Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial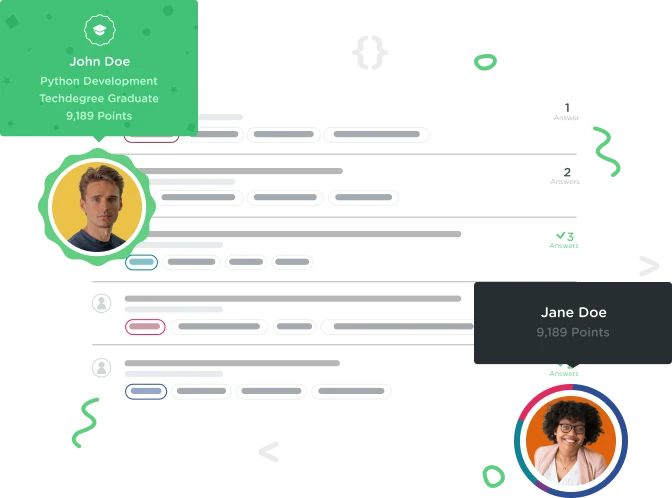
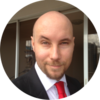
Gabor Galantai
Courses Plus Student 17,247 PointsMy frog turns alright but seems to change its speed of movement
My frog turns very nicely, but sometimes it barely moves in the direction it's supposed to move, seeming almost as if it would be jumping in place, other times it goes off the screen in 2 jumps... I can't see a reason why it does that.
Here is my code:
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class PlayerMovement : MonoBehaviour {
private Animator playerAnimator;
private float moveHorizontal;
private float moveVertical;
private Vector3 movement;
private float turningSpeed = 20f;
private Rigidbody playerRigidbody;
// Use this for initialization
void Start () {
// Gather components from the Player GameObject
playerAnimator = GetComponent<Animator>();
playerRigidbody = GetComponent<Rigidbody>();
}
// Update is called once per frame
void Update () {
moveHorizontal = Input.GetAxisRaw("Horizontal");
moveVertical = Input.GetAxisRaw("Vertical");
movement = new Vector3(moveHorizontal, 0.0f, moveVertical);
}
void FixedUpdate () {
// If player's movement vector doesn't equal zero...
if (movement != Vector3.zero) {
// ...then create a target rotation based on the movement vector
Quaternion targetRotation = Quaternion.LookRotation(movement, Vector3.up);
// ...and create another rotation that moves from the current rotation to the target rotation
Quaternion newRotation = Quaternion.Lerp(playerRigidbody.rotation, targetRotation, turningSpeed * Time.deltaTime);
// ...and change the player's rotation to the new incremental rotation
playerRigidbody.MoveRotation(newRotation);
// ...then accelerate the player to play the jump animation
playerAnimator.SetFloat("Speed", 3f);
} else {
playerAnimator.SetFloat("Speed", 0f);
}
}
}
1 Answer
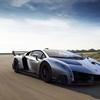
Chelsea C.
Full Stack JavaScript Techdegree Student 3,392 PointsHm. Weird. Here's my code which works fine. See if it works for you
using System.Collections; using System.Collections.Generic; using UnityEngine;
public class PlayerMovement : MonoBehaviour {
private Animator playerAnimator;
//When the player presses keys on the keyboard, this is where the information will go.
private float moveHorizontal;
private float moveVertical;
private Vector3 movement;
private float turningSpeed = 20f;
private Rigidbody playerRigidbody;
// Use this for initialization
void Start () {
// Gather components fromt the Player GameObject
playerAnimator = GetComponent<Animator> ();
playerRigidbody = GetComponent<Rigidbody> ();
}
// Update is called once per frame
void Update () {
//sets a decimal number called moveHorizontal that's going to tell us whether the player is trying to move left or right.
moveHorizontal = Input.GetAxisRaw ("Horizontal");
moveVertical = Input.GetAxisRaw ("Vertical");
movement = new Vector3 (moveHorizontal, 0.0f, moveVertical);
}
//This method is good for objects that rely on physics
//It doesn't run as often
void FixedUpdate () {
//If it's anything other than all zeros then the player is pressing keys
if (movement != Vector3.zero) {
//player animation
//set the player speed
//This rotation should happen before the player moves forward
// ...then create a target rotation based on the movement vector
Quaternion targetRotation = Quaternion.LookRotation(movement, Vector3.up);
//...and create another roation that moves from the current roation to the target rotation
Quaternion newRotation = Quaternion.Lerp (playerRigidbody.rotation, targetRotation, turningSpeed * Time.deltaTime);
// ...and change the players rotation to the new incremental rotation.
playerRigidbody.MoveRotation(newRotation);
playerAnimator.SetFloat ("Speed", 3f);
} else {
playerAnimator.SetFloat ("Speed", 0f);
}
}
}
Gabor Galantai
Courses Plus Student 17,247 PointsGabor Galantai
Courses Plus Student 17,247 PointsIt still doesn't work properly. When I navigate the frog, sometimes it goes at seemingly normal pace, other times it's as if it would be jumping almost in one place.