Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial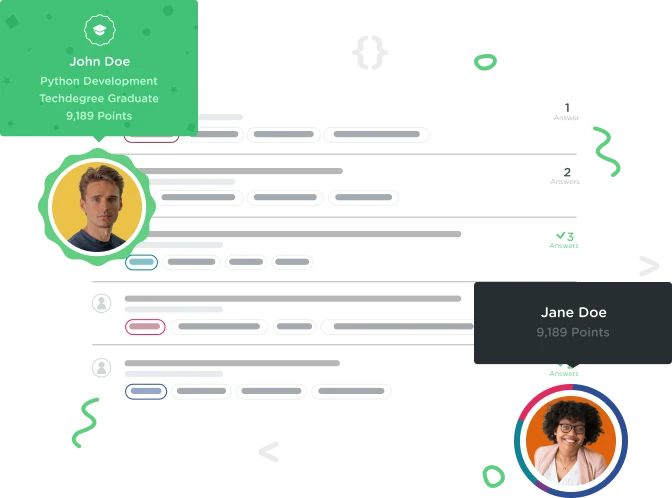
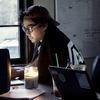
Martel Storm
4,157 PointsMy first app does not work! I just want the button to change the color of the screen when clicked.
This is my first attempt sharing code. Any tips on how to do it correctly? I have the button connected to both Outlet and Action.
import UIKit
class ViewController: UIViewController {
@IBOutlet weak var randomWordButton: UIButton! let colorProvider = BackGroundColorProvider()
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
@IBAction func showWord() {
let randomColor = colorProvider.randomColor()
view.backgroundColor = randomColor
}
}
// Separate File
import GameKit import UIKit
struct BackGroundColorProvider {
let colors = [
UIColor(red: 90/255.0, green: 187/255.0, blue: 181/255.0, alpha: 1.0), // teal color
UIColor(red: 222/255.0, green: 171/255.0, blue: 66/255.0, alpha: 1.0), //yellow color ]
func randomColor() -> UIColor {
let randomNumber = GKRandomSource.sharedRandom().nextInt(upperBound: colors.count)
return colors[randomNumber] } }
2 Answers
Dan Lindsay
39,611 PointsTo check the connection, first go to your Main.storyboard. Once there, make sure your Document Outline is open. If you are not sure what part that is, click the small box(hovering your curser over the box reveals it's name) down beside where it says View as: iPhone7(wC hR), to the left of those words. That will open the Document Outline, and it will show the View Controller Scene with a drop down menu, showing your views, buttons, labels and whatever else you have added to the view controller. When I create outlets or actions, I usually control-drag from this area (using the Assistant Editor) to my code. Sometimes when dragging from the item in the view controller, you can accidentally drag from a constraint, instead of the button or label.
Once you have the Document Outline open, highlight the Button, and open the Connections inspector, which is near the top right hand side of Xcode. It looks like a circle with an arrow pointing to the left inside of it. Now you will see if that button, or whatever you have selected in the Document Outline, is connected and what type of action it is connected too. You could just delete the action here, delete the action in your view controller code, and try again and see if that works.
In terms of how to create the connection, there are a few different ways(which I won't get into here), I just tend to use the Document Outline and Assistant Editor, as I don't seem to run into problems this way. I have with others(i.e. accidentally dragging from a constraint instead of the actual button). Everyone has there own preference though.
I don't know how to do screen-shots, otherwise I would post some of those to make it a bit more clear. If none of this helps, I would have to see the whole project and run it on my end to see what the error could be. You would have to have the project up on github for that though. But, if this is all the code you have so far, and this error keeps happening, maybe just create a new project, with your code like the two files I posted above, and the button in Storyboard. Mine worked just fine. Let me know if you figure it out, and I will do my best to help if I can.
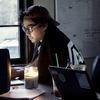
Martel Storm
4,157 PointsThis is wonderful thank you for taking the time to help with this!
Dan Lindsay
39,611 PointsHey Martel,
I was able to get this working in Xcode myself, going off the code you shared. My feeling is that your IBOutlet or IBAction is broken. I noticed that you have your function that changes background color called "showWord", which that function has nothing to do with. Did you change the name of your action AFTER you connected it? That would cause problems. Without seeing the whole project though, I can't tell what is causing your error.
Here is what my two files look like, and I just have one button hooked up in Storyboard:
import UIKit
class ViewController: UIViewController {
let colorChanger = BackgroundColorProvider()
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
@IBAction func changeColor(_ sender: Any) {
let newColor = colorChanger.randomColor()
view.backgroundColor = newColor
}
}
and the second
import UIKit
import GameKit
struct BackgroundColorProvider {
let colors = [
UIColor(red: 90/255.0, green: 187/255.0, blue: 181/255.0, alpha: 1.0), // teal color
UIColor(red: 222/255.0, green: 171/255.0, blue: 66/255.0, alpha: 1.0), //yellow color
]
func randomColor() -> UIColor {
let randomNumber = GKRandomSource.sharedRandom().nextInt(upperBound: colors.count)
return colors[randomNumber]
}
}
In the Main.storyboard file, select the button and checkout the Connections inspector to make sure it is connected right.
Hope this helps
Dan
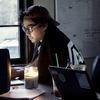
Martel Storm
4,157 PointsHow to properly connect and check a connection? To connect @IBActions (or specifically in this example) I hold control and drag from the button to the view controller which creates one for me. Or can you drag to an existing @IBAaction to change it? Is this how you check connections? View Controller Scene > View Controller > View > Button? Also when you hover over the little black dot next to the line of code next to @IBActions and @IBAOutlets is highlights what it's connected to in the scene. I'm not sure of how much of what I said is correct.
Martel Storm
4,157 PointsMartel Storm
4,157 PointsThis is the error i'm getting libc++abi.dylib: terminating with uncaught exception of type NSException (lldb)