Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial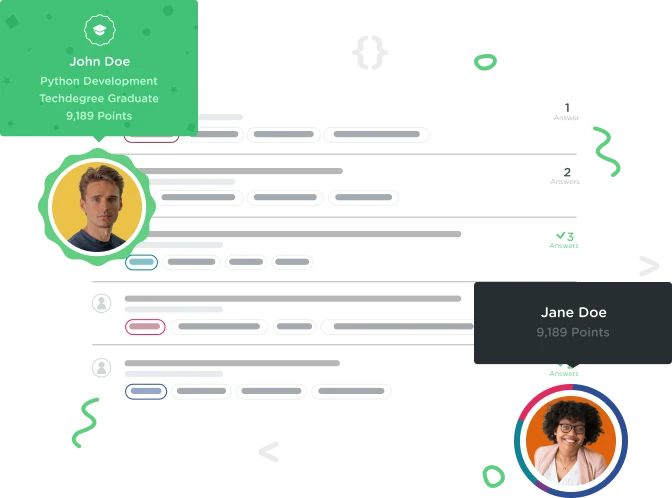

Joel Rodriguez
473 PointsMy emulator isn't loading my app. It would say something like "Unfortunately, (name of app) has stopped working"
Emulator seems to be working good but my app won't run, please help me. Also would love if someone would tell me how I could connect my android phone to android studio. Thanks :).
here is my AndroidManifest.xml code
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="dreamfactory.funfacts2">
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity
android:name=".FunFactsApp"
android:label="@string/app_name"
android:theme="@style/Theme.AppCompat">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
My main activity file
package dreamfactory.funfacts2;
import android.os.Bundle; import android.support.design.widget.FloatingActionButton; import android.support.design.widget.Snackbar; import android.support.v7.app.AppCompatActivity; import android.support.v7.widget.Toolbar; import android.view.View; import android.view.Menu; import android.view.MenuItem; import android.widget.Button; import android.widget.TextView;
public class FunFactsApp extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_fun_facts_app);
Toolbar toolbar = (Toolbar) findViewById(R.id.toolbar);
setSupportActionBar(toolbar);
FloatingActionButton fab = (FloatingActionButton) findViewById(R.id.fab);
fab.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
Snackbar.make(view, "Replace with your own action", Snackbar.LENGTH_LONG)
.setAction("Action", null).show();
// Declare our View variables and assign them the views from the layout file
final TextView factLabel = (TextView) findViewById(R.id.factTextView);
Button showFactButton = (Button) findViewById(R.id.showFactButton);
View.OnClickListener listener = new View.OnClickListener() {
@Override
public void onClick(View v) {
// The button was clicked, so update the fact label with a new fact
String fact = "Ostriches can run faster than horses.";
factLabel.setText(fact);
}
};
showFactButton.setOnClickListener(listener);
}
});
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.menu_fun_facts_app, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
//noinspection SimplifiableIfStatement
if (id == R.id.action_settings) {
return true;
}
return super.onOptionsItemSelected(item);
}
}
1 Answer

Ozhan Saat
Courses Plus Student 4,002 PointsSounds like there is something wrong with the app and not the emulator. Try to do some debugging. It is a very useful thing to practice.
To use your device for testing the first thing you have to do is enable developer options (google it and you'll find good descriptions with screenshots). Then connect your phone to your machine. If you're using a mac you don't need to do anything else. If you have a windows machine then you will need to install drivers. Again, you will need to use google to find how to set up your particular device.
Joel Rodriguez
473 PointsJoel Rodriguez
473 PointsHow do I debug? And thank you, I successfully connected my phone to the computer but it still says "Unfortunately (app name) has stopped working).