Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial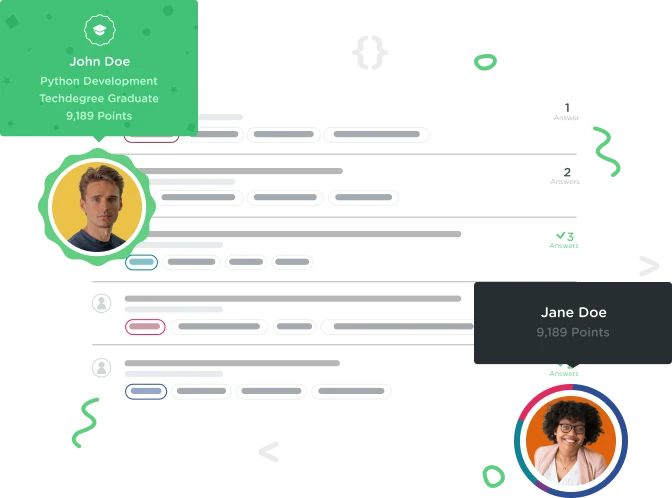

orange sky
Front End Web Development Techdegree Student 4,945 Pointsmy div is sticking out of my column
Hello!
If you run this code, you will see the div with and id called icon:
1) It is sticking out of the column. How can I fix that?
2) the image in the div does seem centered. Is it centered for you. How can I prove that it is centered?
thanks!
<!DOCTYPE html>
<html>
<head>
<title>Positioning Schemes</title>
<meta name="viewport" content="width=device-width">
<link rel="stylesheet" href="css/normalize.css">
<style>
body {
font: normal 1.1em/1.5 sans-serif;
color: #222;
background-color: #edeff0;
}
/* Layout Element Colors
================================ */
.main-header { background-color: #384047; }
.main-logo a { background-color: #5fcf80; }
.main-nav a { background-color: #3f8abf; }
.primary-content { background-color: #caebf6; }
.secondary-content { background-color: #bfe3d0; }
.main-footer { background-color: #b7c0c7; }
.main-header{
padding:15px;
min-height:150px;
}
.main-logo a, .main-nav a{
display:block;
text-decoration:none;
padding:10px 20px;
color:white;
border-radius:5px;
}
.main-nav li{
margin-top:8px;
border-radius:5px;
}
#icon {
background-color: #39add1;
margin-top: 34px;
height: 180px;
border-radius: 5px;
position: relative;
}
#icon:after{
content:"";
background: url('icon.png') no-repeat;
position:absolute;
background-size:100%;
display:inline-block;
width:150px;
height:150px;
top:0;
bottom:0;
left:0;
right:0;
margin: auto;
}
.col{
padding:20px;
}
.main-footer{
padding:15px;
text-align:center;
}
/*media query with relative
==========================================*/
@media(min-width:769px){
.main-header{
position:fixed;
top:0;
width:100%;
z-index:100;
}
body{
padding-top:150px;
font-size:16px;
}
.main-banner{
display:none;
}
.main-logo, .main-nav{
position:absolute;
}
.main-logo{
top:50px;
}
.main-nav li{
display:inline-block;
}
.main-nav{
top:70px;
left:200px;
}
.main-nav li{
margin-right:10px;
}
/*columns
===================*/
.content-row{
position:relative;
}
.col{
position:absolute;
width:30%;
}
.primary-content{
left:30%;
width:40%;
}
.secondary-content{
right:0;
}
html, body, .col, .content-row,.main-wrapper{
height:100%;
}
.feat-img{
float:left;
width:30%;
}
*{
box-sizing:border-box;
}
.group::after{
content:"";
display:block;
clear:both;
}
}
</style>
</head>
<body>
<div class="main-wrapper">
<header class="main-header">
<h1 class="main-logo"><a href="#">Logo</a></h1>
<ul class="main-nav group">
<li><a href="#">Link 1</a></li>
<li><a href="#">Link 2</a></li>
<li><a href="#">Link 3</a></li>
<li><a href="#">Link 4</a></li>
</ul>
</header>
<div class="main-banner">
<h1>This is the Main Banner Heading</h1>
<p>Andouille pork chop pancetta drumstick ground round beef ribs swine brisket ham.</p>
</div>
<div class="content-row group">
<div class="extra-content col">
<h3>Extra Content</h3>
<p>Filet mignon turkey flank doner strip steak. Frankfurter ham hock turkey, venison sirloin pig chuck shank capicola hamburger doner spare ribs boudin.</p>
<hr>
<p> Drumstick bresaola meatloaf ham hock salami tri-tip landjaeger beef filet mignon biltong boudin turkey.</p>
<div id="icon"></div>
</div>
<div class="primary-content col">
<h2>Primary Content</h2>
<img class="feat-img" src="http://lorempixel.com/400/300" />
<p>Bacon ipsum dolor sit amet chicken pork ground round brisket corned beef ball tip shank tail salami filet mignon ham hock pork belly venison shankle. Pig kielbasa drumstick sausage pork chop boudin. Chicken t-bone salami pork chop, beef ribs kevin ham tri-tip beef venison biltong brisket.</p>
<p>Venison strip steak meatball chicken, brisket prosciutto sirloin. Capicola drumstick brisket tri-tip salami. Chicken beef jerky, tail turkey prosciutto cow ham sirloin boudin tenderloin. Meatloaf tri-tip turducken brisket andouille, pork belly corned beef fatback hamburger.</p>
</div>
<div class="secondary-content col">
<h3>Secondary Content</h3>
<p>Strip steak tenderloin kevin swine meatloaf capicola, doner beef turducken pancetta corned beef pork loin shoulder.</p>
<hr>
<p>Pork filet mignon leberkas, tail swine venison pancetta turkey shoulder brisket chalkers likes hamburgers.</p>
</div>
</div>
<footer class="main-footer">
<p>©2014 Example Layout</p>
</footer>
</div>
</body>
</html>
5 Answers
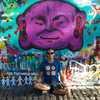
Andrew Stelmach
12,583 PointsCheck the codepen, Orange - the icon looks pretty centered to me!
As for 'why' it has to be 'min-height' and not 100% height, I wish I knew myself! I've had a google and a play around with it, but it's still a mystery to me also. I'm sure it's something to do with the positioning properties of the various elements causing the collapse, but I don't know more than I'm afraid. If you find out, do let me know!
As for centering the image, you could use width: 100%; or give it let less than 100% width and use margin-left: whatever; to centre it. Or you could give it position: relative; and then left: whatever;
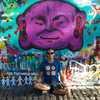
Andrew Stelmach
12,583 PointsDon't need the .group class on the content-row, because nothing's being floated, apart from the image.
The content row needs a 'min-height'. Giving it the following solves your problems:
.content-row { min-height: 550px; }
However, using the absolute positioning method for columns is fine, UNLESS you want the columns' sizes to adapt to the size of their content on their own - they won't, in a way i.e. if you put more content into the biggest column, you'll have to adjust the min-height of the content-row again.
But, using absolute positioning is useful if you want the columns to filled with colour, because when floating the columns, this is very difficult to achieve.
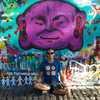
Andrew Stelmach
12,583 Points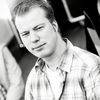
Geert-Jan Hendriks
23,126 PointsThe problem is the absolute positioning of your colums in the content-row div. You can float those divs, but the height won't be equal. There are some numerous fixes for this, in the example below i used a method to display the colums as a table-cell using display: table-cell and display: table.
The image is not centered, its width is 100%.
<!DOCTYPE html>
<html>
<head>
<title>Positioning Schemes</title>
<meta name="viewport" content="width=device-width">
<link rel="stylesheet" href="css/normalize.css">
<style>
body {
font: normal 1.1em/1.5 sans-serif;
color: #222;
background-color: #edeff0;
}
/* Layout Element Colors
================================ */
.main-header { background-color: #384047; }
.main-logo a { background-color: #5fcf80; }
.main-nav a { background-color: #3f8abf; }
.primary-content { background-color: #caebf6; }
.secondary-content { background-color: #bfe3d0; }
.main-footer { background-color: #b7c0c7; }
.main-header{
padding:15px;
min-height:150px;
}
.main-logo a, .main-nav a{
display:block;
text-decoration:none;
padding:10px 20px;
color:white;
border-radius:5px;
}
.main-nav li{
margin-top:8px;
border-radius:5px;
}
#icon {
background-color: #39add1;
margin-top: 34px;
height: 180px;
border-radius: 5px;
position: relative;
}
#icon:after{
content:"";
background: url('icon.png') no-repeat;
position:absolute;
background-size:100%;
display:inline-block;
width:150px;
height:150px;
top:0;
bottom:0;
left:0;
right:0;
margin: auto;
}
.col{
padding:20px;
}
.main-footer{
padding:15px;
text-align:center;
}
/*media query with relative
==========================================*/
@media(min-width:769px){
.main-header{
position:fixed;
top:0;
width:100%;
z-index:100;
}
body{
padding-top:150px;
font-size:16px;
}
.main-banner{
display:none;
}
.main-logo, .main-nav{
position:absolute;
}
.main-logo{
top:50px;
}
.main-nav li{
display:inline-block;
}
.main-nav{
top:70px;
left:200px;
}
.main-nav li{
margin-right:10px;
}
/*columns
===================*/
.content-row{
/*position:relative;*/
display: table;
}
.col{
/*position:absolute;*/
width:30%;
display: table-cell;
}
.primary-content{
/*left:30%;*/
width:40%;
}
.secondary-content{
/*right:0;*/
}
/*
html, body, .col, .content-row, .main-wrapper{
height:100%;
}
*/
html, body{
height: 100%;
}
.feat-img{
float:left;
width:30%;
}
*{
box-sizing:border-box;
}
.group::after{
content:"";
display:block;
clear:both;
}
}
</style>
</head>
<body>
<div class="main-wrapper">
<header class="main-header">
<h1 class="main-logo"><a href="#">Logo</a></h1>
<ul class="main-nav group">
<li><a href="#">Link 1</a></li>
<li><a href="#">Link 2</a></li>
<li><a href="#">Link 3</a></li>
<li><a href="#">Link 4</a></li>
</ul>
</header>
<div class="main-banner">
<h1>This is the Main Banner Heading</h1>
<p>Andouille pork chop pancetta drumstick ground round beef ribs swine brisket ham.</p>
</div>
<div class="content-row group">
<div class="extra-content col">
<h3>Extra Content</h3>
<p>Filet mignon turkey flank doner strip steak. Frankfurter ham hock turkey, venison sirloin pig chuck shank capicola hamburger doner spare ribs boudin.</p>
<hr>
<p> Drumstick bresaola meatloaf ham hock salami tri-tip landjaeger beef filet mignon biltong boudin turkey.</p>
<div id="icon"></div>
</div>
<div class="primary-content col">
<h2>Primary Content</h2>
<img class="feat-img" src="http://lorempixel.com/400/300" />
<p>Bacon ipsum dolor sit amet chicken pork ground round brisket corned beef ball tip shank tail salami filet mignon ham hock pork belly venison shankle. Pig kielbasa drumstick sausage pork chop boudin. Chicken t-bone salami pork chop, beef ribs kevin ham tri-tip beef venison biltong brisket.</p>
<p>Venison strip steak meatball chicken, brisket prosciutto sirloin. Capicola drumstick brisket tri-tip salami. Chicken beef jerky, tail turkey prosciutto cow ham sirloin boudin tenderloin. Meatloaf tri-tip turducken brisket andouille, pork belly corned beef fatback hamburger.</p>
</div>
<div class="secondary-content col">
<h3>Secondary Content</h3>
<p>Strip steak tenderloin kevin swine meatloaf capicola, doner beef turducken pancetta corned beef pork loin shoulder.</p>
<hr>
<p>Pork filet mignon leberkas, tail swine venison pancetta turkey shoulder brisket chalkers likes hamburgers.</p>
</div>
</div>
<footer class="main-footer">
<p>©2014 Example Layout</p>
</footer>
</div>
</body>
</html>
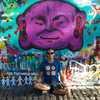
Andrew Stelmach
12,583 PointsRe the image - it's not centered, What kind of effect do you want to achieve?

orange sky
Front End Web Development Techdegree Student 4,945 PointsHello guys!
Since it is a Layout lesson using position/absolute and relative, I will have focus on this topic. Andrew, I find it interesting that I still have to give .content-row a min-height. I thought I had given all my containers a height with this rule below. Can you please tell me why giving .content-row at 100% height is not enough.
html, body, .col, .content-row,.main-wrapper{ height:100%; }
2) As for the image, thanks for telling me it is not centered; I am following the lecture and the rules above are the only rules mentioned to center the picture in the div with the id=icon. Can you please tell me how I can center this icon in my div.
Thanks Geert, for the table display suggestion.
Cheers!

orange sky
Front End Web Development Techdegree Student 4,945 Pointsgot it!