Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial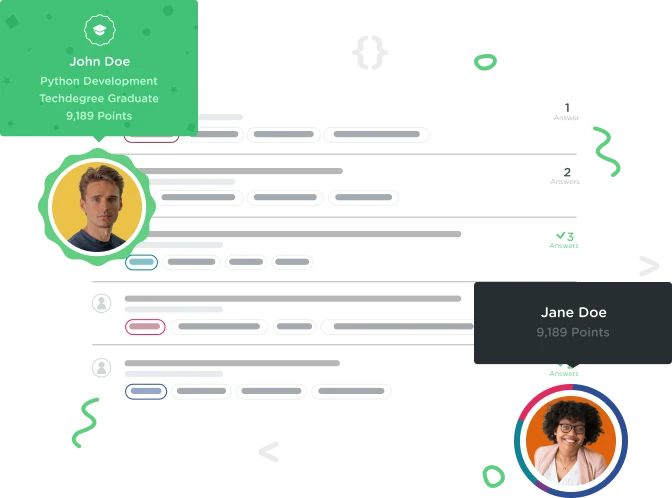

Samuel Cleophas
12,348 PointsMy Code Works! But it's telling me it's wrong
Here is the code
class Student {
constructor(gpa, credits){
this.gpa = gpa;
this.credits = credits;
}
get level() {
if (this.credits <= 30) {
return 'Freshman';
} else if (this.credits <= 60) {
return 'Sophmore';
} else if (this.credits <= 90) {
return 'Junior';
} else {
return 'Senior';
}
}
stringGPA() {
return this.gpa.toString();
}
}
const student = new Student(3.9);
2 Answers
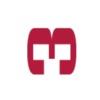
trio-group I.AM
25,711 PointsHey!
Your code is totally valid! You simply have a typo in the word 'Sophomore'.
You can make your code more specific, like this:
get level() {
if (this.credits <= 30) {
return 'Freshman';
} else if (this.credits > 30 && this.credits <= 60) {
return 'Sophomore';
} else if (this.credits > 60 && this.credits <= 90) {
return 'Junior';
} else {
return 'Senior';
}
}
But correcting only the typo will also do the trick!
Hope this helps!

Antonio De Rose
20,884 Pointsget level() {
if (this.credits <= 30) {// this falls into the bracket under 30 and equal
return 'Freshman';
} else if (this.credits <= 60) { //this falls into under 60 and equal, that means, it covers the less than 30 or equal too
return 'Sophmore';
} else if (this.credits <= 90) { this covers all of this, and under 60 and under 30
return 'Junior';
} else {
return 'Senior';
}
}
//you need to write the condition, for the for scenarios / conditions, to fit into, one bracket at a given time,
//not to fall into multiple brackets.
//you can compare and combine, to have the condition, checked between 30 and 60
//by using || operator for OR, and && operator for AND
//as you read the question it is better, to approach in the same order, as the question, gives you
//the scenario of 90 to start with, but your condition starts with 30