Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial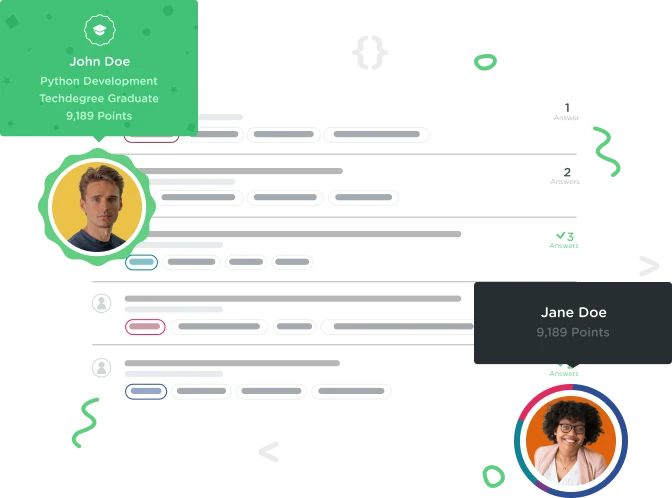
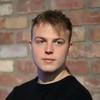
Kit Howlett
6,774 PointsMy code worked but isn't exactly the same. Is that okay?
Hi,
Before Dave showed his solution I tried to write this program. I managed to get a similar outcome although my code is very different. (See below)
Is this an issue. How can I learn how to get to the answer is the most efficient way. Does it just take lots of practice?
var randomNumber = getRandomNumber(10000);
var counter = 1;
var guess = true;
function getRandomNumber(upper) {
return Math.floor( Math.random() * upper) + 1;
}
while (guess) {
var guessNumber = getRandomNumber(10000);
if (guessNumber == randomNumber) {
guess = false;
} else {
counter += 1;
guessNumber = getRandomNumber(10000);
}
}
alert(`The number was ${randomNumber}. It took ${counter} guesses.`);
Thank you!
Kit :)
1 Answer

Firas Al-Mahrouqi
2,750 PointsWouldn't updating the counter before making a guess result in a wrong count should the loop terminate on the first attempt?
Broderick Lemke
13,483 PointsBroderick Lemke
13,483 PointsHi Kit! This snippet of code looks good to me. There are a few key differences with your code and Dave's but from what I'm seeing there isn't a major issue with efficiency. Over time you will learn things that can really take a hit on efficiency (traveling through an array multiple times, looping more than needed, connecting to an outside datasource repeatedly) and will come up with ways to work around them.
I would say that Dave's code might be more readable and maintainable where as you have a lot more going on, but this isn't a wrong answer at all. I do see a bit of your code that could be improved though, which would help the readability! Let's imagine our randomNumber picked at the start of the program is 1337. We then enter the while loop because guess is true. We set guessNumber to a random number, let's say 1853. We check if they're equal and they're not, so we go into the else statement. We add 1 to the counter and pick a new random value for guessNumber: 1963. Now we get to the end of the loop and check if guess is still true which it is. Everything seems to work so far but then we generate a new random number. We never used the 1963 we generated in the else statement. I would update the code like this:
As a final note: If you're really interested in learning more about efficiency you could always look into Big O notation which is a system that is used to describe how well a piece of code will scale and if it is suitable for the amount of data you're dealing with and performance level you are looking for.
P.S. Nice work with the template literals in your alert at the end :)