Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial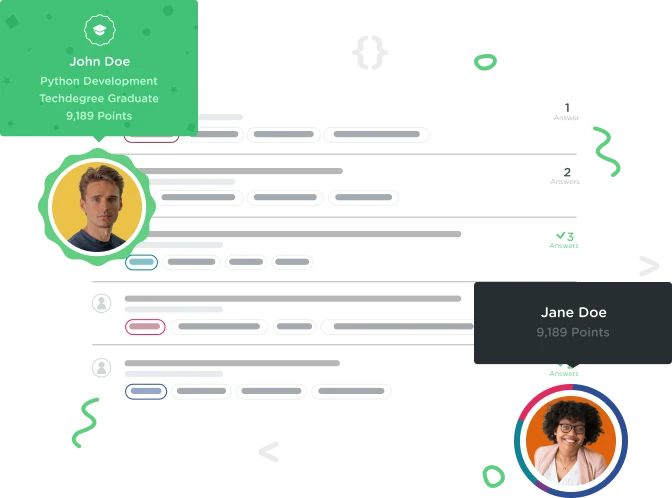
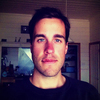
Sam Donald
36,305 PointsMy improvements for the JavaScript Loops, Arrays and Objects quiz challenge.
I added an if loop to change the use of 'question' or 'questions' depending on the number of correct answers. I also added the code to display the correct response next to the correct answer results in green. And the incorrect responses next to the wrong answer results in red, with a wrong message beneath each wrong answer.
here's my workspace snapshot. To test it just Fork it and then preview. https://w.trhou.se/6p7pqmdthl
It's from this challenge: https://teamtreehouse.com/library/javascript-loops-arrays-and-objects/tracking-multiple-items-with-arrays/build-a-quiz-challenge-part-1
It looks like Snapshot's not working so here's my code. You can compile it in your editor to test or just read in here. I like to test.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>JavaScript Quiz</title>
<link rel="stylesheet" href="css/styles.css">
</head>
<body>
<h1>JavaScript Quiz</h1>
<div id="output">
</div>
<script src="js/quiz.js"></script>
</body>
</html>
@import url('http://necolas.github.io/normalize.css/3.0.2/normalize.css');
/*General*/
body {
background: #fff;
max-width: 980px;
margin: 0 auto;
padding: 0 20px;
font: Helvetica Neue, Helvectica, Arial, serif;
font-weight: 300;
font-size: 1em;
line-height: 1.5em;
color: #8d9aa5;
}
a {
color: #3f8aBf;
text-decoration: none;
}
a:hover {
color: #3079ab;
}
a:visited {
color: #5a6772;
}
h1, h2, h3 {
font-weight: 500;
color: #384047;
}
h1 {
font-size: 1.8em;
margin: 60px 0 40px;
}
h2 {
font-size: 1em;
font-weight: 300;
margin: 0;
padding: 30px 0 10px 0;
}
#home h2 {
margin: -40px 0 0;
}
h3 {
font-size: .9em;
font-weight: 300;
margin: 0;
padding: 0;
}
h3 em {
font-style: normal;
font-weight: 300;
margin: 0 0 10px 5px;
padding: 0;
color: #8d9aa5;
}
ol {
margin: 0 0 20px 32px;
padding: 0;
}
#home ol {
list-style-type: none;
margin: 0 0 40px 0;
}
li {
padding: 8px 0;
display: list-item;
width: 100%;
margin: 0;
counter-increment: step-counter;
}
#home li::before {
content: counter(step-counter);
font-size: .65em;
color: #fff;
font-weight: 300;
padding: 2px 6px;
margin: 0 18px 0 0;
border-radius: 3px;
background:#8d9aa5;
line-height: 1em;
}
.lens {
display: inline-block;
width: 0;
height: 0;
border-top: 8px solid transparent;
border-bottom: 8px solid transparent;
border-right: 8px solid #8d9aa5;
border-radius: 5px;
position: absolute;
margin: 5px 0 0 -19px;
}
#color div {
width: 50px;
height: 50px;
display: inline-block;
border-radius: 50%;
margin: 5px;
}
span {
color: red;
font-size: 1.2em;
}
span.correctResponse {
color: green;
}
span.returnedMessage {
display: block;
font-size: 0.93em;
}
var questions = [
// ["question", correct_aswer, "message_if_wrong"]
["How many characters in this question?", 37, "37, spaces count as characters in a string"],
["How many children can Chinese famalies have?", 2, "Chinese famalies can now have 2 children"],
["How many eyes did the three blind mice have?", 6, "6, just because they're blind doesn't mean they have no eyes."]
];
var correctAnswers = 0;
var queston;
var answer;
var messageIfWrong;
var response;
var html;
var correct = [];
var wrong = [];
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function buildList(arr){
var listHTML = '<ol>';
for (var i = 0; i < arr.length; i++){
listHTML += '<li>' + arr[i] + '</li>';
}
listHTML += '</ol>';
return listHTML;
}
for (var i = 0; i < questions.length; i++) {
question = questions[i][0];
answer = questions[i][1];
messageIfWrong = questions[i][2];
response = parseInt(prompt(question));
if (response === answer){
correctAnswers += 1;
correct.push(question + " " + '<span class="correctResponse">' + answer + '</span>');
} else {
wrong.push(question + " " + '<span>' + response + '</span>' + '<span class="returnedMessage">' + messageIfWrong + '</span>');
}
}
// Change grammar of number right depending on number right
if (correctAnswers === 1) {
html = "You got " + correctAnswers + " question right.";
} else {
html = "You got " + correctAnswers + " questions right.";
}
//Change grammar of correct answers h2 depending on number right
if(correctAnswers < 1){
html += "<h2>You got no questions correct</h2>";
} else if (correctAnswers > 1) {
html += "<h2>You got these questions correct</h2>";
} else {
html += "<h2>You got this question correct</h2>";
}
html += buildList(correct);
//Change grammar of wrong answers h2 depending on number right
if(correctAnswers === questions.length) {
html += "<h2>You got no questions wrong</h2>";
} else if (correctAnswers > 1 && correctAnswers < questions.length){
html += "<h2>You got this question wrong</h2>";
} else {
html += "<h2>You got these questions wrong</h2>";
}
html += buildList(wrong);
print(html);