Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial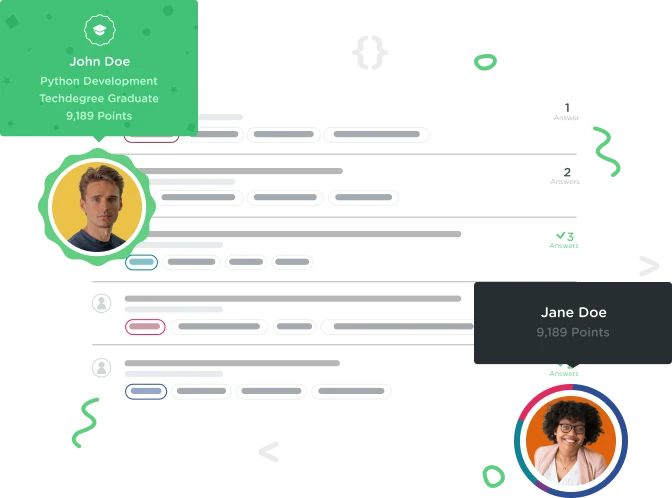

venkatesh rane
356 PointsMy labels and Values are not Changing
updateDisplay();
4 Answers

Vikzilla III
Courses Plus Student 4,365 PointsI was having a problem where my currentWeather object's properties were all null or 0. And realized it was because I was still returning an empty object at the end of the getCurrentDetails() method:
return new CurrentWeather();
As opposed to returning the instance of CurrentWeather that we set the values for:
return currentWeather;

Nasir Mahamoud
22,892 PointsThe problem may be in your getCurrentDetails method. Check logcat for caught json exceptions. Then make sure all of your json keys are spelled correctly. If even one key is misspelled, your code will break at the exception, and your display will not update.
Here is a working copy of the getCurrentDetails method:
private CurrentWeather getCurrentDetails(String jsonData) throws JSONException {
JSONObject forecast = new JSONObject(jsonData);
String timezone = forecast.getString("timezone");
Log.i(TAG,"From JSON: " + timezone);
JSONObject currently = forecast.getJSONObject("currently");
CurrentWeather currentWeather = new CurrentWeather();
currentWeather.setHumidity(currently.getDouble("humidity"));
currentWeather.setTime(currently.getLong("time"));
currentWeather.setIcon(currently.getString("icon"));
currentWeather.setPrecipChance(currently.getDouble("precipProbability"));
currentWeather.setSummary(currently.getString("summary"));
currentWeather.setTemperature(currently.getDouble("temperature"));
currentWeather.setTimeZone(timezone);
Log.d(TAG, currentWeather.getFormattedTime());
return currentWeather;
}
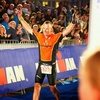
Steve Hunter
57,712 PointsCan you show us the code where you are calling updateDisplay()
as well as the code inside updateDisplay()
please?
Steve.

venkatesh rane
356 Points public void onResponse(Response response) throws IOException {
try {
String jsonData = response.body().string();
Log.v(TAG, jsonData );
if (response.isSuccessful()) {
mCurrentWeather = getCurrentDetails(jsonData);
runOnUiThread(new Runnable() {
@Override
public void run() {
updateDisplay();
}
});
} else {
alertUserAboutError();
}
}
catch (IOException e) {
Log.e(TAG, "Exception caught:", e);
}
catch (JSONException e){
Log.e(TAG, "Exception caught:", e);
}
}
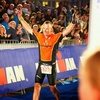
Steve Hunter
57,712 PointsOK - that all looks fine, I think. Can you show me the updateDisplay()
function too, please.
Also, when this code is executed, do you get some errors logged into Logcat? The updateDisplay()
method is called from within a try/catch
block - are the catch
bits running and avoiding the try
?
I'd recommend putting some breakpoints around your code to see where execution reaches - or put some Toast commands around to do the same. A Toast right before you call updateDisplay()
will let you know if the code is reaching there, or not.
Steve.
Nancy Melucci
Courses Plus Student 36,143 PointsNancy Melucci
Courses Plus Student 36,143 PointsTHANK YOU for that information!!!