while(dispenser.dispense()) {
System.out.println("Chomp!");
}
if(dispenser.isEmpty()) {
System.out.println("Ate all the PEZ!");
}
dispenser.pezLoad(4);
dispenser.pezLoad(2);
while(dispenser.dispense()) {
System.out.println("Chomp!");
}
try {
dispenser.pezLoad(200);
System.out.println("This will never happen!");
} catch(IllegalArgumentException iae) {
System.out.println("Ouch!!");
System.out.printf("%s\n", iae.getMessage());
}
}
}
java Example Ouput:
treehouse:~/workspace$ java Example
We're making a new Pez Dispenser.
The Dispenser character is Yoda.
The Dispenser is Currently Empty.
The Dispenser is now being loaded.
Loading . . .
The Dispenser is no longer empty!
Chomp!
Chomp!
Chomp!
Chomp!
Chomp!
Chomp!
Chomp!
Chomp!
Chomp!
Chomp!
Chomp!
Chomp!
Ate all the PEZ!
Chomp!
Chomp!
Ouch!!
Too many PEZ!!!
2 Answers

Craig Dennis
Treehouse TeacherYour code with comments...
public void pezLoad(int pezAmount) {
// Add pezAmount to the total and set the total to be the result
mPezCount += pezAmount;
// Then check...hmm...this probably be checked first using a separate variable
if (mPezCount > MAX_PEZ) {
throw new IllegalArgumentException("Too many PEZ!!!");
}
// Finally set the total to whatever was passed in.
// Whoops! so when you pass in 2, you set the total to 2
mPezCount = pezAmount;
}
That help?
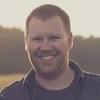
Jonny Patterson
7,099 PointsHi Varun
I'm fairly new to this, having just completed the exercise, but I think the issue may be in your pezLoad() method. I tried your code and was able to make it work by doing this:
public void pezLoad(int pezAmount) {
int newPezCount = mPezCount + pezAmount;
if (mPezCount > MAX_PEZ) {
throw new IllegalArgumentException("Too many PEZ!!!");
}
mPezCount = newPezCount;
}
Unfortunately, as a newbie, I'm not quite sure how to explain why your current code doesn't work. I suspect the issue is the final line in your pezLoad() method:
mPezCount = pezAmount;
As far as I can tell this means that no matter what happens within the method, the last thing it does is to set mPezCount to whatever you entered as pezAmount. In your example the last pezAmount entered is 2, which is why you are returning two 'chomps' .
I hope this makes sense, and isn't misleading! Perhaps someone more experienced can verify!
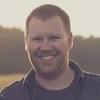
Jonny Patterson
7,099 PointsActually, come to think of it, if you just comment out the last line of your pezLoad() method it should work, as pezAmount won't be replacing mPezCount. Appears to work correctly:
public void pezLoad(int pezAmount) {
mPezCount += pezAmount;
if (mPezCount > MAX_PEZ) {
throw new IllegalArgumentException("Too many PEZ!!!");
}
//mPezCount = pezAmount;
}
Varun Biswas
Courses Plus Student 516 PointsVarun Biswas
Courses Plus Student 516 PointsSo, why was this added in the first place. Should this come with an else {}
Craig Dennis
Treehouse TeacherCraig Dennis
Treehouse TeacherI think you should make a variable to store the result, and if the result is too large throw the exception. Then if it passes the exception check, set the total to the result.
Make sense?
Varun Biswas
Courses Plus Student 516 PointsVarun Biswas
Courses Plus Student 516 PointsAbsolutely, Basics are fun. I'm touching Java after 8 years and i feel like a kid again. Here is what I did.
Here is the output. Ate all the PEZ!
Pez Count = 0.
Pez Count = 4.
Pez Count = 6.
Chomp!
Chomp!
Chomp!
Chomp!
Chomp!
Chomp!
Ouch!!
Too many PEZ!!!
Pez Count = 0.