Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial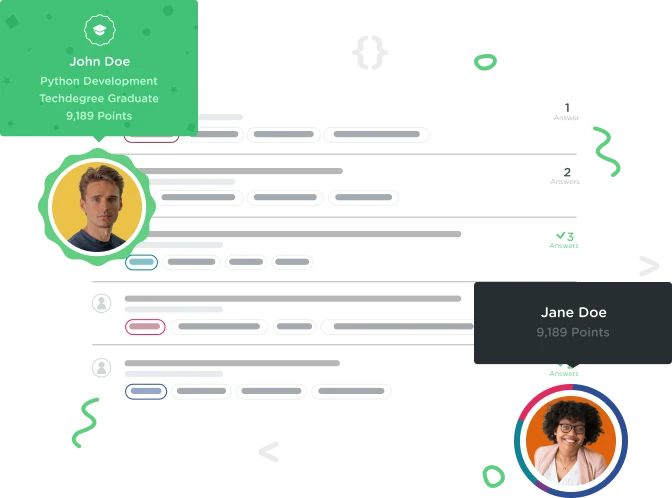

Rodwell Mazambara
1,402 PointsMy covers function is working fine in workspaces but failing in code challenge
My covers function is working fine in workspaces but failing in code challenge. I can't figure out why.
COURSES = {
"Python Basics": {"Python", "functions", "variables",
"booleans", "integers", "floats",
"arrays", "strings", "exceptions",
"conditions", "input", "loops"},
"Java Basics": {"Java", "strings", "variables",
"input", "exceptions", "integers",
"booleans", "loops"},
"PHP Basics": {"PHP", "variables", "conditions",
"integers", "floats", "strings",
"booleans", "HTML"},
"Ruby Basics": {"Ruby", "strings", "floats",
"integers", "conditions",
"functions", "input"}
}
def covers (courses_set):
courses_list=[]
for course in courses_set:
for course_title in COURSES:
if course in course_title:
courses_list.append(course_title)
return courses_list
4 Answers

Mike Wagner
23,559 PointsYou're actually quite close. It seems that what you're doing right now is checking the passed in argument (in the example this is covers({"Python"})
) against the course names, rather than the topics the course covers. If you were to change the function call to covers({"boolean"})
or covers({"floats"})
you would be returning an empty list, despite multiple courses covering those topics. However, by using something like covers({"Basics"})
you return the course names even though none of the courses covers a topic called such.
Simply put, you're just not reaching down far enough and checking against the right things. You should be able to sort this out on your own now, but if you have any further problem, feel free to drop a comment on this answer and I'll help as I can.

Rodwell Mazambara
1,402 PointsThanks Mike. I have modified my code and I thought my code was now searching at the right level. Can you figure out what is wrong with what I have done? I am at my wits' end.
def covers (courses_set):
courses_list=[]
for search_item in courses_set:
for key in COURSES.keys():
if search_item in COURSES[key]:
courses_list.append(key)
return courses_list

Mike Wagner
23,559 PointsThis could be related to the fact that you're not checking if the item is already in the list before appending it. I checked your new code in my interpreter and it seems to now return the course titles properly, but if I were to use something resembling covers({"integers", "variables"})
it will create duplicates in the list, returning ['Python Basics', 'Java Basics', 'PHP Basics', 'Ruby Basics', 'Python Basics', 'Java Basics', 'PHP Basics']
instead. If you do a quick check to make sure the key
isn't already in courses_list
before you append it, you should be good :)

Rodwell Mazambara
1,402 PointsThanks Mike. I have modified the code to check if the course title is already in the list but again I am getting no joy.
def covers (courses_set):
courses_list=[]
for search_item in courses_set:
for key in COURSES.keys():
if search_item in COURSES[key]:
if key not in courses_list:
courses_list.append(key)
return courses_list

Mike Wagner
23,559 PointsThis version of your function works fine for the Challenge. I'm assuming you changed something else, added in a print statement that you didn't need, or something else. If you take the list COURSES that shows up with a restart on the Challenge and copy/paste this function in beneath it, you'll be fine to move forward

Rodwell Mazambara
1,402 PointsThought as much Mike. I ended up following Greg's logic but I am pretty positive that using the logic above should equally work. Thanks hey!
Rodwell Mazambara
1,402 PointsRodwell Mazambara
1,402 PointsThanks Mike. I have modified my code and I thought my code was now searching at the right level. Can you figure out what is wrong with what I have done? I am at my wits' end.