Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial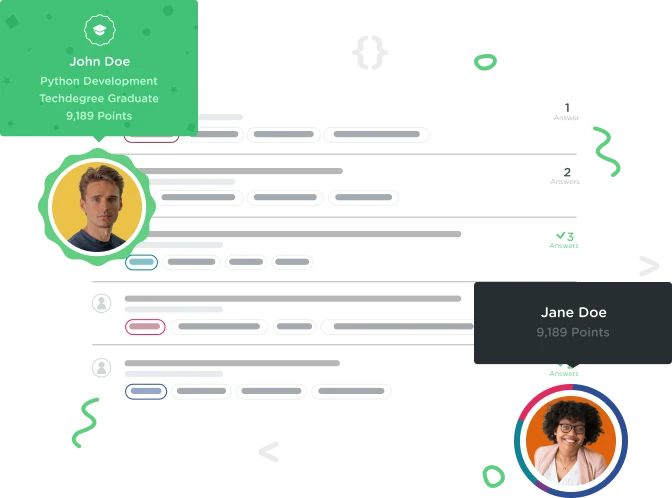

Rick Hoen
1,257 PointsMy coding seems correct, but I do not pass.
Hi!
I coded this challenge with "if" and "else if" statements.
The coding seems to be correct, but I do not pass the challenge. It keeps saying that I should check my logic for FizzBuzz values and make sure I return the correct string.
What is going wrong here?
Cheers, Rick
func fizzBuzz(n: Int) -> String {
// Enter your code between the two comment markers
if (n%3 == 0) {
return "Fizz"
}
else if (n%5 == 0) {
return "Buzz"
}
else if (n%3 == 0) && (n%5 == 0) {
return "FizzBuzz"
}
// End code
return "\(n)"
}
2 Answers

Jon Brantingham
7,860 PointsYou need to place the most restrictive test at the top.
This means divisible by 3 and 5. The way you have it, 15 would print fizz. Being divisible by 3 is not implied, but the final return does imply that is not divisible by 3 or 5.
You should also place your else if statements on the same line as the close curly brace. Swift Style Guide
func fizzBuzz(n: Int) -> String {
// Enter your code between the two comment markers
if (n%3 == 0) && (n%5 == 0) {
return "FizzBuzz"
} else if (n%3 == 0) {
return "Fizz"
} else if (n%5 == 0) {
return "Buzz"
}
return "\(n)"
// End code
}
fizzBuzz(n: 3) //Returns "Fizz"
fizzBuzz(n: 5) //Returns "Buzz"
fizzBuzz(n: 15) //Returns "FizzBuzz"
fizzBuzz(n: 16) //Returns "16"
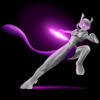
Tiwong Nance, Jr.
19,650 PointsHello Rick. The code seems right, but you need to change the order of execution. Since we want to check if something is divisible by 5 or 3, we need to do the one with both first. Because the if statement only executes the first true statement, "FizzBuzz" would never be returned. Also, we can just put else for the last statement, because it's implied that the number must be divisible by 3. Your code should look similar to this:
func fizzBuzz(n: Int) -> String {
// Enter your code between the two comment markers
if (n%3 == 0) && (n%5 == 0) {
return "FizzBuzz"
} else if (n%5 == 0) {
return "Buzz"
}
else {
return "Fizz"
} // End code
return "(n)"
}
Hope this helped. :)
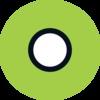
Blair Rorani
6,658 PointsWhy is it implied? Because of the first if? What if I entered the value of 4 - what would the function return?