Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial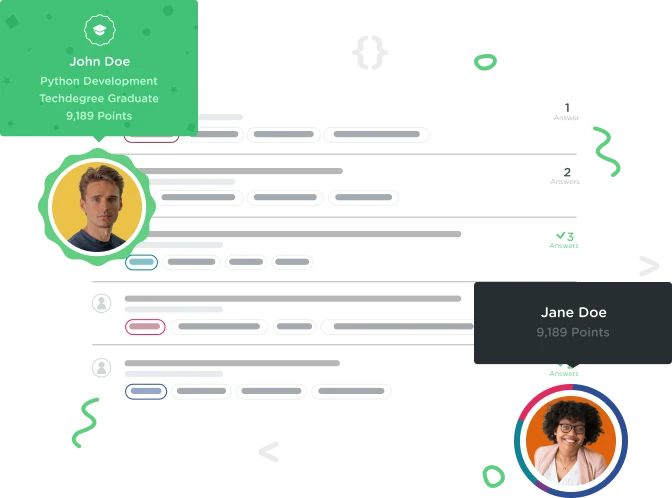

konstantinslastnikov
3,094 PointsMy code returns "Bummer! Try again!", while the same code works fine on Workspces
class Hand(list): def init(self, dices=None, *args, **kwargs): super().init() if not isinstance(dices, list): raise ValueError("You must provide a list of Die class objects") for item in dices: if not isinstance(item, Die): raise ValueError("The {} is not Die class objects".format(item)) for item in dices: self.append(item)
@property
def total(self):
return sum(self)
@classmethod
def roll(cls, size = 0):
dices = []
for _ in range(size):
dices.append(D20())
return cls(dices)
import random
class Die:
def __init__(self, sides=2):
if sides < 2:
raise ValueError("Can't have fewer than two sides")
self.sides = sides
self.value = random.randint(1, sides)
def __int__(self):
return self.value
def __add__(self, other):
return int(self) + other
def __radd__(self, other):
return self + other
class D20(Die):
def __init__(self):
super().__init__(sides = 20)
class Hand(list):
def __init__(self, dices=None, *args, **kwargs):
super().__init__()
if not isinstance(dices, list):
raise ValueError("You must provide a list of Die class objects")
for item in dices:
if not isinstance(item, Die):
raise ValueError("The {} is not Die class objects".format(item))
for item in dices:
self.append(item)
@property
def total(self):
return sum(self)
@classmethod
def roll(cls, size = 0):
dices = []
for _ in range(size):
dices.append(D20())
return cls(dices)
2 Answers
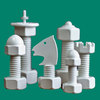
Steven Parker
229,744 PointsI notice that you have created an "__init__
" override for the "Hand" class that makes an argument necessary to create an instance. The challenge is expecting to be able to create an instance without an argument, just as you could in the original code. Adding a required argument was not part of the instructions.
And based on what the instructions do ask for, this override can be omitted entirely. Your new "roll" method has everything needed to work. Good job on that part.
Otherwise, the only other issue is that you'll need "from dice import D20
" to allow your new "roll" method to use it.

konstantinslastnikov
3,094 PointsI found the issue. I had to import D20 for dice.py
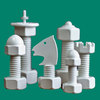
Steven Parker
229,744 PointsThat, and get rid of the "__init__
", right?
konstantinslastnikov
3,094 Pointskonstantinslastnikov
3,094 PointsYes, you're right, code perfectly works without overriding an init I just try to understand why it fails Anyway, thanks!