Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial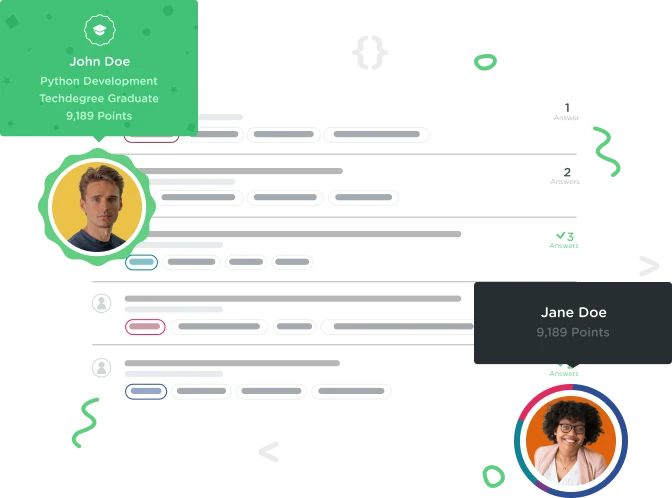
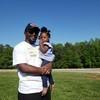
Darius Hill
8,545 PointsMy code work for this challenge. Looking for tips to make it better.
var correctAnswer= 0;
var questionOne = prompt( ' How old am I?');
var questionTwo = prompt( ' What is my name?');
var questionThree = prompt(' How many kids do I have?');
var questionFour = prompt(' What is my favorite NFL team');
var questionFive = prompt( ' What color is my car?');
if (parseInt(questionOne)=== 28) {
correctAnswer += 1
}
if (questionTwo.toUpperCase() === 'DARIUS') {
correctAnswer += 1 }
if (parseInt(questionThree) === 2) {
correctAnswer += 1 }
if (questionFour.toUpperCase() === 'BILLS') {
correctAnswer += 1 }
if (questionFive.toUpperCase() === 'GREEN') {
correctAnswer += 1 }
if (correctAnswer === 5 ) {
document.write('<p>Gold crown</p>');
} else if (correctAnswer === 4 || correctAnswer === 3) {
document.write('<p>Silver crown</p>');
} else if (correctAnswer === 2 || correctAnswer === 1) {
document.write('<p>Bronze crown</p>');
} else if (correctAnswer === 0) {
document.write('<p>No corwn rewared</p>');
}
2 Answers

Wiktor Bednarz
18,647 PointsHello Darius,
your code is clean and understandable as it is. It would be hard to make it better by introducing as few new concepts for you as possible, however I can try to keep the basic code of your app and restructure it to be more update friendly and a bit more elegant.
var correctAnswer= 0;
// Changing both answers and questions into an array.
// This way you can easily expand more question-answer pairs into your quiz for future use,
// and the whole app looks a little cleaner
//
// However using an object to store those question-answer pairs would be even better -
// - skipping this solution simply because I don't know if you are familiar with JS objects yet.
var Answers = [];
Answers.push(28);
Answers.push('DARIUS');
Answers.push(2);
Answers.push('BILLS');
Answers.push('GREEN');
var questions = [];
questions.push(parseInt(prompt( ' How old am I?')));
questions.push(prompt( ' What is my name?').toUpperCase());
questions.push(parseInt(prompt(' How many kids do I have?')));
questions.push(prompt(' What is my favorite NFL team').toUpperCase());
questions.push(prompt( ' What color is my car?').toUpperCase());
// By changing questions and answers into array,
// you can simply loop through both of them and compare parallel values.
// This function will work no matter how many question-answer pairs you've got.
//
// Sidenote: you could use more compact mapping function here. I suggest you to read on .map() method on MDN.
function quiz(Answers, questions) {
for (let i=0; i<Answers.length; i++) {
if (Answers[i]===questions[i]) {
correctAnswer++;
}
}
}
// Chaning the scoring part into an independent function as well
// lets you easily change the system in future iterations of the app.
// Maybe you'll want more complex scoring system by adding more grades?
//
// Either way, modular composition of seperate tasks into functions lets you
// and other programmers more easily understand and change the code.
function score(correctAnswer) {
if (correctAnswer === 5 ) {
document.write('<p>Gold crown</p>');
} else if (correctAnswer === 4 || correctAnswer === 3) {
document.write('<p>Silver crown</p>');
} else if (correctAnswer === 2 || correctAnswer === 1) {
document.write('<p>Bronze crown</p>');
} else if (correctAnswer === 0) {
document.write('<p>No corwn rewared</p>');
}
}
// After establishing all the functions, we can call them to execute the program.
quiz(Answers, questions);
score(correctAnswer);
I think that this modification doesn't rely on many concepts and functions that aren't known to you, but it will show you some basic principles and tips of writing modular and easily updatable code.
Hope this helps and keep up the good work man!
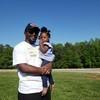
Darius Hill
8,545 PointsThanks for the reply Wiktor.