Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial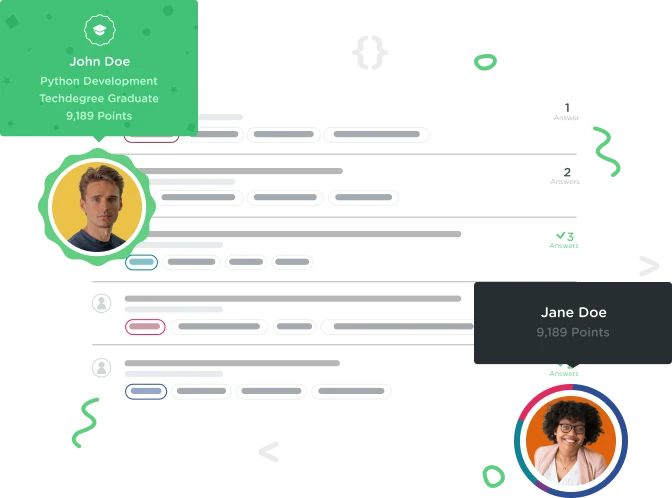

Dalton Coble
6,489 PointsMy code wont run do to an error. please help(Solved)
my code:
import java.util.Arrays;
import java.util.Date;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
import java.util.TreeSet;
import com.teamtreehouse.Treet;
import com.teamtreehouse.Treets;
public class Example {
public static void main(String[] args) {
Treet[] treets = Treets.load();
System.out.printf("There are %d treets. %n",
treets.length);
Set<String> allHashTags = new HashSet<String>();
Set<String> allMentions = new TreeSet<String>();
for (Treet treet : treets) {
allHashTags.addAll(treet.getHashTags());
allMentions.addAll(treet.getMentions());
}
System.out.printf("Hash tags: %s %n", allHashTags);
System.out.printf("Mentions: %s %n", allMentions);
}
Map<String, Integer> hashTagCounts = new HashMap<String, Integer>();
for (Treet treet : treets) {
for (String hashTag : treet.getHashTags()) {
Integer count = hashTagCounts.get(hashTag);
if (count == null) {
count = 0;
count ++;
hashTagCounts.put(hashTag, count);
}
}
System.out.printf("Hash tag counts: %s %n", hashTagCounts);
}
}
when I use this I get 6 errors.
What is wrong here.

Dalton Coble
6,489 PointsExample.java:30: error: illegal start of type
for (Treet treet : treets) {
^
Example.java:30: error: ')' expected
for (Treet treet : treets) {
^
Example.java:30: error: <identifier> expected
for (Treet treet : treets) {
^
Example.java:40: error: <identifier> expected
System.out.printf("Hash tag counts: %s %n", hashTagCounts);
^
Example.java:40: error: illegal start of type
System.out.printf("Hash tag counts: %s %n", hashTagCounts);
^
Example.java:40: error: <identifier> expected
System.out.printf("Hash tag counts: %s %n", hashTagCounts);
^
6 errors
these are my errors.
1 Answer
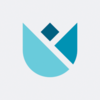
Jannes Blume
4,107 PointsHello Jody,
Although I haven't seen the video your post references to, I assume that you have tried to write a method that counts hashtags and puts them into a HashMap with the count being their value.
Now here's what mistakes I noticed:
- You placed code outside a method (beginning at the initialization of hashTagCounts).
- You are trying to access an object initialized in the main method, which is not visible due to the fact that it is stored in a method variable.
You can try the following:
import java.util.Arrays;
import java.util.Date;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
import java.util.TreeSet;
import com.teamtreehouse.Treet;
import com.teamtreehouse.Treets;
class Example {
private static Treet[] treets = Treets.load();
public static void main(String[] args) {
System.out.printf("There are %d treets. %n",
treets.length);
Set<String> allHashTags = new HashSet<String>();
Set<String> allMentions = new TreeSet<String>();
for (Treet treet : treets) {
allHashTags.addAll(treet.getHashTags());
allMentions.addAll(treet.getMentions());
}
System.out.printf("Hash tags: %s %n", allHashTags);
System.out.printf("Mentions: %s %n", allMentions);
}
public void hashtags() {
Map<String, Integer> hashTagCounts = new HashMap<String, Integer>();
for (Treet treet : treets) {
for (String hashTag : treet.getHashTags()) {
Integer count = hashTagCounts.get(hashTag);
if (count == null) {
count = 0;
count ++;
hashTagCounts.put(hashTag, count);
}
}
}
System.out.printf("Hash tag counts: %s %n", hashTagCounts);
}
}
I put your code outside of any method into the method hashtags() and changed treets to be a static class variable.
By now calling the new method, you should be able to run the desired code.

Dalton Coble
6,489 PointsThank you for your response.
Craig Dennis
Treehouse TeacherCraig Dennis
Treehouse TeacherCan you also post the errors please?
I wrapped your code with three back ticks followed by java to get the highlighting.