Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial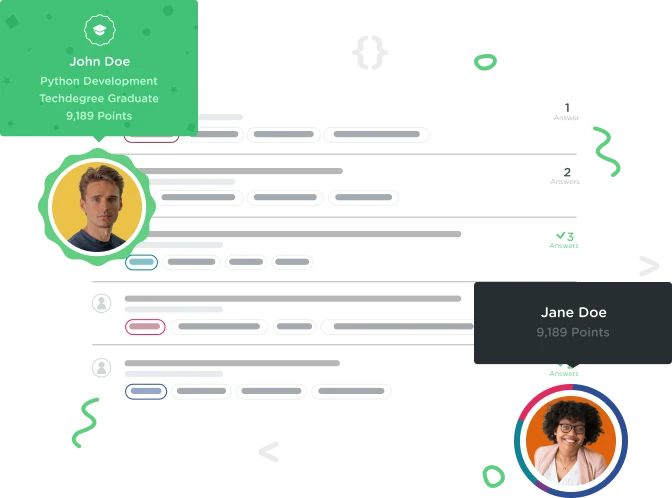

Yuda Leh
7,618 PointsMy inverse of Number Game
So Kenneth asked us to make an inverse of the Number Game, so that is what I did. I made a very very simple one. I am not sure if this was the correct way of doing it so I decided to post it here and ask the community. I want to thanks, everyone in advance for any help. Thanks!!!
My code:
### Computer Does Number Game ###
import random
def your_secret_number():
return int(input("Enter number for computer to guess: "))
def computers_random_number(low, high):
return random.randint(low, high)
def reply_game():
answer = str(input("Do you want to play again? Y/n: "))
if answer == "Y".lower():
game()
def game():
try:
secret = your_secret_number()
low = int(input("Enter min: "))
high = int(input("Enter max: "))
limit = int(input("Limit to give computer guesses: "))
except ValueError:
print("That is not a number")
else:
while(limit > 0):
computers = computers_random_number(low, high)
if computers == secret:
print("Computer guessed {}, your number was {}! Computer guessed your number!!".format(computers, secret))
reply_game()
break
else:
print("Computer guessed {}, the right number was {}!! The computer failed".format(computers, secret))
limit -= 1
reply_game()
game()
4 Answers
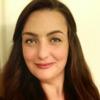
Jennifer Nordell
Treehouse TeacherSorry for the delay in getting back to you. I agree with Alexander Davison. I think you did a great job!
As he points out, there are some improvements that could be done such as making it so the computer doesn't guess the same number twice. And I feel like I can guess what he means by "making the computer smarter". Now, you could have it where the computer just guesses randomly within that range. Or, you could give the computer hints, such as "That number was too high". So that the computer will now guess a random number between the minimum and the last number it just guessed. The end result will be seeing if the computer can guess the number in a certain amount of tries given clues.
That being said, these algorithms might be a bit more advanced than where you are right now. Given your progress through the track thus far, even completing the extra credit assignment and making it work is a huge milestone. If you continue along this path, you'll be able to implement those changes in this game in no time! Keep coding and good luck!

Yuda Leh
7,618 PointsWow, thanks so much. From what I understand to making the computer smarter.
Depending on its last guess, for its the number is 8 and the computer guesses 2 I would let the computer know it's too low and update the range from min: 2 to the max the user enter?
Once again thanks.
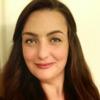
Jennifer Nordell
Treehouse TeacherYes, that's exactly what I mean except that if the computer guessed 2 and it was too low, you'd update the range from 3 to the max instead of 2 to max. Because we've already eliminated 2 as a possibility. But you've got the idea! Keep up the great work!

Yuda Leh
7,618 Points### Computer Does Number Game ###
import random
computer_guesses = []
def your_secret_number():
return int(input("Enter number for computer to guess: "))
def computers_random_number(low, high):
return random.randint(low, high)
def reply_game():
answer = str(input("Do you want to play again? Y/n: "))
if answer == "Y".lower():
computer_guesses[:] = []
game()
else:
exit()
def game():
has_guessed = False
c = 0
try:
secret = your_secret_number()
low = int(input("Enter min: "))
high = int(input("Enter max: "))
limit = int(input("Limit to give computer guesses: "))
except ValueError:
print("That is not a number")
else:
while(limit > 0):
computers = computers_random_number(low, high)
computer_guesses.append(computers)
######################################################################################
if computers == secret:
print("Computer guessed {}, your number was {}! Computer guessed your number!!".format(computers, secret))
reply_game()
break
else:
print("Computer guessed {}, the right number was {}!! The computer failed".format(computers, secret))
# print(computer_guesses)
limit -= 1
if computers == computer_guesses[c]:
# print("{} already guessed".format(computers))
comp = computers
if computers > secret:
print("message to comp -> you [computer] are to high")
high = int(computers)
print(high)
print(computers)
else:
print("message to comp -> you [computer] are to low")
low = int(computers)
print(low)
print(computers)
limit += 1
continue
c += 1
reply_game()
######################################################################################
game()
Hey there Jennifer Nordell, here in the "#####...." I tried to make it so the computer didn't count a limit and essentially skipped the number guess if it had already done so and that number was incorrect. I ran my program a few time and even manually set then computers var to 5 and did a couple test. The program seems to work, but something doesn't feel right, I am not sure if it really works (seems really messy). Do you have any advice? I have also completed the updating of the range depending on of the computer was too high or too low. -thanks
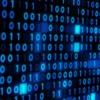
Alexander Davison
65,469 PointsYou did a good job! Maybe make it so the computer can't guess the same number twice?
Also, maybe you can make the computer a little bit smarter. :)

Yuda Leh
7,618 PointsThanks for the reply, Going to try and make the computer smarter from what I understand now

Freda Wan
6,391 PointsTook up your challenge of making sure the computer can't guess the same number twice.
Any other suggestions welcome. Jennifer Nordell
'''
REVERSE NUMBER GAME
Additional challenge: ensuring the computer does not guess same number twice.
'''
import random
exclude_list = []
def game():
user_num = int(input("Choose a number between 0 and 9. "))
guess_range = range(10)
computer_num = random.randint(1, 10)
while True:
if computer_num == user_num:
print("Computer wins! Correctly guessed {}".format(computer_num))
break
elif computer_num < user_num:
exclude_list.append(computer_num)
print("Computer guessed {}, a lower number than yours.".format(computer_num))
l1 = [i for i in guess_range if i > computer_num]
l2 = [i for i in l1 if i not in exclude_list]
computer_num = random.choice(l2)
else:
exclude_list.append(computer_num)
print("Computer guessed {}, a higher number than yours.".format(computer_num))
l3 = [i for i in guess_range if i < computer_num]
l4 = [i for i in l3 if i not in exclude_list]
computer_num = random.choice(l4)
game()
Joseph Marcus
11,069 PointsHey Yuda Leh and Jennifer Nordell ...
Cool stuff...
See my attempt below...
"""This program is the inverse number game that allows the computer
guess a number chosen by a human """
import random
def main():
try:
user_choice_number = int(get_user_chosen_number())
if user_choice_number not in range(1, 10):
print('Please enter a number between 1 and 10')
retry_game()
else:
guesses_allowed = int(get_number_of_times_to_guess())
allow_computer_guess(user_choice_number, guesses_allowed)
retry_game()
except ValueError:
print('Your input is not a valid number')
def get_user_chosen_number():
return input('Enter a number between 1 and 10: ')
def get_number_of_times_to_guess():
return input('How many times can I guess? ')
def retry_game():
retry_game = input('Do you want to play again? Y/n: ')
if retry_game.lower() == 'y':
main()
else:
print('Thank you for playing. Byebye.')
def get_computer_guess(min, max):
return random.randint(min, max)
def allow_computer_guess(user_choice_number, guesses_allowed):
min = 1
max = 10
already_guessed = []
while guesses_allowed > 0:
input('\nPress enter so I can guess...')
computer_guess = get_computer_guess(min, max)
guesses_allowed -= 1
if computer_guess in already_guessed:
computer_guess = get_computer_guess(min, max)
else:
already_guessed.append(computer_guess)
if computer_guess == user_choice_number:
print('Yippee! I got it! You chose {}!\n'.format(computer_guess))
break
elif computer_guess < user_choice_number:
min = computer_guess
print('Hmmn...My guess was less...trying again with a higher number...I guessed {}'.format(computer_guess))
else:
max = computer_guess
print('Phew! My guess was too high...trying again. I guessed {}'.format(computer_guess))
else:
print("O well! I guess I'd guess it right next time")
main()
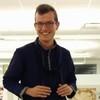
slavster
1,551 PointsNice Job! My one thought while looking through your code was that you only perform the "computer_guess in already_guessed" check once. Given the range is only 1-10, there is a 10% chance that on the next line where the computer generates a random number, that it will just generate the same number again, My solution to this was to put a loop within a loop to continuously check the uniqueness of the randomly generated number and only allow the game to go forward if it is truly unique. Here's my small edit:
while guesses_allowed > 0:
input('\nPress enter so I can guess...')
computer_guess = get_computer_guess(min, max)
guesses_allowed -= 1
while True:
if computer_guess in already_guessed:
computer_guess = get_computer_guess(min, max)
else:
already_guessed.append(computer_guess)
break

Ee Kok Yoong
318 PointsMy attempt................................................................................................................... top part of the codes are not differentiated with dark background.
import random
num = 50 low = 1 high = 100
while True: guess = random.randint(low, high) if guess > num: print(guess) high = guess
elif guess < num:
print(guess)
low = guess
else:
print("This is the answer", guess)
break
Ashish Shah
1,468 PointsAshish Shah
1,468 Pointsdef computers_random_number(low, high): - Why did you take low and high?