Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial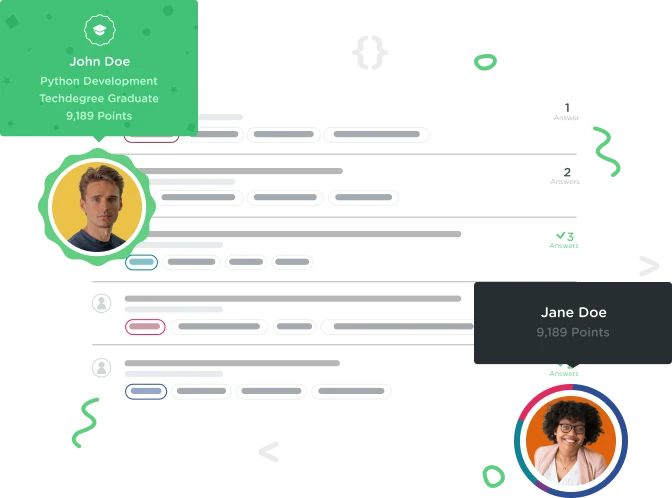

Jacob Correa
Full Stack JavaScript Techdegree Student 1,303 PointsMy conditional statements sometimes work and sometimes don't. Using || and &&
My first 3 questions had 3 possible answers.
I used the "or" operator to allow answer1, answer2 or answer3 to work for the first 3 questions and the code was successful.
I also included an && operator so that:
The SECOND "if statement" makes sure you didn't give the same answer that you gave for the first question.
The THIRD "if statement" makes sure you didn't give the same answer you gave for the first or second questions.
This code is SOMETIMES successful:
-
If you give
answer1
,answer2
, andanswer3
for questions 1, 2, and 3, in any order... each answer is considered correct.- That is successful. :D
-
If you give
answer1
for all 3 questions... each answer is considered correct.- That is unsuccessful. :(
-
If you give
answer2
for all 3 questions... each answer is considered correct.- That is unsuccessful. :(
If you give answer3
for all 3 questions... the first answer is correct, the second answer is incorrect, and the third answer is also incorrect.
- That is successful. :D
Why does answer3 yield the intended result, while answer1 and answer2 do not?
/*
1. Store correct answers
- When quiz begins, no answers are correct
*/
const answer1 = 'Consistent';
const answer2 = 'Persistent';
const answer3 = 'Insistent';
const answer4 = 'Benzaldehyde';
const answer5 = 'In This Sign Thou shalt Conquer';
// 2. Store the rank of a player
let rank = 0;
// 3. Select the <main> HTML element
const main = document.querySelector('main')
/*
4. Ask at least 5 questions
- Store each answer in a variable
- Keep track of the number of correct answers
*/
let playerAnswer1 = prompt('What is one of the principles of the acronym CPI?');
let playerAnswer2 = prompt('What is another principle of the acronym CPI?');
let playerAnswer3 = prompt('What is the final principle of the acronym CPI?');
let playerAnswer4 = prompt('What chemical is the base component of Cherry Flavors?');
let playerAnswer5 = prompt('What does the latin phrase In Hoc Signo Vinces translate to in English?');
if ( playerAnswer1.toUpperCase() === answer1.toUpperCase() || playerAnswer1.toUpperCase() === answer2.toUpperCase() || playerAnswer1.toUpperCase() === answer3.toUpperCase() ) {
rank += 1;
console.log(playerAnswer1);
console.log('1: correct');
}
if ( playerAnswer2.toUpperCase() === answer1.toUpperCase() || playerAnswer2.toUpperCase() === answer2.toUpperCase() || playerAnswer2.toUpperCase() === answer3.toUpperCase() && playerAnswer2.toUpperCase() != playerAnswer1.toUpperCase() ) {
rank += 1;
console.log(playerAnswer2);
console.log('2: correct');
}
if ( playerAnswer3.toUpperCase() === answer1.toUpperCase() || playerAnswer3.toUpperCase() === answer2.toUpperCase() || playerAnswer3.toUpperCase() === answer3.toUpperCase() && playerAnswer3.toUpperCase() != playerAnswer1.toUpperCase() && playerAnswer3.toUpperCase() != playerAnswer2.toUpperCase() ) {
rank += 1;
console.log(playerAnswer3);
console.log('3: correct');
}
if ( playerAnswer4.toUpperCase() === answer4.toUpperCase() ) {
rank += 1;
console.log(playerAnswer4);
console.log('4: correct');
}
if ( playerAnswer5.toUpperCase() === answer5.toUpperCase() ) {
rank += 1;
console.log(playerAnswer5);
console.log('5: correct')
}
/*
5. Rank player based on number of correct answers
- 5 correct = Gold
- 3-4 correct = Silver
- 1-2 correct = Bronze
- 0 correct = No crown
*/
let medal = '';
if ( +rank === 5 ) {
medal = "Gold";
} else if ( 5 > +rank && +rank >= 3 ) {
medal = "Silver";
} else if ( +rank < 3 && 0 < +rank ) {
medal = "Bronze";
} else {
medal = "No crown";
}
// 6. Output results to the <main> element
console.log(medal);
main.innerHTML = `Your score has earned you ${medal}. You truly deserve this.`;
Please let me know if you can make any sense of this
Thanks, Jake
1 Answer
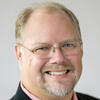
Jason Larson
8,361 PointsThis has to do with the way the AND and OR operators are being processed, kind of like the order of operations with math operators. If you group all your ORs together, it should work. I'm only showing the body of the ifs for brevity, but I've added line breaks to make the grouping easier to see (I hope):
if (playerAnswer1.toUpperCase() === answer1.toUpperCase()
|| playerAnswer1.toUpperCase() === answer2.toUpperCase()
|| playerAnswer1.toUpperCase() === answer3.toUpperCase()
) {}
if (
(playerAnswer2.toUpperCase() === answer1.toUpperCase()
|| playerAnswer2.toUpperCase() === answer2.toUpperCase()
|| playerAnswer2.toUpperCase() === answer3.toUpperCase()
) && playerAnswer2.toUpperCase() != playerAnswer1.toUpperCase()
) {}
if (
(playerAnswer3.toUpperCase() === answer1.toUpperCase()
|| playerAnswer3.toUpperCase() === answer2.toUpperCase()
|| playerAnswer3.toUpperCase() === answer3.toUpperCase()
) && playerAnswer3.toUpperCase() != playerAnswer1.toUpperCase()
&& playerAnswer3.toUpperCase() != playerAnswer2.toUpperCase()
) {}
By the way, you can save yourself a little bit of typing by making your answers all uppercase when you define them, and making the playerAnswers uppercase when they enter them, then you only have to do the comparisons, rather than converting the strings when you compare them.
const answer1 = 'CONSISTENT';
...
let playerAnswer1 = prompt('What is one of the principles of the acronym CPI?').toUpperCase();
let playerAnswer2 = prompt('What is another principle of the acronym CPI?').toUpperCase();
let playerAnswer3 = prompt('What is the final principle of the acronym CPI?').toUpperCase();
...
if (playerAnswer1 === answer1 || playerAnswer1 === answer2 || playerAnswer1 === answer3) {}
...
Jacob Correa
Full Stack JavaScript Techdegree Student 1,303 PointsJacob Correa
Full Stack JavaScript Techdegree Student 1,303 PointsThanks Jason! It worked!!
I'll keep that in mind for the future, along with your tips on more efficient code.
Much appreciated