Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial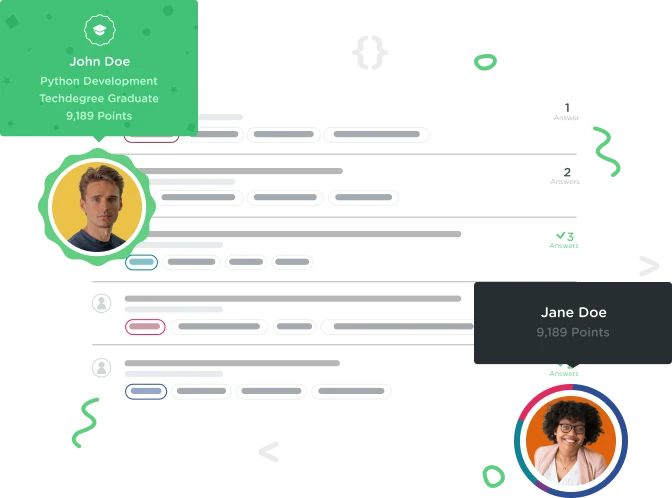
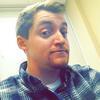
Jake Ford
9,230 PointsMy Index view is not letting me use the strongly typed Movie[] array
I'm getting the following error in the browser upon running:
The model item passed into the dictionary is of type 'MovieGallery.Models.Movie[]', but this dictionary requires a model item of type 'MovieGallery.Models.Movie'.
I don't know why it doesn't like the Movie[] model type..
My controller:
using MovieGallery.Models;
using MovieGallery.Data;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
namespace MovieGallery.Controllers
{ //Controller classes need to be public for MVC to use them!
public class MovieGalleryController : Controller
{ //Action methods need to be public for MVC to find and use them!
//underscore is a common convention for private fields
private MovieRepository _movieRepository = null;
public MovieGalleryController()
{
_movieRepository = new MovieRepository();
}
public ActionResult Index()
{
var movies = _movieRepository.GetMovies();
return View(movies);
}
public ActionResult Detail(int? id)
{
if(id == null)
{
return HttpNotFound();
}
var movie = _movieRepository.GetMovie((int)id);
return View(movie);
}
}
}
My data repository
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using MovieGallery.Models;
namespace MovieGallery.Data
{
public class MovieRepository
{
private static Movie[] _movies = new Movie[]
{
new Movie()
{
Title = "Rogue One, A Star Wars Trilogy",
Id = 1,
Rated = "PG-13",
Length = "2h 13m",
PlotHtml = "<p><b>Plot</b>: In a time of conflict, a group of unlikely heroes band together on a mission to steal the plans to the Death Star, the Empire’s ultimate weapon of destruction. This key event in the Star Wars timeline brings together ordinary people who choose to do extraordinary things, and in doing so, become part of something greater than themselves.</p>",
Actors = new string[]
{
"Felicity Jones",
"Diego Luna",
"Alan Tudyk",
"Donnie Yen",
"Wen Jiang"
}
},
new Movie()
{
Title = "Beerfest",
Id = 2,
Rated = "PG-13",
Length = "2h 10m",
PlotHtml = "<p><b>Plot</b>: In a time of conflict, a group of unlikely heroes band together on a mission to steal the plans to the Death Star, the Empire’s ultimate weapon of destruction. This key event in the Star Wars timeline brings together ordinary people who choose to do extraordinary things, and in doing so, become part of something greater than themselves.</p>",
Actors = new string[]
{
"Felicity Jones",
"Diego Luna",
"Alan Tudyk",
"Donnie Yen",
"Wen Jiang"
}
},
new Movie()
{
Title = "Jumanji",
Id = 3,
Rated = "PG-13",
Length = "2h 10m",
PlotHtml = "<p><b>Plot</b>: In a time of conflict, a group of unlikely heroes band together on a mission to steal the plans to the Death Star, the Empire’s ultimate weapon of destruction. This key event in the Star Wars timeline brings together ordinary people who choose to do extraordinary things, and in doing so, become part of something greater than themselves.</p>",
Actors = new string[]
{
"Felicity Jones",
"Diego Luna",
"Alan Tudyk",
"Donnie Yen",
"Wen Jiang"
}
}
};
public Movie[] GetMovies()
{
return _movies;
}
public Movie GetMovie(int id)
{
Movie movieToReturn = null;
foreach (var movie in _movies)
{
if(movie.Id == id)
{
movieToReturn = movie;
break;
}
}
return movieToReturn;
}
}
}
My Index view
@model MovieGallery.Models.Movie[]
@{
ViewBag.Title = "Movies";
}
<h2>@ViewBag.Title</h2>
<div class="row">
@foreach (var movie in Model)
{
<div class="col-md-3">
<h4>@movie.DisplayText</h4>
<img src="/Images/@movie.CoverImageFileName"
alt="@movie.DisplayText"
class="img-responsive" />
</div>
}
</div>
My Movie Model
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
namespace MovieGallery.Models
{
public class Movie
{
public int Id { get; set; }
public string Title { get; set; }
public string Rated { get; set; }
public string Length { get; set; }
public string PlotHtml { get; set; }
public string[] Actors { get; set; }
public string DisplayText
{
get
{
return Id + " - " + Title;
}
}
public string CoverImageFileName
{
get
{
return Title.Replace(" ", "-").ToLower() + "-" + Id + ".jpg";
}
}
}
}
1 Answer

James Churchill
Treehouse TeacherJacob,
I'm not immediately seeing the error in your code. Could you share the entire project maybe via a GitHub repo?
Thanks ~James