Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial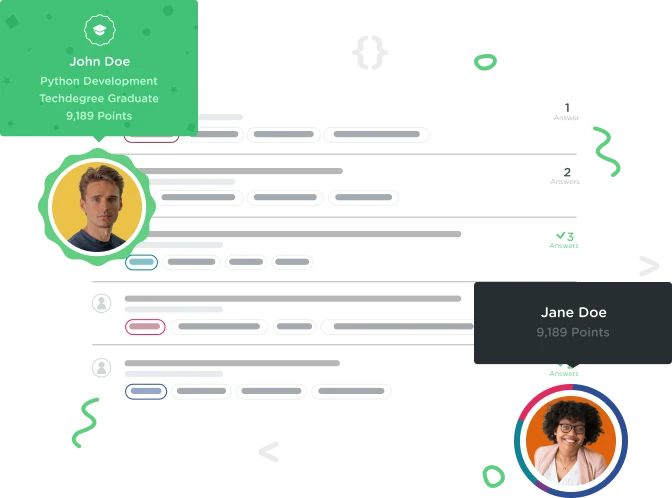
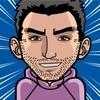
victorvillaplana
11,582 PointsMy game says I won even before starting!
Hi, After adding the code to determine if the player wins or loses, when I run the code in the console it says 'Congratulations!' even before starting to play. Could you help me find where my mistake is? Thanks!!
class Game {
public static final int MAX_MISSES = 7;
private String answer;
private String hits;
private String misses;
public Game(String answer) {
this.answer = answer.toLowerCase();
hits = "";
misses = "";
}
private char normalizeGuess(char letter) {
if(!Character.isLetter(letter)) {
throw new IllegalArgumentException("A letter is required");
}
letter = Character.toLowerCase(letter);
if ((hits.indexOf(letter) != -1) || misses.indexOf(letter) != -1) {
throw new IllegalArgumentException(letter + " has already been guessed");
}
return letter;
}
public boolean applyGuess(String letters) {
if (letters.length() == 0) {
throw new IllegalArgumentException("No letter found");
}
return applyGuess(letters.charAt(0));
}
public boolean applyGuess(char letter) {
letter = normalizeGuess(letter);
boolean isHit = answer.indexOf(letter) != -1;
if (isHit) {
hits += letter;
} else {
misses += letter;
}
return isHit;
}
public int getRemainingTries() {
return MAX_MISSES - misses.length();
}
public String getCurrentProgress(){
String progress = "";
for (char letter : answer.toCharArray()) {
char display = '_';
if (hits.indexOf(letter) == -1) {
display = letter;
}
progress += display;
}
return progress;
}
public boolean isWon() {
return getCurrentProgress().indexOf('_') == -1;
}
public String getAnswer() {
return answer;
}
}
import java.util.Scanner;
class Prompter {
private Game game;
public Prompter(Game game) {
this.game = game;
}
public boolean promptForGuess() {
Scanner scanner = new Scanner(System.in);
boolean isHit = false;
boolean isAcceptable = false;
do {
System.out.print("Enter a letter: ");
String guessInput = scanner.nextLine();
try {
isHit = game.applyGuess(guessInput);
isAcceptable = true;
} catch (IllegalArgumentException iae) {
System.out.printf("%s. Please try again. %n",
iae.getMessage());
}
} while (!isAcceptable);
return isHit;
}
public void displayProgress() {
System.out.printf("You have %d remaining tries: %s%n",
game.getRemainingTries(),
game.getCurrentProgress());
}
public void displayOutcome() {
if (game.isWon()) {
System.out.printf("Congratulations! You won with %d tries remaining.%n",
game.getRemainingTries());
} else {
System.out.printf("Sorry... The answer was %s.%n",
game.getAnswer());
}
}
}
public class Hangman {
public static void main(String[] args) {
// Your incredible code goes here...
Game game = new Game("treehouse");
Prompter prompter = new Prompter(game);
while (game.getRemainingTries() > 0 && !game.isWon()) {
prompter.displayProgress();
prompter.promptForGuess();
}
prompter.displayOutcome();
}
}
2 Answers

Sean M
7,344 PointsHi Victor. I have the exact same code as you, and it is running fine in Intellij. Are you using it in Java IDE?
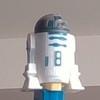
Teacher Russell
16,873 PointsActually, I think the error is up in the getCurrentProgress method, not in isWon(). He should be checking if the letter is in hits with != -1, right?

Dearce Goodman
2,331 PointsI found that public boolean isWon(){ return getCurrentProgress().indexOf('-') == -1; will fix the problem. your .indexOf('_') was looking for the wrong char.
'-' compared to '_'
Teacher Russell
16,873 PointsTeacher Russell
16,873 PointsCheck out your isWon method again. What are you looking for in indexOf?:)