Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial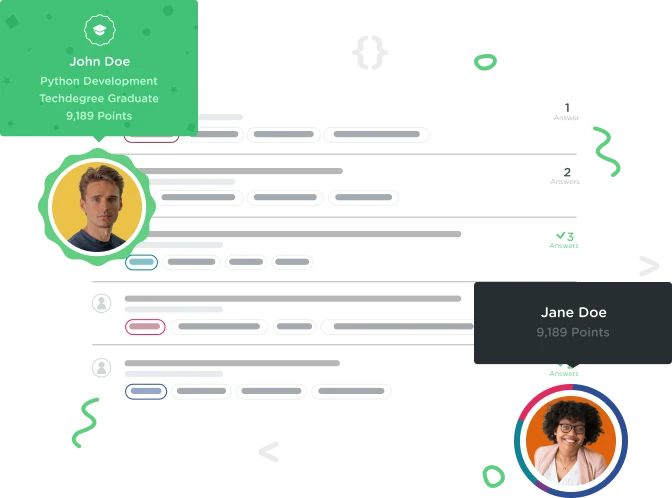
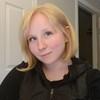
Serina C.
Python Development Techdegree Student 2,357 PointsMy Conditional Challenge Solution - Input Welcome!
Does this look okay? Is there anything I can improve on?
Thanks!
alert('Can you guess the endings of these spicy Vines?');
var wrongScore = 0;
var rightScore = 0;
var guess1 = prompt ('I want to be a (...) (...)');
if (guess1.toUpperCase() === 'COWBOY BABY') {
++rightScore;
alert ('Correct!');
} else {
++wrongScore;
alert ('Thats wrong.');
}
var guess2 = prompt ('Happy Crimus. Its Crismun. Merry Crisis. Merry (...) ');
if (guess2.toUpperCase() === 'CHRYSLER') {
++rightScore;
alert ('Correct!');
} else {
++wrongScore;
alert ('Thats wrong.');
}
var guess3 = prompt ('Whats better that this? Guys bein (...)');
if (guess3.toUpperCase() === 'DUDES') {
++rightScore;
alert ('Correct!');
} else {
++wrongScore;
alert ('Thats wrong.');
}
var guess4 = prompt ('Oh hi, thanks for checking in Im still a piece of (...)');
if (guess4.toUpperCase() === 'GARBAGE') {
++rightScore;
alert ('Correct!');
} else {
++wrongScore;
alert ('The only thing thats garbage is that guess! WRONG.');
}
var guess5 = prompt ('I spilled lipstick in your Valentino bag." "You spilled — whaghwhha — lipstick in my (...) (...) (...)?');
if (guess5.toUpperCase() === 'VALENTINO WHITE BAG') {
++rightScore;
alert ('Correct!');
} else {
++wrongScore;
alert ('Thats wrong.');
}
if ( rightScore === 5) {
document.write ('<p> Wow! You really know your stuff! Your final score is ' + rightScore + ' correct, and ' + wrongScore + ' wrong.</p> <p>You get the gold crown! </p>');
} else if ( rightScore === 4 || rightScore === 3) {
document.write ('<p> Not bad. You know enough, but you should still study up! Your final score is ' + rightScore + ' correct, and ' + wrongScore + ' wrong.</p> <p> You get the silver crown. </p>');
} else if ( rightScore === 2 || rightScore === 1) {
document.write ('<p> Did you even try? Your final score is ' + rightScore + ' correct, and ' + wrongScore + ' wrong.</p> <p> You get the bronze crown. </p>');
} else {
document.write ('<p> DISAPOINTMENT!</p> <p> You get nothing. </p>');
}
2 Answers
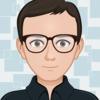
Armin Kadic
16,242 PointsUsually what I like to do is compare my code with someone else's. That way I can maybe learn something new, a different approach with the same solution. Your code is really nice and clean, there are some details that I learned from your code to be honest :) like using "(...)" the way you did, and there are some details that I would change like the "( rightScore === 4 || rightScore === 3)". But I can't judge since I'm not an expert, so I'm going to paste in my solution for this challenge for you to see. I usually try to make the code as short as possible. I didn't update it because of the material presented so far to that point. Just remember that in coding there are often multiple ways to solve a problem!
// Quiz begins, no answers correct
let correct = 0;
// Ask Questions
const planet = prompt("What planet are we on?");
if ( planet.toUpperCase() === "EARTH") {
correct += 1;
}
const cover = prompt("What covers 71% of the earth?");
if ( cover.toUpperCase() === "WATER") {
correct += 1;
}
const friend = prompt("What animal is the best friend to a human?");
if ( friend.toUpperCase() === "DOG") {
correct += 1;
}
const sky = prompt("What is the color of the sky?");
if ( sky.toUpperCase() === "BLUE") {
correct += 1;
}
const humans = prompt("What keeps humans together?");
if ( humans.toUpperCase() === "LOVE") {
correct += 1;
}
// Output results
document.write("<h1>You got " + correct + " out of 5 questions correct.</h1>");
// Output rank
if ( correct === 5 ) {
document.write("<h1><strong>You earned a gold crown!</strong></h1>");
} else if ( correct >= 3 ) {
document.write("<h1><strong>You earned a silver crown.</strong></h1>");
} else if ( correct >= 1 ) {
document.write("<h1><strong>You earned a bronze crown.</strong></h1>");
} else {
document.write("<h1><strong>No crown for you...</strong></h1>");
}
Ps. My code is far from perfect! :)
I hope this helps a little.
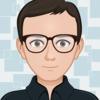
Armin Kadic
16,242 PointsI'm really glad that I could help! As you advance, you will start to see all the ways you can improve your old code. One thing that I would change in the past is to not focus too much on the basics, of course you should have good understanding of it (which I see you do), but later you will learn more methods to do something. It kinda gets harder but also easier in the same time. Don't let me confuse you, that is just my opinion. The code will be easier to read, but there will also be many new ways to do something... ahh, nevermind me :) just keep going as you do! Oh and btw, don't forget to do this course:
https://teamtreehouse.com/library/getting-started-with-es2015-2
It really helps to get up to date with JavaScript and learn all the good new stuff! I would recommend to first finish all about JavaScript on that track, and then to do this JavaScript update.
Have fun coding and good luck! :)
Serina C.
Python Development Techdegree Student 2,357 PointsSerina C.
Python Development Techdegree Student 2,357 PointsHey, thanks for getting back to me super quickly!
Posting your code for comparison was actually super helpful and much appreciated! When I'm doing these challenges, I like to research a bit before I actually start so that I can have some kind of idea of how I'd like to approach the task. So I usually run through everyone's solutions really-really quickly, pick out a few pieces of code that I may have questions about, then hop onto stackoverflow or MDN to clarify. Like, I wasn't really familiar with <strong></strong> , and also '++' for adding points to a score, but I definitely saw those 2 concepts floating around in the answers, as well as in your own.
So, being able to compare to someone else's solution is really important for me to understand how I can improve or adjust my own code. Like with your code, I see that I could have used += rather than ++, and I could have really shortened up the end by using >= rather than going for the 'or operator'.
If I had to re-do it, I would make those 2 corrections, and I also would probably get rid of the "wrong" and "right" alerts. I also like that you mentioned keeping your code short. I'll keep that in mind for the next challenge, because it was sort of unnecessary to add all that extra 'fluff'. And, I think that your solution is much more concise and easier to read than mine is, if I'm honest :)
Thanks again for the quick response and for your input!! I really appreciate it!!