Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial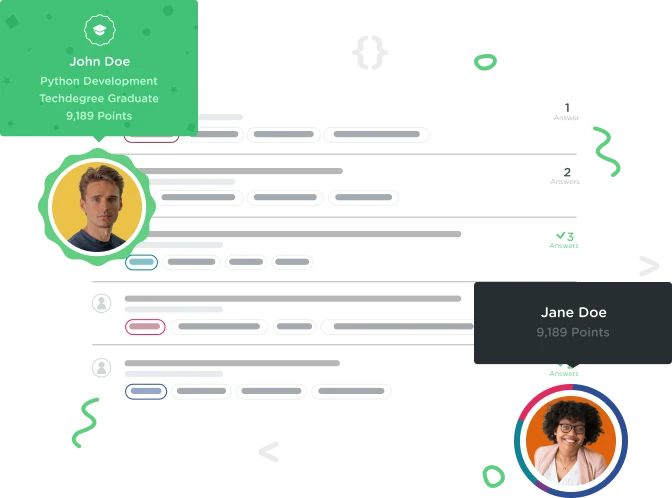
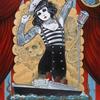
Saira Bottemuller
Courses Plus Student 1,749 PointsMy funnybunny random number generator:
var randomNumber = Math.floor(Math.random() * 100) +1;
document.write("Your totally random number is: " + " " + randomNumber + "!" + "<br />" + "<br />");
var firstUserNumber = prompt("Now, please enter an integer");
var firstLimit = parseInt(firstUserNumber);
var secondRandomNumber = Math.floor(Math.random() * firstLimit) + 1;
document.write("You entered " + firstUserNumber + ", now here's a random number between one and " + firstUserNumber + ": " + "<br />" + "<br />" + secondRandomNumber + "<br />" + "<br />");
var secondUserNumber = prompt("Do you dare enter a second, non-equal integer?!");
var secondLimit = parseInt(secondUserNumber);
var thirdRandomNumber = Math.floor(Math.random() * secondLimit) + 1;
document.write("Now you've gone and entered " + secondUserNumber + ", so I suppose I can go ahead and pick a random number between " + firstUserNumber + " and " + secondUserNumber + "... Let me see... Howabout " + "<br />" + "<br />" + thirdRandomNumber + "! Take THAT!");
3 Answers
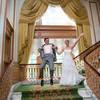
Colin Bell
29,679 PointsHey Saira,
Just wanted to give you a small tip. Your thirdRandomNumber
is actually returning a random number between 1 and the secondLimit
instead of a random number between firstLimit
and secondLimit
.
To do that, you'd have find the range between the two numbers by subtracting the smaller number from the bigger one. Then adding the smaller number to that:
var firstLimit = parseInt(firstUserNumber);
var secondLimit = parseInt(secondUserNumber);
function getRandom(x, y) {
if (x-y !== 0) {
return Math.floor(Math.random() * (y - x + 1)) + x;
}
else if (x - y === 0){return "You picked the same number when I specifically asked you not to?!"}
}
var thirdRandomNumber = getRandom(firstLimit, secondLimit);
Hope that helps a little.
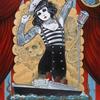
Saira Bottemuller
Courses Plus Student 1,749 PointsYes, it definitely does, thank you! Haha I love the "same number" statement :D Thank you for the tip, that could present problems down the line if I'm not doing it correctly. This also illustrates a need for me to do more testing, instead of relying on just one test! Thanks Colin! :D
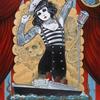
Saira Bottemuller
Courses Plus Student 1,749 PointsI do have a question though, at which point should this code be inserted, or would it be best to restructure the program altogether, but integrating the new intel you've given me? I'm sure a seasoned JSer could put it in line by line, but I'm pretty new to JS - what's the best policy here for a novice, to go ahead and rewrite the program snippet with the new information/better code, or to try and figure out where to stick the new code in/what to pull out, and risk breaking the program and getting wrapped up like a kitten in masking tape? lol :D I'm trying to build good habits for the future. What would you do?
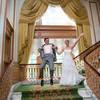
Colin Bell
29,679 PointsI typically like declaring all variables at the top, so I know exactly what is being used. But with the way this flows (prompt then print, prompt then print, ...), if you try doing that then it will capture all of the variables first and print everything to the screen after it's finished with all the prompts.
I'd probably set it up like this. Also note, I made a change to the getRandom() function - The way I had it originally only worked properly if a smaller number was put in first:
var getRandom = function (x, y) {
if (x < y ) {
return Math.floor(Math.random() * (y - x + 1)) + x;
}
else if (x > y) {
return Math.floor(Math.random() * (x - y + 1)) + y;
}
else if (x - y === 0){
return "You picked the same number when I specifically asked you not to?!";
}
}
// First random number gets automatically generated and printed
var randomNumber = Math.floor(Math.random() * 100) +1;
document.write("Your totally random number is: " + " " + randomNumber + "!" + "<br />" + "<br />");
// firstUserNumber captured and secondRandomNumber gets printed
// Notice you can parseInt() on the prompt right away.
// You can always keep it as a separate variable if you want though
var firstUserNumber = parseInt(prompt("Now, please enter an integer"));
var secondRandomNumber = Math.floor(Math.random() * firstUserNumber) + 1;
document.write("You entered " + firstUserNumber + ", now here's a random number between one and " + firstUserNumber + ": " + "<br />" + "<br />" + secondRandomNumber + "<br />" + "<br />");
// secondUserNumber captured and thirdRandomNumber printed.
var secondUserNumber = parseInt(prompt("Do you dare enter a second, non-equal integer?!"));
var thirdRandomNumber = getRandom(firstUserNumber, secondUserNumber);
document.write("Now you've gone and entered " + secondUserNumber + ", so I suppose I can go ahead and pick a random number between " + firstUserNumber + " and " + secondUserNumber + "... Let me see... Howabout " + "<br />" + "<br />" + thirdRandomNumber + "! Take THAT!");