Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial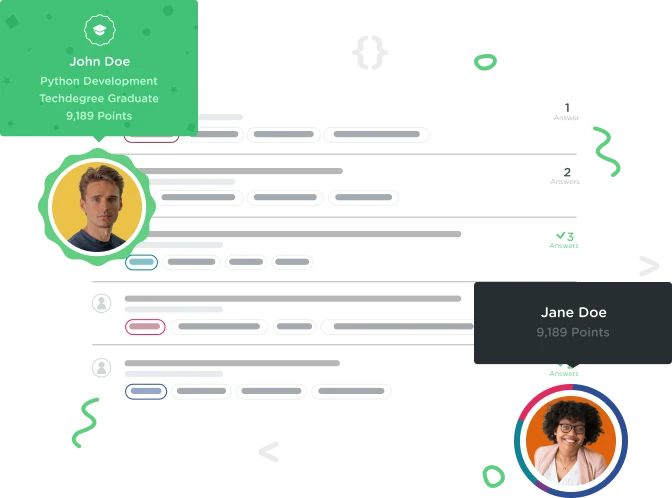

juleshanson
332 PointsMy frog only moves forward, help!
using UnityEngine;
using System.Collections;
public class PlayerMovement : MonoBehaviour {
private Animator playerAnimator;
private float moveHorizontal;
private float moveVertical;
private Vector3 movement;
// Use this for initialization
void Start () {
playerAnimator = GetComponent<Animator> ();
}
// Update is called once per frame
void Update () {
moveHorizontal = Input.GetAxisRaw ("Horizontal");
moveVertical = Input.GetAxisRaw ("Vertical");
movement = new Vector3 (moveHorizontal, 0.0f, moveVertical);
}
void FixedUpdate () {
if (movement != Vector3.zero) {
playerAnimator.SetFloat("Speed", 3f);
} else {
playerAnimator.SetFloat("Speed", 0f);
}
}
}
Thats my code, please help.
3 Answers
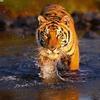
Konrad Pilch
2,435 PointsI recommend to downolad Nick code and paste line by line and see if it works. I would help but i dont know about the code so what you could do its just copy and paste Nick code and see if it works, and then if it does, go line by line comparing it, ud know something is wrong then.

Tom Bedford
15,645 PointsHi Jules, I think you are doing ok so far - the left/right rotations are covered a few videos later on in the course.
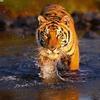
Konrad Pilch
2,435 PointsUnless you didnt do the rotation of other directions with Nick :D Usualy everything you do in porgramming, you need to say it step by step.
So every single thing that you want to happen, you need to say , so if you did forward, you need to do jump, left, right, backwards and all.
Hope this helps too.

John Shoffner
1,514 PointsHi juleshanson,
You are missing the "Quaternion" code; quaternion is a mathematical algorithm used to rotate 3D objects. The process of rotating 3D objects is really easy using Unity's Quaternion class (i.e. really easy, once you understand how to use this class! ;-) ). NOTE: You do not want to write your own code to rotate a 3D object!
Under "Player Input and Cameras", start at the "Quaternions" training video.
<Hint> You're FixedUpdate() method is where the Quaternion code will be added.
HTH