Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial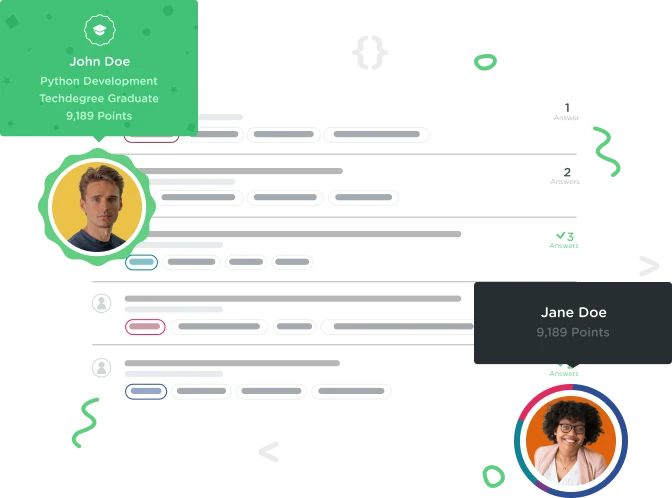

Krasimir Georgiev
19,146 PointsMy dungeon game.Criticism is welcome
Hey guys
Any tips or improvements are welcome.
import random
game_map =[(0,0),(0,1),(0,2),(0,3),(0,4),
(1,0),(1,1),(1,2),(1,3),(1,4),
(2,0),(2,1),(2,2),(2,3),(2,4),
(3,0),(3,1),(3,2),(3,3),(3,4),
(4,0),(4,1),(4,2),(4,3),(4,4)]
monster_pos = game_map[random.randint(0,len(game_map) - 1)]
player_pos = game_map[random.randint(0, len(game_map) - 1)]
door_pos = game_map[random.randint(0, len(game_map) - 1)]
while door_pos == monster_pos:
door_pos = game_map[random.randint(0, len(game_map))]
while player_pos == monster_pos or player_pos == door_pos:
player_pos = game_map[random.randint(0, len(game_map))]
player_shadow = player_pos
player_pos_y, player_pos_x = player_pos
player_shadow_pos_y, player_shadow_pos_x = player_shadow
canGoSouth = False
canGoNorth = False
canGoWest = False
canGoEast = False
gameOver = False
play_again = True
cheatActivated = False
print("You wake up in a dark maze.")
while play_again:
player_shadow = player_pos
player_shadow_pos_y, player_shadow_pos_x = player_shadow
print("Directions you can go in:")
player_shadow_pos_y += 1
if player_shadow_pos_y <= 4 and player_shadow_pos_y >= 0:
print("* North")
canGoNorth = True
else:
canGoNorth = False
player_shadow_pos_y -= 2
if player_shadow_pos_y <= 4 and player_shadow_pos_y >= 0:
print("* South")
canGoSouth = True
else:
canGoSouth = False
player_shadow_pos_x += 1
if player_shadow_pos_x <= 4 and player_shadow_pos_x >= 0:
print("* East")
canGoEast = True
else:
canGoEast = False
player_shadow_pos_x -= 2
if player_shadow_pos_x <= 4 and player_shadow_pos_x >= 0:
print("* West")
canGoWest = True
else:
canGoWest = False
if cheatActivated:
print("Door pos: {}".format(door_pos))
print("Monster pos: {}".format(monster_pos))
print("Player pos: {}".format(player_pos))
direction = input("Where would you like to go?")
if canGoSouth and (direction == 'S' or direction == 'South'):
player_pos_y -= 1
elif canGoNorth and (direction == 'N' or direction == 'North'):
player_pos_y += 1
elif canGoEast and (direction == 'E' or direction == 'East'):
player_pos_x += 1
elif canGoWest and (direction == 'W' or direction == 'West'):
player_pos_x -= 1
elif direction == 'cheat':
cheatActivated = True
print("Cheating activated")
else:
print("Direction not recognized and/or you aren't allowed to go there.")
player_pos = player_pos_y, player_pos_x
if player_pos == monster_pos:
print("Sorry, the monster ate you.")
gameOver = True
elif player_pos == door_pos:
print("Congratulations! You managed to escape the maze!")
gameOver = True
if gameOver:
replay = input("Would you like to play again?")
if not(replay == 'Y' or replay == 'Yes' or replay == 'yes' or replay == 'y'):
play_again = False
1 Answer

Josh Keenan
20,315 PointsIt looks great! Maybe you could add some stuff to it: More monsters Health (Player and monster) Fighting Weapons (Each with different damage capabilities) Inventory Armour (Armor if you like American English..)
Or remake it using classes!
Krasimir Georgiev
19,146 PointsKrasimir Georgiev
19,146 PointsThose are awesome suggestions Josh! I'll update the game soon!