Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial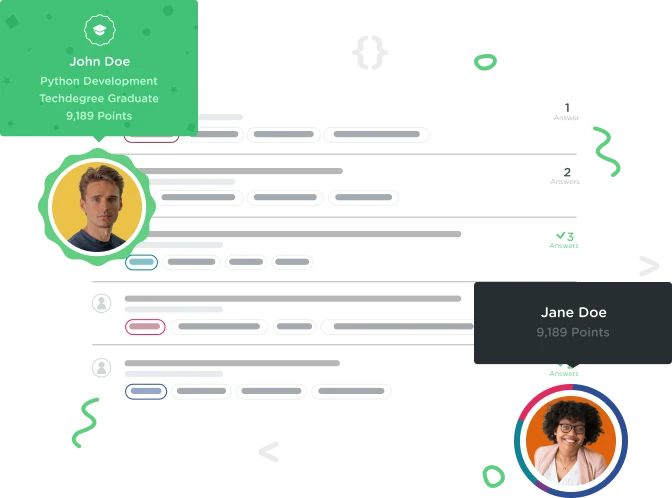
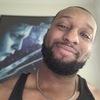
Brandon White
Full Stack JavaScript Techdegree Graduate 35,771 PointsMy Code Works in the REPL, but the output from the challenge is Bummer: reverse didn't accept an argument
backwards = [
'tac',
'esuoheerT',
'htenneK',
[5, 4, 3, 2, 1],
]
def reverse(iterable):
def switch(item):
return item[::-1]
return list(map(switch, iterable))
treehouse:~/workspace$ python
Python 3.9.0 (default, Jan 21 2021, 00:46:14)
[GCC 5.4.0 20160609] on linux
Type "help", "copyright", "credits" or "license" for more information.
>>> backwards = [
... 'tac',
... 'esuoheerT',
... 'htenneK',
... [5, 4, 3, 2, 1],
... ]
>>>
>>> def reverse(iterable):
... def switch(item):
... return item[::-1]
... return list(map(switch, iterable))
...
>>> frontwards = reverse(backwards)
>>> frontwards
['cat', 'Treehouse', 'Kenneth', [1, 2, 3, 4, 5]]
>>>
When I test my code in the REPL, I get a list with the iterable reversed. If I understood the reasoning behind the error I think I could probably figure it out on my own. But I don't get why my function wouldn't be accepting an argument.
Any help would be mightily appreciated.
I thought maybe the issue was the challenge didn't like me nesting a function in reverse, so I tried the following, but I got the same error:
backwards = [
'tac',
'esuoheerT',
'htenneK',
[5, 4, 3, 2, 1],
]
def reverse(iterable):
return [item[::-1] for item in iterable]
1 Answer
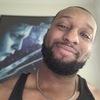
Brandon White
Full Stack JavaScript Techdegree Graduate 35,771 PointsPlease disregard. I misunderstood what the challenge was asking.
The following passed the first part of the challenge:
def reverse(item):
return item[::-1]
>>> def reverse(item):
... return item[::-1]
...
>>> frontwards = reverse(backwards)
>>> frontwards
[[5, 4, 3, 2, 1], 'htenneK', 'esuoheerT', 'tac']