Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial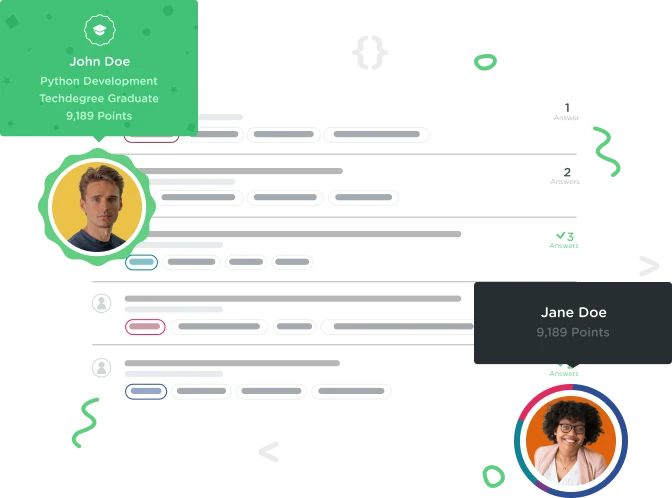
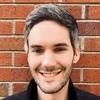
Jesse Vorvick
6,047 PointsMy extra credit solution. Please critique/ask questions!
This one was very difficult for me. But it seems to do everything correctly. This includes stating when a search yields no results and showing multiple students of the same name. Hope this helps and please feel free to critique.
var message = '';
var student;
var search;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getStudentReport( student ) {
var report = '';
for ( var i = 0; i < students.length; i += 1 ) {
if (student.name === students[i].name) {
report += '<h2>Student: ' + students[i].name + '</h2>';
report += '<p>Track: ' + students[i].track + '</p>';
report += '<p>Points: ' + students[i].points + '</p>';
report += '<p>Achievements: ' + students[i].achievements + '</p>';
}
}
return report;
}
while (true) {
search = prompt('Search student records: type a name [Jody] (or type "quit" to end)');
if (search === null || search.toLowerCase() === 'quit') {
break;
}
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if ( student.name.toLowerCase() === search.toLowerCase() ) {
message = getStudentReport( student );
print(message);
break;
} else {
message = '"' + search + '" is not a current student.';
print(message);
}
}
}
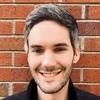
Jesse Vorvick
6,047 PointsHi Lyndon,
Sorry for the late reply. In case you are still wondering, I think I understand your question.
The reason two names are printed if two objects with the same name match the search is because of the for
loop in getStudentReport. This loop iterates through the entire array once because of the code in its parentheses: ( var i = 0; i < students.length; i += 1 )
. If any object in the array has a name property that matches the initial search, they are printed to the page. Not just the first match.
Now, that wasn't the most detailed explanation, but it has been a while since I posted this. So, please let me know if you would like me to elaborate!
4 Answers
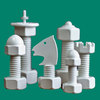
Steven Parker
231,248 PointsIt looks like the "not a current student" message will be printed repeatedly while checking the list. You might want to keep track of a found/not found state and then issue the message only once after the loop.
Otherwise, good job!
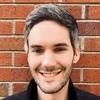
Jesse Vorvick
6,047 PointsI really like that idea. Fortunately it doesn't seem to be the case now, whereas before it would display "is not a current student" no matter what I searched for. The way I got out of that was by adding a break;
statement in the preceding "if" condition.
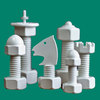
Steven Parker
231,248 PointsThe downside of the "break" is when you have two students with the same name only the first will be shown.
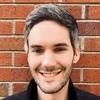
Jesse Vorvick
6,047 PointsRight. That was the other part of the extra credit for this video. My solution was this part of the above code:
function getStudentReport( student ) {
var report = '';
for ( var i = 0; i < students.length; i += 1 ) {
if (student.name === students[i].name) {
report += '<h2>Student: ' + students[i].name + '</h2>';
report += '<p>Track: ' + students[i].track + '</p>';
report += '<p>Points: ' + students[i].points + '</p>';
report += '<p>Achievements: ' + students[i].achievements + '</p>';
}
}
return report;
}
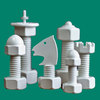
Steven Parker
231,248 PointsJesse Vorvick — Glad to help. You can mark a question solved by choosing a "best answer".
And happy coding!
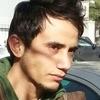
Ahmad Alshaar
10,193 PointsThank You very much for posting , it was very useful.

Colin Theseira
Full Stack JavaScript Techdegree Student 2,025 PointsGreat job with the extra credit on this project.

Dimitris Karatsoulas
5,788 PointsThis might be the best working solution for the extra credit challenge I had found wondering through all the answers. Thank you bery much for you contribution Jesse!
tkiajeqgkn
6,667 Pointstkiajeqgkn
6,667 PointsHey,
Thank you very much for posting your solution. It is a shame that they do not post a video showing their own.
Could you please explain to me how this solution works? I fail to understand why in your function getStudentReport, you are not required to mention anything about adding "student" to the report, and you only add "students[i]". How is that, and how does it work?
Re-iterating, just in case you don't understand - as it appears this was super easy for you so you may not understand my question! Haha - how is it, that you only referenced one variable, and yet two names are printed?
Many thanks.