Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial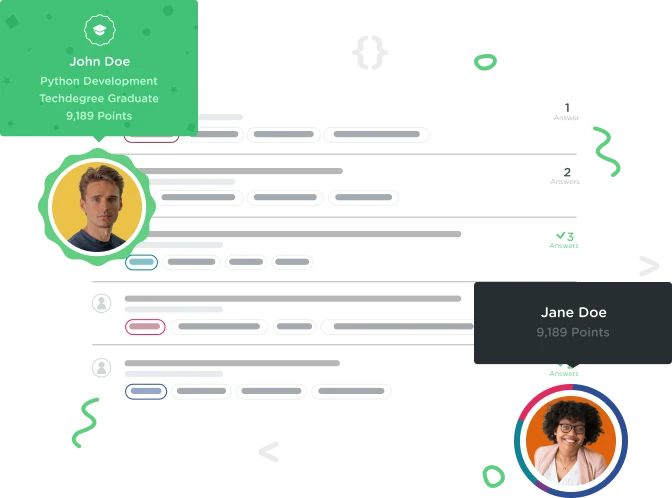
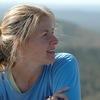
Annika Noren
26,930 PointsMy code works in workspaces, but not in in the code challenge. It is challenge #4 of 5: def most_courses
Here is my code (it works in workspaces):
def most_courses(my_dict): pairs = {} for teacher in my_dict: counter = 0 for course in my_dict[teacher]: counter += 1 pairs.update({teacher : counter}) max_value = max(pairs.values()) max_key = [k for k, v in pairs.items() if v == max_value] return(max_key)
I realize it is not as clean as other solutions, but I'd still like to figure out why it doesn't pass.
# The dictionary will look something like:
# {'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Each key will be a Teacher and the value will be a list of courses.
#
# Your code goes below here.
def num_teachers(my_dict):
return(len(my_dict))
def num_courses(my_dict):
return(sum(len(value) for value in my_dict.values()))
def courses(my_dict):
course_list = list()
for teacher in my_dict.keys():
for course in my_dict[teacher]:
course_list.append(course)
return(course_list)
def most_courses(my_dict):
pairs = {}
for teacher in my_dict:
counter = 0
for course in my_dict[teacher]:
counter += 1
pairs.update({teacher : counter})
max_value = max(pairs.values())
max_key = [k for k, v in pairs.items() if v == max_value]
return(max_key)
4 Answers
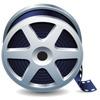
John Goodwin
17,921 PointsHi Annika,
I copied and pasted your code from the code block and it passed the challenge. I'm not sure why it isn't passing for you. This is the part after teachers.py, not the few lines at the top.
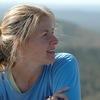
Annika Noren
26,930 PointsHi John, Thank you for confirming my code (not the stuff at the top, but the code in the black space.) I appreciate your time
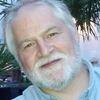
Jeff Muday
Treehouse Moderator 28,716 PointsYou did a really nice job on the coding this up. But the automated test wants a STR return rather than a LIST type return. One modification should make it work for you, see below comment on last line.
You are well prepared for the last part of the challenge with your "pairs" dictionary concept.
Best of luck on your Python journey, may it bring you great rewards!
def most_courses(my_dict):
pairs = {}
for teacher in my_dict:
counter = 0
for course in my_dict[teacher]:
counter += 1
pairs.update({teacher : counter})
max_value = max(pairs.values())
max_key = [k for k, v in pairs.items() if v == max_value]
return(max_key[0]) # return the first item and it will work
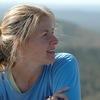
Annika Noren
26,930 PointsThank you, Jeff! Much appreciated.