Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial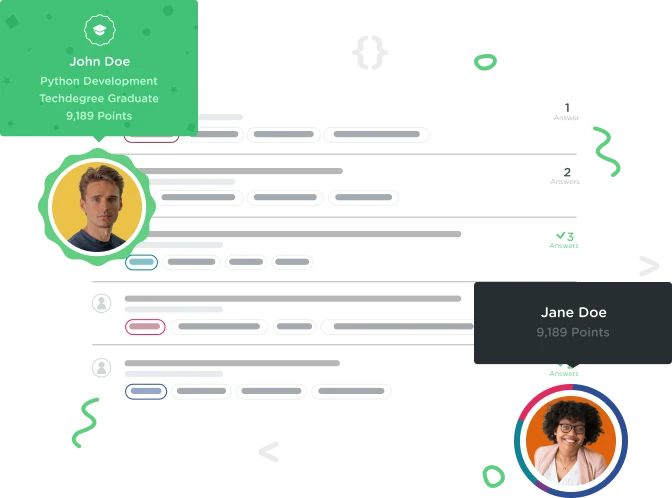

Dominik Huber
4,631 PointsMy first bigger Console Project "Blackjack" - please give me feedback :)
Hi guys,
I recently finished the MVP for the blackjack game in console written in C#. It took me many hours to make this game work in it's very basics.
I tried to use the learned stuff (OOP) as much as I can and I'm pretty satisfied with the outcome.
I see now why OOP is so nice to program because you can visualize the classes in your brain. Anyway the Board class could use some rework because it get's really messy at the end :D
Please give me feedback but also don't forget that I'm a complete beginner. It was very funny to make this and I learned a ton.
Here is the link to the Project: https://github.com/dhuber666/BlackjackConsole
1 Answer

James Churchill
Treehouse TeacherDominik,
Wow! Awesome job taking what you've learned and building an application.
Here are some things to consider:
1) I'd add a .gitignore
file to the root of your repo in order to configure git to ignore files that you don't need to be committing to your repo. For an example of a .gitignore
file to use for Visual Studio, see https://github.com/github/gitignore/blob/master/VisualStudio.gitignore.
2) While code styling is a personal preference sort of thing, I'd remove all of the extraneous extra whitespace (i.e. multiple, concurrent blank lines) from your code.
3) In this method, you have a number of places where you've used "magic numbers" (i.e. literal numeric values).
public int DealerTurn()
{
TheDealer.DisplayHand();
while(TheDealer.ComputeValue() < 17)
{
TheDealer.DealCardToDealer();
}
if(TheDealer.ComputeValue() == 17)
{
return 17;
} else if (TheDealer.ComputeValue() > 21)
{
return 0;
} else if (TheDealer.ComputeValue() > 17 && TheDealer.ComputeValue() <= 21)
{
return TheDealer.ComputeValue();
}
return TheDealer.ComputeValue();
}
While this works, you can make your code easier to read and maintain by replacing these literal values with constants. You can then give your constants names that help convey what these numbers mean in your specific context.
For example, create a constant in the Board class:
private const int MaxHandValue = 21;
You could use the public
access modifier if the constant value is used outside of the Board class. Then update the DealerTurn
method like this:
public int DealerTurn()
{
TheDealer.DisplayHand();
while(TheDealer.ComputeValue() < 17)
{
TheDealer.DealCardToDealer();
}
if(TheDealer.ComputeValue() == 17)
{
return 17;
} else if (TheDealer.ComputeValue() > MaxHandValue)
{
return 0;
} else if (TheDealer.ComputeValue() > 17 && TheDealer.ComputeValue() <= MaxHandValue)
{
return TheDealer.ComputeValue();
}
return TheDealer.ComputeValue();
}
I'll leave it as an exercise for you to replace the "17" literal values with a constant (or more than one constant if "17" can have multiple meanings).
4) What are the case 2
and case 3
switch cases doing in this method?
public void NextAction(int choice, Player player)
{
switch (choice)
{
case 1:
DealCardTurn(player);
break;
case 2:
break;
case 3:
break;
default:
break;
}
}
Adding a code comment to explain this code might be helpful.
5) I like your use of an enum for the card color!
public enum CardColor
{
Club = 0,
Diamond = 1,
Hearth = 2,
Spade = 3
}
Again, great job! I look forward to seeing your next learning project.
Thanks ~James