Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial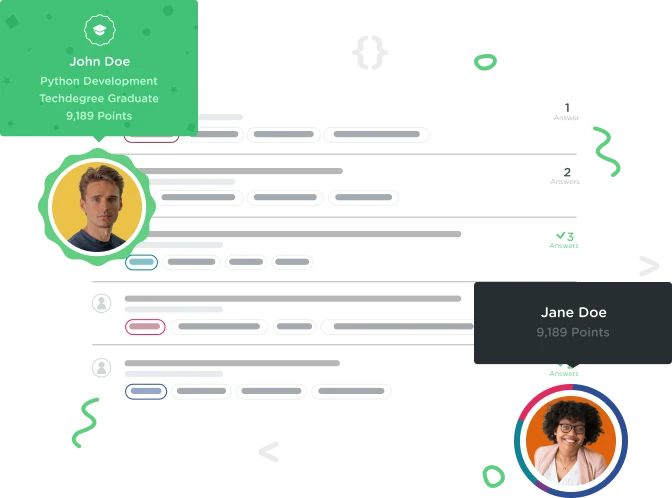
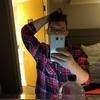
Gemma Weirs
15,054 PointsMy if/else statement isn't working
I'm trying to understand the basics of if/else statements and I wrote one for learning purposes.
var magicNumber = 350 / 2;
if (magicNumber < 65) {
console.log("There isn't a magic number.");
} else (magicNumber > 65) {
console.log("The magic number is " + magicNumber);
}
But the console keeps saying there's an unexpected {. I can't see where I've gone wrong.
3 Answers
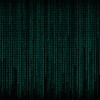
Andrew Dushane
9,264 PointsYou don't need a condition for your else statement. It captures anything 'else' that's not true in your if statement. So take out (magicNumber > 65)
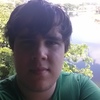
David Omar
5,676 Pointsyou have to use else if. else is used as a default with no comparisons
var magicNumber = 350 / 2;
if (magicNumber < 65) {
console.log("There isn't a magic number.");
} else if(magicNumber > 65) {
console.log("The magic number is " + magicNumber);
else {
//whatever...
}
}
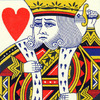
Iskandar Fendi
5,700 PointsFor else in if/else statement, you don't need to add boolean value inside of it (Like mNumber < 65). I think that's the reason why you program fail.
It's just
else
{
console.log("more than 65");
}
You can just simply said
if( number < 65 ) {
console.log(" less than 65");
}
console.log("More than 65");
The program will check the number variable let's say 125, the compiler check the first condition (<65), it will fail the first condition and it will skip it and go to the next line and read the "more than 65 line". You can add else around "more than 65" but it's unnecessary because it's either less than or more than. (At least, that's for Java not Javascript, but please try it);
But if you have condition, that want to have different response for certain value, then if, else if, else will be take in place:
var mNumber = 200
if ( mNumber == 200 )
{
console.log("It's 200!");
}
else if (mNumber < 200)
{
console.log("It's less than 200");
}
else
{
console.log("It's more than 200");
}
Remember, if it's involved if/else if/ then you should put else at the end, but if it's only if-statement, than you don't need to add else
Hope this helps. Let me know if you have any further questions
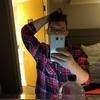
Gemma Weirs
15,054 PointsThanks, that makes things a little clearer. :)
I tried out both adding else and leaving it out.
For example, I changed magicNumber to evaluate to a number lower than 65, and then omitted the else statement. The result was, "There isn't a magic number" immediately followed by, "The magic number is 35."
So I added the else statement back in, and then I got the desired results, which was, "There isn't a magic number".
Gemma Weirs
15,054 PointsGemma Weirs
15,054 PointsThat worked. :) Thanks. I hadn't thought of it this way.