Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial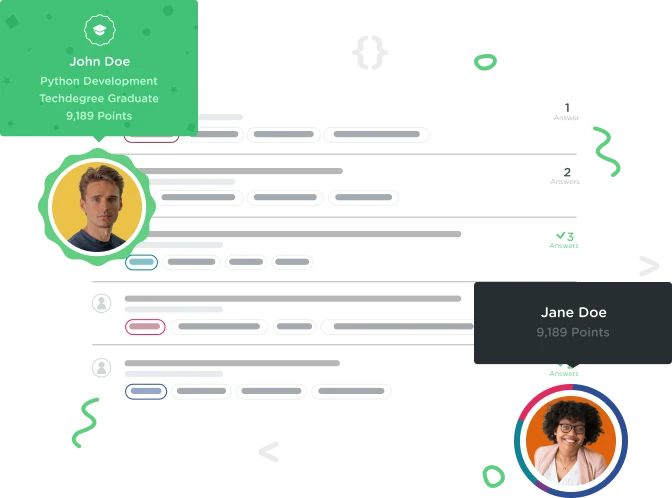

stella grady
332 PointsMy code works but still got a message saying otherwise
for each contact i print the value of its name and phone number. isnt that what im suppose to do?
contact_list = [
{"name" => "Jason", "phone_number" => "123"},
{"name" => "Nick", "phone_number" => "456"}
]
contact_list.each do |contact|
contact.each_value do |value|
puts value
end
end
1 Answer
John Coolidge
12,614 PointsHello Stella,
The exercise wants you to print out each name and phone number using the puts method by using the .each method.
When you structure your .each method, you take the name of the hash or array (contact_list), add .each to it, followed by do, followed by a variable, in this case, contact. You have as much in your example:
contact_list.each do |contact|
contact.each_value do |value|
puts value
end
The first problem is, you've got an unnecessary .each method in your example above. You can remove it, leaving this:
contact_list.each do |contact|
puts value
end
Now you're getting closer. You will need to print out both the contact name and phone number to the screen. So, the next question is, how do you access the values in a hash? You need to use the following:
contact["name"] #to access the name
contact["phone_number"] #to access the phone number
Each hash in the array is saved under the local variable "contact" and in order to access the key "name" you need to put it in brackets after the hash, which in this case is stored in the variable "contact".
So, in order to put all of this together you need to set up your .each method as I described above.
When you decide what you want to puts, namely the contact information, all you need to do is supply the contact["name"] and the contact["phone_number"] after "puts", using a comma to separate them.
contact_list.each do |contact|
puts contact["name"], contact["phone_number"]
end
I hope this assists in explaining how to access hash key/values in an array using the .each method.
John