Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial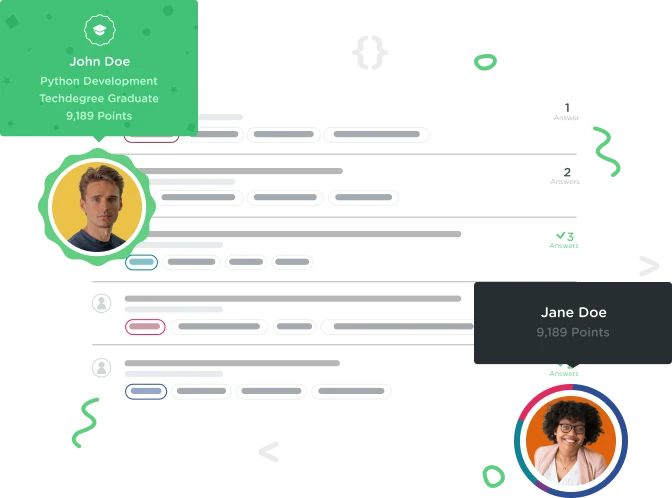

Anthia Tillbury
3,388 PointsMy first game
Learning Swift from scratch I am up to the Class course and decided to see if I could make a simple game with the knowledge I have learned:
• Types • Operators • Arrays • Dictionaries • Control Flow: Loops, If, Boolean • Switch Statements • Functions • Structs • Classes
Practice is different to theory and I have the following sixth draft of the game that I put together, figuring out each problem as I went without asking for help. I am close to the end and now need to finish the game, so I would like a critique on how I did.
OUTLINE:
There are four houses to visit, each one has bones your dog can take. Some houses are farther away than others and require more stamina, which you get from bones (2 bones = 1 stamina). If you visit a house that you have previously been to, the owner will be waiting and take x bones back!
class Abode {
var name: [String]
var bones: Int
var travel: Int
var owner: Int
var visited: [String]
init(name: String, bones: Int, travel: Int, owner: Int) {
self.name = [name]
self.bones = bones
self.travel = travel
self.owner = owner
self.visited = self.name
}
}
class Dog {
var boneCollecion: Int
var stamina: Int
var routeTaken: [String]
init(boneCollection: Int, stamina: Int, routeTaken: [String]) {
self.boneCollecion = boneCollection
// self.boneCollecion = hasVisited(penalty: Int)
self.stamina = Int (stamina / 2)
self.routeTaken = routeTaken
}
}
let albert = Abode(name: "Albert", bones: 2, travel: 0, owner: 1)
let bernárd = Abode(name: "Bernárd", bones: 2, travel: 1, owner: 1)
let cox = Abode(name: "Cox", bones: 7, travel: 9, owner: 5)
let darling = Abode(name: "Darling", bones: 20, travel: 15, owner: 10)
var james = Dog(boneCollection: albert.bones, stamina: albert.bones, routeTaken: albert.name)
//// calculate if house has been visited already
//// if so, a penalty of x bones shall be deducted by house owner
//func hasVisited(penalty: Int) -> Int {
// if james.routeTaken == albert.visited {
// james.boneCollecion - albert.owner
// }
// return penalty
//}
I tried to not repeat myself and keep the code tight, it's not a big complex game but there is a fair bit of referencing going on.
First I initialised the Abode class with four houses ("Albert", Bernard". "Cox" and "Darling" i.e. "A", "B", "C", "D") to give each house it's unique characteristics. The idea being that "Albert" is the house one first visits, here "James" (the dog) gets two bones, of which earns him a Stamina value of one (two bones = 1 stamina), that stamina amassed over time determines if he's able to reach other houses via the travel value.
The other mechanic of the game is that if doggy get's too lazy and visits the same house twice in a row, he will be caught out by the owner waiting for him: I created a helper function outside of the classes to calculate this, but I couldn't link to it when initialising from within the Dog class.
During initialisation of the different houses I passed the name of the Abode into an array that I intended to fill up with the houses visited. As it presently stands the array only contains the present house visited (i.e. the last) but it makes more sense otherwise James couldn't visit any of the houses more than once, which adds a little more fairness and realism that people let their guard down over time.
So if James went from "Albert" to "Bernard" it would be fine, but then back to "Bernard" strait away (because he can get more bones) then the owner would deduct x amount of bones!
The function does not work with the initialiser, I just don't know what's wrong. However, the even bigger issue is how to I collate all the different properties visited?
Many thanks for helping out..
2 Answers
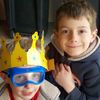
Chris Stromberg
Courses Plus Student 13,389 PointsI tried not to change too much of your code. I made the visited property a bool. I also added a visitAdobeNamed function. Not sure if this is what you were after.
class Abode {
var name: [String]
var bones: Int
var travel: Int
var owner: Int
var visited: Bool = false
init(name: String, bones: Int, travel: Int, owner: Int) {
self.name = [name]
self.bones = bones
self.travel = travel
self.owner = owner
//self.visited = self.name
}
}
class Dog {
var boneCollecion: Int
var stamina: Int
var routeTaken: [String]
init(boneCollection: Int, stamina: Int, routeTaken: [String]) {
self.boneCollecion = boneCollection
// self.boneCollecion = hasVisited(penalty: Int)
self.stamina = Int (stamina / 2)
self.routeTaken = routeTaken
}
func visitAbodeNamed(_ name: Abode){
if name.visited == false {
name.visited = true
self.boneCollecion += name.bones
} else {
if self.boneCollecion <= 0 {
return
} else {
self.boneCollecion -= name.bones
}
}
}
}
let albert = Abode(name: "Albert", bones: 2, travel: 0, owner: 1)
let bernárd = Abode(name: "Bernárd", bones: 2, travel: 1, owner: 1)
let cox = Abode(name: "Cox", bones: 7, travel: 9, owner: 5)
let darling = Abode(name: "Darling", bones: 20, travel: 15, owner: 10)
var james = Dog(boneCollection: albert.bones, stamina: albert.bones, routeTaken: albert.name)
james.visitAbodeNamed(albert)
james.boneCollecion // 4 bones
james.visitAbodeNamed(bernárd)
james.boneCollecion // 6 bones
james.visitAbodeNamed(albert)
james.boneCollecion // 4 bones
//// calculate if house has been visited already
//// if so, a penalty of x bones shall be deducted by house owner
//func hasVisited(penalty: Int) -> Int {
// if james.routeTaken == albert.visited {
// james.boneCollecion - albert.owner
// }
// return penalty
//}

Anthia Tillbury
3,388 PointsMany thanks Chris for your help!
I took a look at this code again and managed to get everything working the way I wanted it:
class Abode {
var name: [String]
var bones: Int
var travel: Int
var owner: Int
init(name: String, bones: Int, travel: Int, owner: Int) {
self.name = [name]
self.bones = bones
self.travel = travel
self.owner = owner
}
}
class Dog {
var boneCollecion: Int = 0
var stamina: Int
var routeTaken: [String]
init(boneCollection: Int, stamina: Int, routeTaken: [String]) {
self.boneCollecion = boneCollection
self.stamina = Int (stamina / 2)
self.routeTaken = routeTaken
}
func whereToGo(_ desired: Abode) {
if self.stamina < desired.travel {
print("you don't have the legs for this journey, pall!")
print("you'll need to build up a stamina of \(desired.travel),")
print("but you only have \(stamina), ha!")
} else if routeTaken == desired.name {
self.boneCollecion -= desired.owner
print("I'm \(desired.name), this is my house. You made a mess of my lawn last time!")
print("Give me \(desired.owner) bones!")
print("Don't come back in a hurry troublemaker!")
} else {
self.stamina -= desired.travel
self.routeTaken = desired.name
self.boneCollecion += desired.bones
self.stamina += desired.bones / 2
print("ahh, I remember now I left \(desired.bones) bones here!")
print("now I have \(boneCollecion) ? to my name")
}
}
//trigger point: 40 bones
func bonusStamina(_ trade: Bool) -> (Int, Int) {
print("trade bones for stamina?")
if trade == true {
self.stamina += (boneCollecion / 2)
self.boneCollecion -= 40
print("you got it busta, stamina: \(stamina)")
} else {
print("See you in another 40!")
}
return (stamina, boneCollecion)
}
}
let zero = Abode(name: "Start", bones: 0, travel: 0, owner: 0)
let albert = Abode(name: "Albert", bones: 2, travel: 0, owner: 1)
let bernárd = Abode(name: "Bernárd", bones: 2, travel: 1, owner: 1)
let cox = Abode(name: "Cox", bones: 7, travel: 9, owner: 5)
let darling = Abode(name: "Darling", bones: 20, travel: 25, owner: 10)
However, you can see there is a bonus function in there that will exchange bones for stamina once the threshold of 40 is reached and I wanted to know how to automatically call that please?