Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial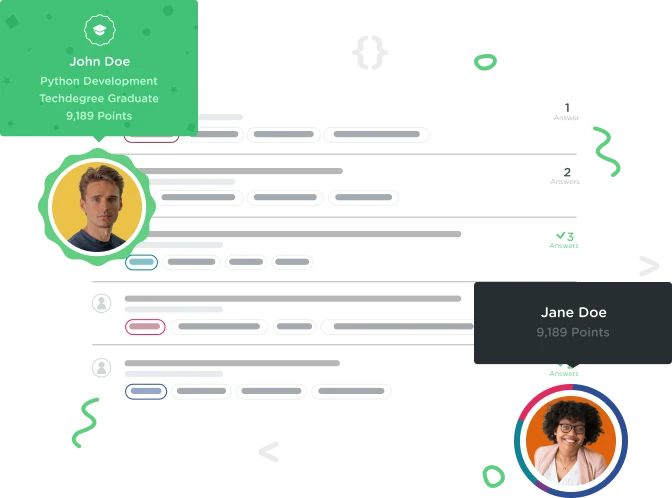

Marco Fregoso
90 PointsMy JavaScript code so that Format and Genre display according to the category selected (Every Step Explained)
This is what I came up after watching the course and applying some JavaScript, I know I could've made it better but I'm also just learning, would really appreciate some feedback.
//setting the default class for every option group inside #format and #genre so that they are hidden by default
$("#format optgroup, #genre optgroup").toggleClass("not-visible");
var $lastToggle; // created variable to keep track of the last selector whose class was toggled
$("#category").on("click", function() { //if #category is clicked do the following:
//if an option nested inside #category has been selected
if ($("#category option:selected").val() != "") {
var selected = $(this).val().toLowerCase(); //get the value of the selected option
switch (selected) {
case "books":
console.log("inside books selected case");
/*select option groups within #format and #genre with a label value of "Books"and store or
"cache" the selector into a variable so that the selector doesn't have to be sought-after every
time we need to use it*/
var $formatGenreBooks = $("#format optgroup[label='Books'], #genre optgroup[label='Books']");
/*testing to see whether or not the "Book" option group has a class named "not-visible"
in case someone clicks the same category twice so that the class doesn't toggle back and forth
if it doesn't need to do it, if that's true do the following*/
if ($formatGenreBooks.hasClass("not-visible")){
/*Toggle "not-visible" class so that it removes the class and the option group for the
given case becomes visible*/
$formatGenreBooks.toggleClass("not-visible");
/*First we test to see if the lastToggle wasn't the same case as the current one
then we test to see if there is a $lastToggle, that is checking if it is not undefined,
in other wordschecking if it has a value if both are true proceed*/
if ($lastToggle != $formatGenreBooks && typeof $lastToggle != "undefined") {
console.log("Last toggle restored happened");
/*Setting Format and Genre value back to its original value, this code runs only when
there was a $lastToggle, we then go to its direct parent, that is the select element
we then set its value to "" so that it will select the direct child with a "" value (the
default's value of "select one"), we will then trigger the change event on the
select element so that it will update as if it was manually changed by a user*/
$lastToggle.parent().val("").trigger("change");
/*If there is a last toggle this code runs and we toggle the class so that it is removed
from the last case and it switches back to its initial value*/
$lastToggle.toggleClass("not-visible");
}
//after the last check we then set this to be the $lastToggle to keep track of the last case
$lastToggle = $formatGenreBooks;
console.log("books was invisible and is now visible");
}
break;
case "movies":
/*The exact same thing is happening here as in the code above, read the last code documentation*/
console.log("inside movies selected case");
var $formatGenreMovies = $("#format optgroup[label='Movies'], #genre optgroup[label='Movies']");
if ($formatGenreMovies.hasClass("not-visible")) {
$formatGenreMovies.toggleClass("not-visible");
if ($lastToggle != $formatGenreMovies && typeof $lastToggle != "undefined") {
console.log("Last toggle restored happened");
$lastToggle.parent().val("").trigger("change");
$lastToggle.toggleClass("not-visible");
}
$lastToggle = $formatGenreMovies;
console.log("Movies was invisible and is now visible");
}
break;
case "music":
console.log("inside music selected case");
var $formatGenreMusic = $("#format optgroup[label='Music'], #genre optgroup[label='Music']");
if ($formatGenreMusic.hasClass("not-visible")) {
$formatGenreMusic.toggleClass("not-visible");
if ($lastToggle != $formatGenreMusic && typeof $lastToggle != "undefined") {
console.log("Last toggle restored happened");
$lastToggle.parent().val("").trigger("change");
$lastToggle.toggleClass("not-visible");
}
$lastToggle = $formatGenreMusic;
console.log("Music was invisible and is now visible");
}
break;
}
}
});